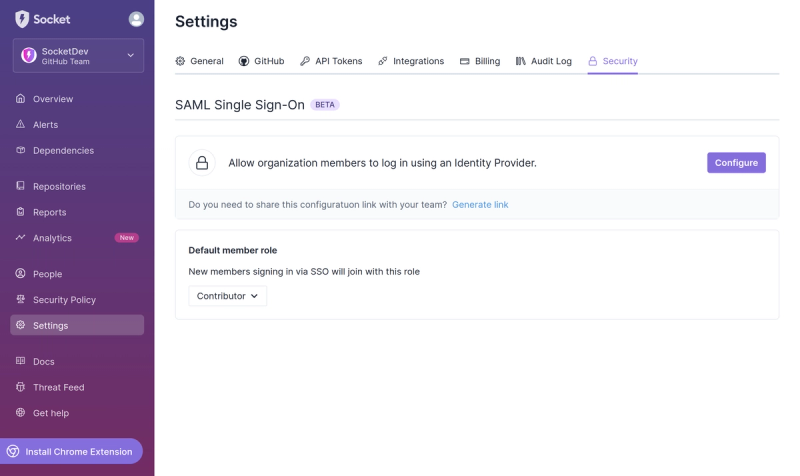
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
@hackolade/mongodb-client-encryption
Advanced tools
Readme
The Node.js wrapper for libmongocrypt
You can install mongodb-client-encryption
with the following:
npm install mongodb-client-encryption
Run the following command to build libmongocrypt and setup the node bindings for development:
bash ./etc/build-static.sh
Some tests require a standalone server to be running with authentication enabled. Set up a single server running with the following conditions:
param | value |
---|---|
host | localhost |
port | 27017 |
This is the standard setup for a standalone server with no authentication.
Run the test suite using:
npm test
There are breaking changes planned for this package. In the next major version, callbacks will be removed from the public API on all asynchronous functions. Additionally, the classes documented here will be moved into node-mongodb-native.
An internal class to be used by the driver for auto encryption NOTE: Not meant to be instantiated directly, this is for internal use only.
An error indicating that something went wrong specifically with MongoDB Client Encryption
An error indicating that ClientEncryption.createEncryptedCollection()
failed to create data keys
An error indicating that ClientEncryption.createEncryptedCollection()
failed to create a collection
An error indicating that mongodb-client-encryption failed to auto-refresh Azure KMS credentials.
*
any serializable BSON value
BSON.Long
A 64 bit integer, represented by the js-bson Long type.
object
Configuration options that are used by specific KMS providers during key generation, encryption, and decryption.
object
A data key as stored in the database.
string
A string containing the name of a kms provider. Valid options are 'aws', 'azure', 'gcp', 'kmip', or 'local'
object
The ClientSession class from the MongoDB Node driver (see https://mongodb.github.io/node-mongodb-native/4.8/classes/ClientSession.html)
object
The result of a delete operation from the MongoDB Node driver (see https://mongodb.github.io/node-mongodb-native/4.8/interfaces/DeleteResult.html)
object
The BulkWriteResult class from the MongoDB Node driver (https://mongodb.github.io/node-mongodb-native/4.8/classes/BulkWriteResult.html)
object
The FindCursor class from the MongoDB Node driver (see https://mongodb.github.io/node-mongodb-native/4.8/classes/FindCursor.html)
Binary
The id of an existing dataKey. Is a bson Binary value. Can be used for ClientEncryption.encrypt, and can be used to directly query for the data key itself against the key vault namespace.
function
object
Configuration options for making an AWS encryption key
object
Configuration options for making a GCP encryption key
object
Configuration options for making an Azure encryption key
object
function
object
min, max, sparsity, and range must match the values set in the encryptedFields of the destination collection. For double and decimal128, min/max/precision must all be set, or all be unset.
object
Options to provide when encrypting data.
An internal class to be used by the driver for auto encryption NOTE: Not meant to be instantiated directly, this is for internal use only.
Param | Type | Description |
---|---|---|
client | MongoClient | The client autoEncryption is enabled on |
[options] | AutoEncryptionOptions | Optional settings |
Create an AutoEncrypter
Note: Do not instantiate this class directly. Rather, supply the relevant options to a MongoClient
Note: Supplying options.schemaMap
provides more security than relying on JSON Schemas obtained from the server.
It protects against a malicious server advertising a false JSON Schema, which could trick the client into sending unencrypted data that should be encrypted.
Schemas supplied in the schemaMap only apply to configuring automatic encryption for Client-Side Field Level Encryption.
