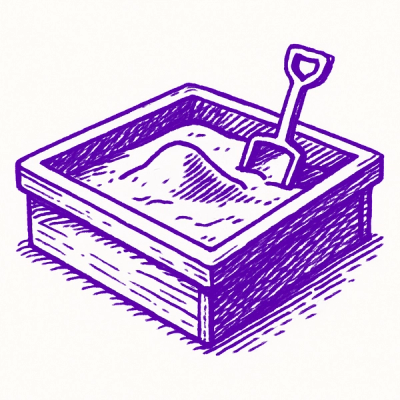
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
@graphql-tools/git-loader
Advanced tools
A set of utils for faster development of GraphQL tools
The @graphql-tools/git-loader package is designed to load GraphQL schema files and documents directly from a Git repository. This is particularly useful for managing and integrating GraphQL schemas and documents that are version-controlled in Git, allowing developers to fetch and utilize these resources dynamically in their GraphQL server implementations.
Load GraphQL schemas from Git
This feature allows developers to load GraphQL schemas directly from a Git repository. The code sample demonstrates how to load a schema from a specific branch and path within a Git-hosted repository using the GitLoader.
import { loadSchema } from '@graphql-tools/git-loader';
async function getSchema() {
const schema = await loadSchema('git://github.com/example/repo#branch:path/to/schema.graphql', {
loaders: [
new GitLoader()
]
});
return schema;
}
Load GraphQL documents from Git
This feature enables the loading of GraphQL documents (queries, mutations, subscriptions) from a Git repository. The provided code sample shows how to fetch documents using a glob pattern from a specified branch and path in a repository.
import { loadDocuments } from '@graphql-tools/git-loader';
async function getDocuments() {
const documents = await loadDocuments('git://github.com/example/repo#branch:path/to/documents/*.graphql', {
loaders: [
new GitLoader()
]
});
return documents;
}
Similar to @graphql-tools/git-loader, graphql-config is used to handle GraphQL project configuration. It supports loading configurations from multiple sources but does not directly integrate Git loading capabilities. This makes @graphql-tools/git-loader more specialized for scenarios involving Git repositories.
The graphql-import package allows importing and bundling GraphQL files. While it facilitates modularizing and reusing GraphQL schema definitions, it lacks the direct Git integration provided by @graphql-tools/git-loader, focusing instead on local file imports.
FAQs
A set of utils for faster development of GraphQL tools
The npm package @graphql-tools/git-loader receives a total of 3,348,726 weekly downloads. As such, @graphql-tools/git-loader popularity was classified as popular.
We found that @graphql-tools/git-loader demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.