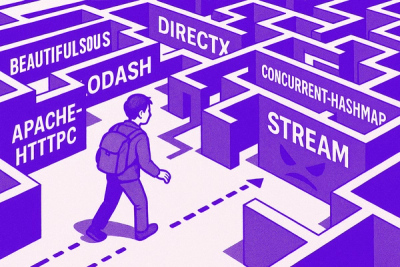
Research
NPM targeted by malware campaign mimicking familiar library names
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
@fordi-org/bloks-parser
Advanced tools
This is a small parser library for parsing the `blok_payload` field of Instagram / Threads API responses. This parser has no API details about IG/Threads APIs; it is _only_ for being able to pick apart the payloads in code.
This is a small parser library for parsing the blok_payload
field of Instagram / Threads API responses. This parser has no API details about IG/Threads APIs; it is only for being able to pick apart the payloads in code.
import createBlokParser from '@fordi-org/bloks-parser';
const bloks_payload = `
(bk.action.map.Make,
(bk.action.array.Make, "login_type", "login_source"),
(bk.action.array.Make, "Password", "Login")
)
`;
const parseBloks = createBlokParser();
const bloks = parseBloks(bloks_payload);
and the result will be:
["bk.action.map.Make",
["bk.action.map.Make", "login_type", "login_source"],
["bk.action.map.Make", "Password", "Login"]
]
The parser is also designed so you can create your own handlers for each blok type when generating the parser, e.g.,
import createBlokParser from '@fordi-org/bloks-parser';
const parse = createBlokParser({
"bk.action.array.Make": (_, entries) => entries,
"bk.action.i32.Const": (_, [value]) => parseInt(value),
"bk.action.bool.Const": (_, [value]) => !!value,
"bk.action.map.Make": (_, [keys, values]) => {
const result = {};
for (let i = 0; i < keys.length; i++) {
result[keys[i]] = values[i];
}
return result;
}
});
const payload = '(bk.action.array.Make,(bk.action.i32.Const,42069),"nice",(bk.action.bool.Const,true),(bk.action.map.Make,(bk.action.array.Make,"a","b","c"),(bk.action.array.Make,(bk.action.i32.Const,1),(bk.action.i32.Const,2),(bk.action.i32.Const,3))))';
console.log(JSON.stringify(parse(payload)));
[42069,"nice",true,{"a":1,"b":2,"c":3}]
The above are exported as BASIC_PROCESSORS:
import createBlokParser, { BASIC_PROCESSORS } from '@fordi-org/bloks-parser';
const parseWithBasics = createBlokParser(BASIC_PROCESSORS);
The special @
processor will allow you to have more custom behavior than just (name, args) => [name, ...args]
, which is the default. This is useful in that you could have something like,
/*...*/
"@": (name, args, isLocal) => {
console.warn(`Unknown blok: ${isLocal ? '#' : ''}${name} (\n ${
JSON.stringify(args, null, 2).split('\n').join('\n ')
}\n)`);
return [name, ...args];
},
/*...*/
So, like, for debugging, that'll report when it comes across a block type you haven't handled yet.
To get the repo working:
npm ci
npm build:peg
If you make changes to src/bloks.pegjs
, you must run npm build:peg
again.
Of note, there's few unit tests as yet. If you submit an issue, I'll fix it and write a test against the error case.
FAQs
This is a small parser library for parsing the `blok_payload` field of Instagram / Threads API responses. This parser has no API details about IG/Threads APIs; it is _only_ for being able to pick apart the payloads in code.
The npm package @fordi-org/bloks-parser receives a total of 3 weekly downloads. As such, @fordi-org/bloks-parser popularity was classified as not popular.
We found that @fordi-org/bloks-parser demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
Research
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.