Other validation rules in the JSON schema will not be enforced by the driver and will result in an error.
Example (Create an AutoEncrypter that makes use of mongocryptd)
// Enabling autoEncryption via a MongoClient using mongocryptd
const { MongoClient } = require('mongodb');
const client = new MongoClient(URL, {
autoEncryption: {
kmsProviders: {
aws: {
accessKeyId: AWS_ACCESS_KEY,
secretAccessKey: AWS_SECRET_KEY
}
}
}
});
await client.connect();
// From here on, the client will be encrypting / decrypting automatically
Example (Create an AutoEncrypter that makes use of libmongocrypt's CSFLE shared library)
// Enabling autoEncryption via a MongoClient using CSFLE shared library
const { MongoClient } = require('mongodb');
const client = new MongoClient(URL, {
autoEncryption: {
kmsProviders: {
aws: {}
},
extraOptions: {
cryptSharedLibPath: '/path/to/local/crypt/shared/lib',
cryptSharedLibRequired: true
}
}
});
await client.connect();
// From here on, the client will be encrypting / decrypting automatically
Return the current libmongocrypt's CSFLE shared library version
as { version: bigint, versionStr: string }
, or null
if no CSFLE
shared library was loaded.
Ask the user for KMS credentials.
This returns anything that looks like the kmsProviders original input option. It can be empty, and any provider specified here will override the original ones.
The level of severity of the log message
Value | Level |
---|---|
0 | Fatal Error |
1 | Error |
2 | Warning |
3 | Info |
4 | Trace |
Properties
Name | Type | Description |
---|---|---|
[keyVaultClient] | MongoClient | A MongoClient used to fetch keys from a key vault |
[keyVaultNamespace] | string | The namespace where keys are stored in the key vault |
[kmsProviders] | KMSProviders | Configuration options that are used by specific KMS providers during key generation, encryption, and decryption. |
[schemaMap] | object | A map of namespaces to a local JSON schema for encryption |
[bypassAutoEncryption] | boolean | Allows the user to bypass auto encryption, maintaining implicit decryption |
[options.logger] | logger | An optional hook to catch logging messages from the underlying encryption engine |
[extraOptions] | AutoEncryptionExtraOptions | Extra options related to the mongocryptd process |
Configuration options for a automatic client encryption.
Properties
Name | Type | Default | Description |
---|---|---|---|
[mongocryptdURI] | string | A local process the driver communicates with to determine how to encrypt values in a command. Defaults to "mongodb://%2Fvar%2Fmongocryptd.sock" if domain sockets are available or "mongodb://localhost:27020" otherwise | |
[mongocryptdBypassSpawn] | boolean | false | If true, autoEncryption will not attempt to spawn a mongocryptd before connecting |
[mongocryptdSpawnPath] | string | The path to the mongocryptd executable on the system | |
[mongocryptdSpawnArgs] | Array.<string> | Command line arguments to use when auto-spawning a mongocryptd | |
[cryptSharedLibPath] | string | Full path to a MongoDB Crypt shared library on the system. If specified, autoEncryption will not attempt to spawn a mongocryptd, but makes use of the shared library file specified. Note that the path must point to the shared libary file itself, not the folder which contains it * | |
[cryptSharedLibRequired] | boolean | If true, never use mongocryptd and fail when the MongoDB Crypt shared libary cannot be loaded. Defaults to true if [cryptSharedLibPath] is specified and false otherwise * |
Extra options related to the mongocryptd process * Available in MongoDB 6.0 or higher.
Param | Type | Description |
---|---|---|
level | logLevel | The level of logging. |
message | string | The message to log |
A callback that is invoked with logging information from the underlying C++ Bindings.
