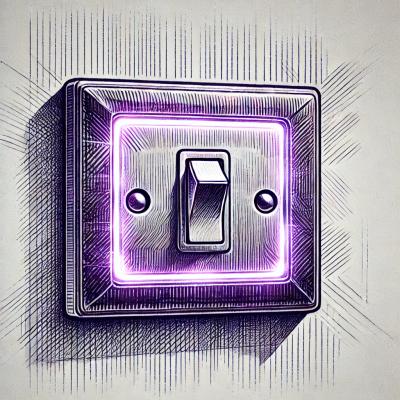
Research
Security News
Kill Switch Hidden in npm Packages Typosquatting Chalk and Chokidar
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
@interlay/interbtc-api
Advanced tools
The interBTC TypeScript library connects the Polkadot and Kusama ecosystems with Bitcoin. It allows the creation of iBTC on Polkadot and kBTC on Kusama, fungible "wrapped" tokens that represent Bitcoin. Wrapped tokens are backed by Bitcoin 1:1 and allow redeeming of the equivalent amount of Bitcoins by relying on a collateralized third-party (Vaults). In comparison to other bridge constructions (like tBTC, wBTC, or RenVM) anyone can become an intermediary by depositing collateral making interBTC the only truly open system.
The bridge itself follows the detailed specification: Explore the specification »
It is implemented as a collection of open-source Substrate modules using Rust: Explore the implementation »
You can visit bridge.interlay.io to see the library in action.
The library assumes you have a version of the Interlay or Kintsugi networks running locally or remotely.
To use the library, you will first need to create a PolkadotJS APIPromise
instance,
and then instantiate a InterBtcApi
instance.
import { createInterBtcApi } from "@interlay/interbtc";
// If you are using a local development environment
// const PARACHAIN_ENDPOINT = "ws://127.0.0.1:9944";
// if you want to use the Interlay-hosted beta network
const PARACHAIN_ENDPOINT = "wss://api.interlay.io/parachain";
const bitcoinNetwork = "mainnet";
const interBTC = await createInterBtcApi(PARACHAIN_ENDPOINT, bitcoinNetwork);
// When finished using the API, disconnect to allow Node scripts to gracefully terminate
interBTC.disconnect();
To emit transactions, an account
has to be set.
The account should conform to the AddressOrPair
interface.
If the account is not of the KeyringPair
type, then a signer must also
be provided (such as an injected extension signer, from the Polkadot wallet).
See more details here: https://polkadot.js.org/docs/extension/usage/
import { createTestKeyring } from "@polkadot/keyring/testing";
const keyring = createTestKeyring();
const keypair = keyring.getPairs()[0];
interBTC.setAccount(keypair);
The different functionalities are then exposed through the InterBtcApi
instance.
From the account you set, you can then request to issue (mint) interBTC.
import { BitcoinAmount } from "@interlay/monetary-js";
// amount of BTC to convert to interBTC
// NOTE: the bridge fees will be deducted from this. For example, if you request 1 BTC, you will receive about 0.995 interBTC
const amount = BitcoinAmount.from.BTC(0.001);
// request to issue interBTC
const requestResults = await interBTC.issue.request(amount);
// the request results includes the BTC address(es) and the BTC that should be sent to the Vault(s)
// NOTE: the library will automatically distribute issue requests across multiple Vaults if no single Vault can fulfill the request.
// Most of the time, a single Vault will be able to fulfill the request.
Send BTC using the wallet of your choice or the regtest node (see below).
To exchange interBTC back for physical BTC, you can then request to redeem (burn) interBTC.
import { BitcoinAmount } from "@interlay/monetary-js";
// the amount wrapped tokens to redeem
// NOTE: the bridge fees will be deducted from this
const amount = BitcoinAmount.from.BTC(0.001);
// your BTC address
const btcAddress = "tb123....";
// the request results includes the BTC address(es) and the BTC that should be sent to the Vault(s)
// NOTE: the library will automatically distribute redeem requests across multiple Vaults if no single Vault can fulfill the request.
