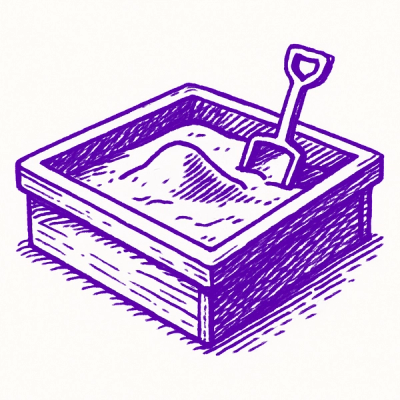
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
@graphql-codegen/typescript-graphql-request
Advanced tools
GraphQL Code Generator plugin for generating a ready-to-use SDK based on graphql-request and GraphQL operations
@graphql-codegen/typescript-graphql-request is a plugin for GraphQL Code Generator that generates TypeScript typings and a GraphQL client using `graphql-request`. It helps in creating strongly-typed GraphQL queries and mutations, making it easier to work with GraphQL APIs in a TypeScript environment.
Generate TypeScript Types
This configuration generates TypeScript types for your GraphQL schema and operations. It uses the `typescript`, `typescript-operations`, and `typescript-graphql-request` plugins to create a strongly-typed client.
```json
{
"schema": "https://my-graphql-api.com/schema",
"documents": "src/**/*.graphql",
"generates": {
"src/generated/graphql.ts": {
"plugins": [
"typescript",
"typescript-operations",
"typescript-graphql-request"
]
}
}
}
```
GraphQL Client Generation
This code demonstrates how to use the generated SDK to make a GraphQL request. The `getSdk` function creates a client instance that can be used to execute queries and mutations with full TypeScript support.
```typescript
import { GraphQLClient } from 'graphql-request';
import { getSdk } from './generated/graphql';
const client = new GraphQLClient('https://my-graphql-api.com/graphql');
const sdk = getSdk(client);
async function fetchData() {
const data = await sdk.MyQuery();
console.log(data);
}
fetchData();
```
Apollo Client is a comprehensive state management library for JavaScript that enables you to manage both local and remote data with GraphQL. Compared to `@graphql-codegen/typescript-graphql-request`, Apollo Client offers more features like caching, local state management, and advanced error handling.
urql is a highly customizable and versatile GraphQL client for React. It provides a set of hooks and utilities to manage GraphQL queries and mutations. While `@graphql-codegen/typescript-graphql-request` focuses on generating TypeScript types and a simple client, urql offers more flexibility and integration with React.
Relay is a JavaScript framework for building data-driven React applications with GraphQL. It emphasizes performance and scalability, offering advanced features like data normalization and efficient data fetching. Unlike `@graphql-codegen/typescript-graphql-request`, Relay is more opinionated and requires a specific setup.
FAQs
GraphQL Code Generator plugin for generating a ready-to-use SDK based on graphql-request and GraphQL operations
The npm package @graphql-codegen/typescript-graphql-request receives a total of 397,930 weekly downloads. As such, @graphql-codegen/typescript-graphql-request popularity was classified as popular.
We found that @graphql-codegen/typescript-graphql-request demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.