Deprecated
instance
inner
Param | Type | Description |
---|---|---|
client | MongoClient | The client used for encryption |
options | object | Additional settings |
options.keyVaultNamespace | string | The namespace of the key vault, used to store encryption keys |
options.tlsOptions | object | An object that maps KMS provider names to TLS options. |
[options.keyVaultClient] | MongoClient | A MongoClient used to fetch keys from a key vault. Defaults to client |
[options.kmsProviders] | KMSProviders | options for specific KMS providers to use |
Create a new encryption instance
Example
new ClientEncryption(mongoClient, {
keyVaultNamespace: 'client.encryption',
kmsProviders: {
local: {
key: masterKey // The master key used for encryption/decryption. A 96-byte long Buffer
}
}
});
Example
new ClientEncryption(mongoClient, {
keyVaultNamespace: 'client.encryption',
kmsProviders: {
aws: {
accessKeyId: AWS_ACCESS_KEY,
secretAccessKey: AWS_SECRET_KEY
}
}
});
Param | Type | Description |
---|---|---|
provider | string | The KMS provider used for this data key. Must be 'aws' , 'azure' , 'gcp' , or 'local' |
[options] | object | Options for creating the data key |
[options.masterKey] | AWSEncryptionKeyOptions | AzureEncryptionKeyOptions | GCPEncryptionKeyOptions | Idenfities a new KMS-specific key used to encrypt the new data key |
[options.keyAltNames] | Array.<string> | An optional list of string alternate names used to reference a key. If a key is created with alternate names, then encryption may refer to the key by the unique alternate name instead of by _id. |
[callback] | ClientEncryptionCreateDataKeyCallback | DEPRECATED - Callbacks will be removed in the next major version. Optional callback to invoke when key is created |
Creates a data key used for explicit encryption and inserts it into the key vault namespace
Returns: Promise
| void
- If no callback is provided, returns a Promise that either resolves with the id of the created data key, or rejects with an error. If a callback is provided, returns nothing.
Example
// Using callbacks to create a local key
clientEncryption.createDataKey('local', (err, dataKey) => {
if (err) {
// This means creating the key failed.
} else {
// key creation succeeded
}
});
Example
// Using async/await to create a local key
const dataKeyId = await clientEncryption.createDataKey('local');
Example
// Using async/await to create an aws key
const dataKeyId = await clientEncryption.createDataKey('aws', {
masterKey: {
region: 'us-east-1',
key: 'xxxxxxxxxxxxxx' // CMK ARN here
}
});
Example
// Using async/await to create an aws key with a keyAltName
const dataKeyId = await clientEncryption.createDataKey('aws', {
masterKey: {
region: 'us-east-1',
key: 'xxxxxxxxxxxxxx' // CMK ARN here
},
keyAltNames: [ 'mySpecialKey' ]
});
Param | Type | Description |
---|---|---|
filter | object | A valid MongoDB filter. Any documents matching this filter will be re-wrapped. |
[options] | object | |
options.provider | KmsProvider | The KMS provider to use when re-wrapping the data keys. |
[options.masterKey] | AWSEncryptionKeyOptions | AzureEncryptionKeyOptions | GCPEncryptionKeyOptions |
Searches the keyvault for any data keys matching the provided filter. If there are matches, rewrapManyDataKey then attempts to re-wrap the data keys using the provided options.
If no matches are found, then no bulk write is performed.
Example
// rewrapping all data data keys (using a filter that matches all documents)
const filter = {};
const result = await clientEncryption.rewrapManyDataKey(filter);
if (result.bulkWriteResult != null) {
// keys were re-wrapped, results will be available in the bulkWrite object.
}
Example
// attempting to rewrap all data keys with no matches
const filter = { _id: new Binary() } // assume _id matches no documents in the database
const result = await clientEncryption.rewrapManyDataKey(filter);
if (result.bulkWriteResult == null) {
// no keys matched, `bulkWriteResult` does not exist on the result object
}
Param | Type | Description |
---|---|---|
_id | ClientEncryptionDataKeyId | the id of the document to delete. |
Deletes the key with the provided id from the keyvault, if it exists.
Returns: Promise.<DeleteResult>
- Returns a promise that either resolves to a DeleteResult or rejects with an error.
Example
// delete a key by _id
const id = new Binary(); // id is a bson binary subtype 4 object
const { deletedCount } = await clientEncryption.deleteKey(id);
if (deletedCount != null && deletedCount > 0) {
// successful deletion
}
Finds all the keys currently stored in the keyvault.
This method will not throw.
Returns: FindCursor
- a FindCursor over all keys in the keyvault.
Example
// fetching all keys
const keys = await clientEncryption.getKeys().toArray();
Param | Type | Description |
---|---|---|
_id | ClientEncryptionDataKeyId | the id of the document to delete. |
Finds a key in the keyvault with the specified _id.
Returns: Promise.<DataKey>
- Returns a promise that either resolves to a DataKey if a document matches the key or null if no documents
match the id. The promise rejects with an error if an error is thrown.
Example
// getting a key by id
const id = new Binary(); // id is a bson binary subtype 4 object
const key = await clientEncryption.getKey(id);
if (!key) {
// key is null if there was no matching key
}
Param | Type | Description |
---|---|---|
keyAltName | string | a keyAltName to search for a key |
Finds a key in the keyvault which has the specified keyAltName.