// Most of the time, a single Vault will be able to fulfill the request.
const requestResults = await interBTC.redeem.request(amount, btcAddress);
One or more Vaults will send BTC to the address specified within the expiry period.
Certain API calls require a parameter of type AccountId
, which represents the Polkadot/Kusama account and can be instantiated as follows
const accountId = await interBTC.api.createType(
"AccountId",
"5Ck5SLSHYac6WFt5UZRSsdJjwmpSZq85fd5TRNAdZQVzEAPT"
);
There are many examples in the integration tests, showing how to use this library. Take a look at them here: https://github.com/interlay/interbtc-api/tree/master/test/integration
Follow Github's instructions on how to fork a repository to create your own fork.
git@github.com:<your_github_profile>/interbtc-api.git
cd interbtc-api
Start by setting your Node version via nvm
nvm use
(If necessary, nvm
will guide you on how to install the version.)
Next, install the dependencies
yarn install
Finally, build the library
yarn build
To run unit tests only, use
yarn test:unit
Note that the parachain needs to be running for all tests to run.
docker-compose up -d
The default parachain runtime is Kintsugi.
Then, to run all tests:
yarn test
yarn test:integration
NOTE: While the parachain is starting up, there will be warnings from the integration tests until it can locate the locally running test vaults. Expect the startup to take a few seconds, before the integration tests start.
At times, when running tests locally, the timeout set in package.json
's jest.testTimeout
setting might not be enough. In that case, you can override the testTimeout
value (in ms) on the command line:
yarn test:integration --testTimeout=900000
To check the logs or the services (for example, vault_1
) you can use this:
docker-compose logs -f vault_1
NOTE: The optional -f
flag attaches the terminal to the log output, you will need to Ctrl + C to exit. Alternatively, omit the flag to just get the current latest log entries.
To stop the locally running parachain, run:
docker-compose down -v
NOTE: This will remove the volumes attached to the images. So your chain will start next time in a clean/wiped state.
We only need to update types when we have changed to newer docker images for the parachain / clients.
Run the parachain (as shown above) and update the metadata:
yarn update-metadata
Then, update the metadata by building the library:
yarn build
This repository contains a number of scripts for various use cases. Check package.json
for all of the available scripts.
Query Undercollateralized borrowers
Given a PARACHAIN_ENDPOINT
(e.g. wss://api-dev-kintsugi.interlay.io/parachain
), the following will print a table with all undercollateralized borrowers on that network.
yarn install
yarn undercollateralized-borrowers --parachain-endpoint PARACHAIN_ENDPOINT
Regtest is a local Bitcoin instance that allows you to practically anything including sending transactions, mining blocks, and generating new addresses. For a full overview, head over to the Bitcoin developer documentation.
Sending Transactions
For the issue process, you need to send a transaction. On regtest this can be achieved with:
bitcoin-cli -regtest -rpcwallet=Alice sendtoaddress VAULT_ADDRESS AMOUNT
Mining Blocks
In regtest, blocks are not automatically produced. After you sent a transaction, you need to mine e.g., 1 block:
bitcoin-cli -regtest generatetoaddress 1 $(bitcoin-cli -regtest getnewaddress)
Getting Balances
You can query the balance of your wallet like so:
bitcoin-cli -regtest -rpcwallet=Alice getbalance
You can hard-reset the docker dependency setup with the following commands:
docker kill $(docker ps -q)
docker rm $(docker ps -a -q)
docker rmi $(docker images -q)
docker volume rm $(docker volume ls -q)
Contributions are what make the open source community such an amazing place to be learn, inspire, and create. Any contributions you make are greatly appreciated.
If you are searching for a place to start or would like to discuss features, reach out to us:
(C) Copyright 2022 Interlay Ltd
interbtc-js is licensed under the terms of the Apache License (Version 2.0). See LICENSE.
Website: Interlay.io
Twitter: @interlayHQ
Email: contact@interlay.io
We would like to thank the following teams for their continuous support:
FAQs
JavaScript library to interact with interBTC
The npm package @interlay/interbtc-api receives a total of 31 weekly downloads. As such, @interlay/interbtc-api popularity was classified as not popular.
We found that @interlay/interbtc-api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.