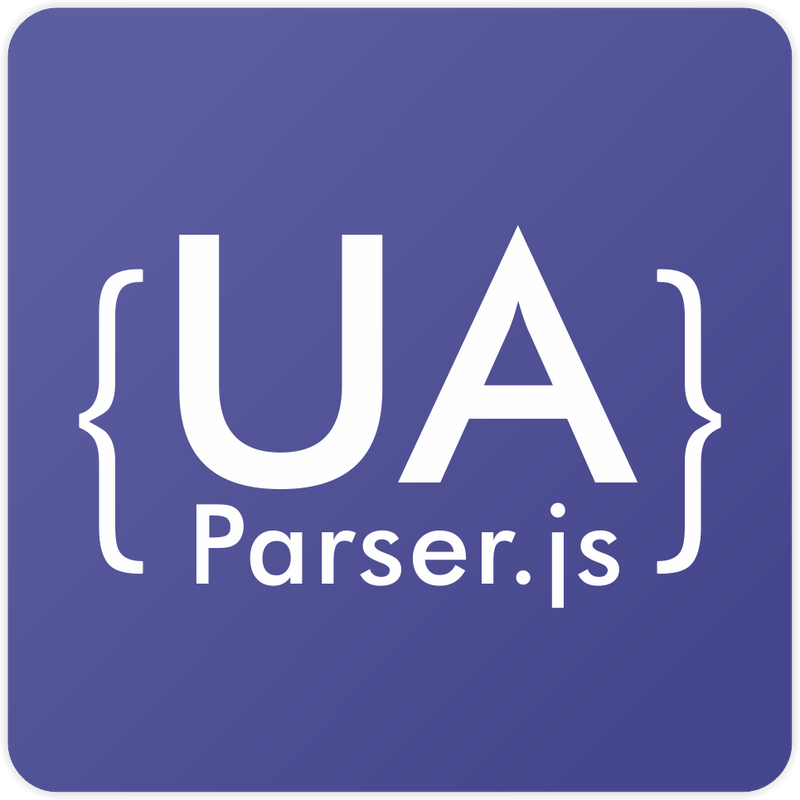
Security News
ua-parser-js Drops MIT License, Adopts Controversial AGPLv3 + PRO Dual Licensing Model in Upcoming v2.0
ua-parser-js is set to drop the MIT license and adopt a controversial dual AGPLv3 + PRO licensing model in its upcoming v2.0 release, raising significant concerns among developers and enterprise users.