Returns: Promise.<(DataKey|null)>
- Returns a promise that either resolves to a DataKey if a document matches the key or null if no documents
match the keyAltName. The promise rejects with an error if an error is thrown.
Example
// get a key by alt name
const keyAltName = 'keyAltName';
const key = await clientEncryption.getKeyByAltName(keyAltName);
if (!key) {
// key is null if there is no matching key
}
Param | Type | Description |
---|---|---|
_id | ClientEncryptionDataKeyId | The id of the document to update. |
keyAltName | string | a keyAltName to search for a key |
Adds a keyAltName to a key identified by the provided _id.
This method resolves to/returns the old key value (prior to adding the new altKeyName).
Returns: Promise.<DataKey>
- Returns a promise that either resolves to a DataKey if a document matches the key or null if no documents
match the id. The promise rejects with an error if an error is thrown.
Example
// adding an keyAltName to a data key
const id = new Binary(); // id is a bson binary subtype 4 object
const keyAltName = 'keyAltName';
const oldKey = await clientEncryption.addKeyAltName(id, keyAltName);
if (!oldKey) {
// null is returned if there is no matching document with an id matching the supplied id
}
Param | Type | Description |
---|---|---|
_id | ClientEncryptionDataKeyId | The id of the document to update. |
keyAltName | string | a keyAltName to search for a key |
Adds a keyAltName to a key identified by the provided _id.
This method resolves to/returns the old key value (prior to removing the new altKeyName).
If the removed keyAltName is the last keyAltName for that key, the altKeyNames
property is unset from the document.
Returns: Promise.<(DataKey|null)>
- Returns a promise that either resolves to a DataKey if a document matches the key or null if no documents
match the id. The promise rejects with an error if an error is thrown.
Example
// removing a key alt name from a data key
const id = new Binary(); // id is a bson binary subtype 4 object
const keyAltName = 'keyAltName';
const oldKey = await clientEncryption.removeKeyAltName(id, keyAltName);
if (!oldKey) {
// null is returned if there is no matching document with an id matching the supplied id
}
Throws:
MongoCryptCreateDataKeyError
- If part way through the process a createDataKey invocation fails, an error will be rejected that has the partial encryptedFields
that were created.MongoCryptCreateEncryptedCollectionError
- If creating the collection fails, an error will be rejected that has the entire encryptedFields
that were created.Param | Type | Description |
---|---|---|
db | Db | A Node.js driver Db object with which to create the collection |
name | string | The name of the collection to be created |
options | object | Options for createDataKey and for createCollection |
options.provider | string | KMS provider name |
[options.masterKey] | AWSEncryptionKeyOptions | AzureEncryptionKeyOptions | GCPEncryptionKeyOptions | masterKey to pass to createDataKey |
options.createCollectionOptions | CreateCollectionOptions | options to pass to createCollection, must include encryptedFields |
A convenience method for creating an encrypted collection.
This method will create data keys for any encryptedFields that do not have a keyId
defined
and then create a new collection with the full set of encryptedFields.
Returns: Promise.<{collection: Collection.<TSchema>, encryptedFields: Document}>
- - created collection and generated encryptedFields
Param | Type | Description |
---|---|---|
value | * | The value that you wish to serialize. Must be of a type that can be serialized into BSON |
options | EncryptOptions | |
[callback] | ClientEncryptionEncryptCallback | DEPRECATED: Callbacks will be removed in the next major version. Optional callback to invoke when value is encrypted |
Explicitly encrypt a provided value. Note that either options.keyId
or options.keyAltName
must
be specified. Specifying both options.keyId
and options.keyAltName
is considered an error.
Returns: Promise
| void
- If no callback is provided, returns a Promise that either resolves with the encrypted value, or rejects with an error. If a callback is provided, returns nothing.
Example
// Encryption with callback API
function encryptMyData(value, callback) {
clientEncryption.createDataKey('local', (err, keyId) => {
if (err) {
return callback(err);
}
clientEncryption.encrypt(value, { keyId, algorithm: 'AEAD_AES_256_CBC_HMAC_SHA_512-Deterministic' }, callback);
});
}
Example
// Encryption with async/await api
async function encryptMyData(value) {
const keyId = await clientEncryption.createDataKey('local');
return clientEncryption.encrypt(value, { keyId, algorithm: 'AEAD_AES_256_CBC_HMAC_SHA_512-Deterministic' });
}
Example
// Encryption using a keyAltName
async function encryptMyData(value) {
await clientEncryption.createDataKey('local', { keyAltNames: 'mySpecialKey' });
return clientEncryption.encrypt(value, { keyAltName: 'mySpecialKey', algorithm: 'AEAD_AES_256_CBC_HMAC_SHA_512-Deterministic' });
}
Experimental: The Range algorithm is experimental only. It is not intended for production use. It is subject to breaking changes.
Param | Type | Description |
---|---|---|
expression | object | a BSON document of one of the following forms: 1. A Match Expression of this form: {$and: [{<field>: {$gt: <value1>}}, {<field>: {$lt: <value2> }}]} 2. An Aggregate Expression of this form: {$and: [{$gt: [<fieldpath>, <value1>]}, {$lt: [<fieldpath>, <value2>]}]} $gt may also be $gte . $lt may also be $lte . |
options | EncryptOptions |
Encrypts a Match Expression or Aggregate Expression to query a range index.
Only supported when queryType is "rangePreview" and algorithm is "RangePreview".
Returns: Promise.<object>
- Returns a Promise that either resolves with the encrypted value or rejects with an error.
Param | Type | Description |
---|---|---|
value | Buffer | Binary | An encrypted value |
callback | decryptCallback | DEPRECATED - Callbacks will be removed in the next major version. Optional callback to invoke when value is decrypted |
Explicitly decrypt a provided encrypted value
Returns: Promise
| void
- If no callback is provided, returns a Promise that either resolves with the decrypted value, or rejects with an error. If a callback is provided, returns nothing.
Example
// Decrypting value with callback API
function decryptMyValue(value, callback) {
clientEncryption.decrypt(value, callback);
}
Example
// Decrypting value with async/await API
async function decryptMyValue(value) {
return clientEncryption.decrypt(value);
}
Ask the user for KMS credentials.
This returns anything that looks like the kmsProviders original input option. It can be empty, and any provider specified here will override the original ones.
Deprecated
Param | Type | Description |
---|---|---|
[err] | Error | If present, indicates an error that occurred in the process of decryption |
[result] | object | If present, is the decrypted result |
Deprecated
An error indicating that something went wrong specifically with MongoDB Client Encryption
Deprecated
An error indicating that ClientEncryption.createEncryptedCollection()
failed to create data keys
Deprecated
An error indicating that ClientEncryption.createEncryptedCollection()
failed to create a collection
Deprecated
An error indicating that mongodb-client-encryption failed to auto-refresh Azure KMS credentials.
Param | Type |
---|---|
message | string |
body | object | undefined |
Deprecated
any serializable BSON value
A 64 bit integer, represented by the js-bson Long type.
Properties
Name | Type | Description |
---|---|---|
[aws] | object | Configuration options for using 'aws' as your KMS provider |
[aws.accessKeyId] | string | The access key used for the AWS KMS provider |
[aws.secretAccessKey] | string | The secret access key used for the AWS KMS provider |
[local] | object | Configuration options for using 'local' as your KMS provider |
[local.key] | Buffer | The master key used to encrypt/decrypt data keys. A 96-byte long Buffer. |
[azure] | object | Configuration options for using 'azure' as your KMS provider |
[azure.tenantId] | string | The tenant ID identifies the organization for the account |
[azure.clientId] | string | The client ID to authenticate a registered application |
[azure.clientSecret] | string | The client secret to authenticate a registered application |
[azure.identityPlatformEndpoint] | string | If present, a host with optional port. E.g. "example.com" or "example.com:443". This is optional, and only needed if customer is using a non-commercial Azure instance (e.g. a government or China account, which use different URLs). Defaults to "login.microsoftonline.com" |
[gcp] | object | Configuration options for using 'gcp' as your KMS provider |
[gcp.email] | string | The service account email to authenticate |
[gcp.privateKey] | string | Binary | A PKCS#8 encrypted key. This can either be a base64 string or a binary representation |
[gcp.endpoint] | string | If present, a host with optional port. E.g. "example.com" or "example.com:443". Defaults to "oauth2.googleapis.com" |
Configuration options that are used by specific KMS providers during key generation, encryption, and decryption.
Properties
Name | Type | Description |
---|---|---|
_id | UUID | A unique identifier for the key. |
version | number | A numeric identifier for the schema version of this document. Implicitly 0 if unset. |
[keyAltNames] | Array.<string> | Alternate names to search for keys by. Used for a per-document key scenario in support of GDPR scenarios. |
keyMaterial | Binary | Encrypted data key material, BinData type General. |
creationDate | Date | The datetime the wrapped data key material was imported into the Key Database. |
updateDate | Date | The datetime the wrapped data key material was last modified. On initial import, this value will be set to creationDate. |
status | number | 0 = enabled, 1 = disabled |
masterKey | object | the encrypted master key |
A data key as stored in the database.
A string containing the name of a kms provider. Valid options are 'aws', 'azure', 'gcp', 'kmip', or 'local'
The ClientSession class from the MongoDB Node driver (see https://mongodb.github.io/node-mongodb-native/4.8/classes/ClientSession.html)
Properties
Name | Type | Description |
---|---|---|
acknowledged | boolean | Indicates whether this write result was acknowledged. If not, then all other members of this result will be undefined. |
deletedCount | number | The number of documents that were deleted |
The result of a delete operation from the MongoDB Node driver (see https://mongodb.github.io/node-mongodb-native/4.8/interfaces/DeleteResult.html)
The BulkWriteResult class from the MongoDB Node driver (https://mongodb.github.io/node-mongodb-native/4.8/classes/BulkWriteResult.html)
The FindCursor class from the MongoDB Node driver (see https://mongodb.github.io/node-mongodb-native/4.8/classes/FindCursor.html)
The id of an existing dataKey. Is a bson Binary value. Can be used for ClientEncryption.encrypt, and can be used to directly query for the data key itself against the key vault namespace.
Deprecated
Param | Type | Description |
---|---|---|
[error] | Error | If present, indicates an error that occurred in the creation of the data key |
[dataKeyId] | ClientEncryption~dataKeyId | If present, returns the id of the created data key |
Properties
Name | Type | Description |
---|---|---|
region | string | The AWS region of the KMS |
key | string | The Amazon Resource Name (ARN) to the AWS customer master key (CMK) |
[endpoint] | string | An alternate host to send KMS requests to. May include port number |
Configuration options for making an AWS encryption key
Properties
Name | Type | Description |
---|---|---|
projectId | string | GCP project id |
location | string | Location name (e.g. "global") |
keyRing | string | Key ring name |
keyName | string | Key name |
[keyVersion] | string | Key version |
[endpoint] | string | KMS URL, defaults to https://www.googleapis.com/auth/cloudkms |
Configuration options for making a GCP encryption key
Properties
Name | Type | Description |
---|---|---|
keyName | string | Key name |
keyVaultEndpoint | string | Key vault URL, typically <name>.vault.azure.net |
[keyVersion] | string | Key version |
Configuration options for making an Azure encryption key
Properties
Name | Type | Description |
---|---|---|
[bulkWriteResult] | BulkWriteResult | An optional BulkWriteResult, if any keys were matched and attempted to be re-wrapped. |
Deprecated
Param | Type | Description |
---|---|---|
[err] | Error | If present, indicates an error that occurred in the process of encryption |
[result] | Buffer | If present, is the encrypted result |
Properties
Name | Type | Description |
---|---|---|
min | BSONValue | is required if precision is set. |
max | BSONValue | is required if precision is set. |
sparsity | BSON.Long | |
precision | number | undefined | (may only be set for double or decimal128). |
min, max, sparsity, and range must match the values set in the encryptedFields of the destination collection. For double and decimal128, min/max/precision must all be set, or all be unset.
Properties
Name | Type | Description |
---|---|---|
[keyId] | ClientEncryptionDataKeyId | The id of the Binary dataKey to use for encryption. |
[keyAltName] | string | A unique string name corresponding to an already existing dataKey. |
[algorithm] | string | The algorithm to use for encryption. Must be either 'AEAD_AES_256_CBC_HMAC_SHA_512-Deterministic' , 'AEAD_AES_256_CBC_HMAC_SHA_512-Random' , 'Indexed' or 'Unindexed' |
[contentionFactor] | bigint | number | the contention factor. |
queryType | 'equality' | 'rangePreview' | the query type supported. only the query type equality is stable at this time. queryType rangePreview is experimental. |
[rangeOptions] | RangeOptions | (experimental) The index options for a Queryable Encryption field supporting "rangePreview" queries. |
Options to provide when encrypting data.
FAQs
Re-published version to have all prebuilds defined as npm packages without platform constraints for cross building an Electron application - Official client encryption module for the MongoDB Node.js driver
The npm package @hackolade/mongodb-client-encryption receives a total of 114 weekly downloads. As such, @hackolade/mongodb-client-encryption popularity was classified as not popular.
We found that @hackolade/mongodb-client-encryption demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.