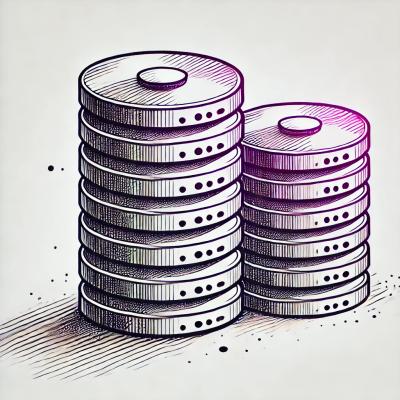
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
@itk-wasm/image-io
Advanced tools
Input and output for scientific and medical image file formats.
Input and output for scientific and medical image file formats.
npm install @itk-wasm/image-io
Import:
import {
readImage,
writeImage,
bioRadReadImage,
bioRadWriteImage,
bmpReadImage,
bmpWriteImage,
fdfReadImage,
fdfWriteImage,
gdcmReadImage,
gdcmWriteImage,
geAdwReadImage,
geAdwWriteImage,
ge4ReadImage,
ge4WriteImage,
ge5ReadImage,
ge5WriteImage,
giplReadImage,
giplWriteImage,
hdf5ReadImage,
hdf5WriteImage,
jpegReadImage,
jpegWriteImage,
lsmReadImage,
lsmWriteImage,
metaReadImage,
metaWriteImage,
mghReadImage,
mghWriteImage,
mincReadImage,
mincWriteImage,
mrcReadImage,
mrcWriteImage,
niftiReadImage,
niftiWriteImage,
nrrdReadImage,
nrrdWriteImage,
pngReadImage,
pngWriteImage,
scancoReadImage,
scancoWriteImage,
tiffReadImage,
tiffWriteImage,
vtkReadImage,
vtkWriteImage,
wasmReadImage,
wasmWriteImage,
wasmZstdReadImage,
wasmZstdWriteImage,
setPipelinesBaseUrl,
getPipelinesBaseUrl,
} from "@itk-wasm/image-io"
Read an image file format and convert it to an itk-wasm Image.
async function readImage(
serializedImage: File | BinaryFile,
options: ReadImageOptions = {}
) : Promise<ReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | File or BinaryFile | Input image serialized in the file format. |
ReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
componentType | typeof IntTypes or typeof FloatTypes | Component type, from itk-wasm IntTypes, FloatTypes, for the output pixel components. Defaults to the input component type. |
pixelType | typeof PixelTypes | Pixel type, from itk-wasm PixelTypes, for the output pixels. Defaults to the input pixel type. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
ReadImageResult
interface:
Property | Type | Description |
---|---|---|
webWorker | Worker | WebWorker used for computation |
image | Image | Output image |
Write an itk-wasm Image converted to an image file format
async function writeImage(
image: Image,
serializedImage: string,
options: WriteImageOptions = {}
) : Promise<WriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
WriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
mimeType | string | Mime type of the output image file. |
componentType | typeof IntTypes or typeof FloatTypes | Component type, from itk-wasm IntTypes, FloatTypes, for the output pixel components. Defaults to the input component type. |
pixelType | typeof PixelTypes | Pixel type, from itk-wasm PixelTypes, for the output pixels. Defaults to the input pixel type. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
WriteImageResult
interface:
Property | Type | Description |
---|---|---|
webWorker | Worker | WebWorker used for computation |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function bioRadReadImage(
serializedImage: File | BinaryFile,
options: BioRadReadImageOptions = {}
) : Promise<BioRadReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
BioRadReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
BioRadReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function bioRadWriteImage(
image: Image,
serializedImage: string,
options: BioRadWriteImageOptions = {}
) : Promise<BioRadWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
BioRadWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
BioRadWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function bmpReadImage(
serializedImage: File | BinaryFile,
options: BmpReadImageOptions = {}
) : Promise<BmpReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
BmpReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
BmpReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function bmpWriteImage(
image: Image,
serializedImage: string,
options: BmpWriteImageOptions = {}
) : Promise<BmpWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
BmpWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
BmpWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function fdfReadImage(
serializedImage: File | BinaryFile,
options: FdfReadImageOptions = {}
) : Promise<FdfReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
FdfReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
FdfReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function fdfWriteImage(
image: Image,
serializedImage: string,
options: FdfWriteImageOptions = {}
) : Promise<FdfWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
FdfWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
FdfWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function gdcmReadImage(
serializedImage: File | BinaryFile,
options: GdcmReadImageOptions = {}
) : Promise<GdcmReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
GdcmReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
GdcmReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function gdcmWriteImage(
image: Image,
serializedImage: string,
options: GdcmWriteImageOptions = {}
) : Promise<GdcmWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
GdcmWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
GdcmWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function geAdwReadImage(
serializedImage: File | BinaryFile,
options: GeAdwReadImageOptions = {}
) : Promise<GeAdwReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
GeAdwReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
GeAdwReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function geAdwWriteImage(
image: Image,
serializedImage: string,
options: GeAdwWriteImageOptions = {}
) : Promise<GeAdwWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
GeAdwWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
GeAdwWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function ge4ReadImage(
serializedImage: File | BinaryFile,
options: Ge4ReadImageOptions = {}
) : Promise<Ge4ReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
Ge4ReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
Ge4ReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function ge4WriteImage(
image: Image,
serializedImage: string,
options: Ge4WriteImageOptions = {}
) : Promise<Ge4WriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
Ge4WriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
Ge4WriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function ge5ReadImage(
serializedImage: File | BinaryFile,
options: Ge5ReadImageOptions = {}
) : Promise<Ge5ReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
Ge5ReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
Ge5ReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function ge5WriteImage(
image: Image,
serializedImage: string,
options: Ge5WriteImageOptions = {}
) : Promise<Ge5WriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
Ge5WriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
Ge5WriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function giplReadImage(
serializedImage: File | BinaryFile,
options: GiplReadImageOptions = {}
) : Promise<GiplReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
GiplReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
GiplReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function giplWriteImage(
image: Image,
serializedImage: string,
options: GiplWriteImageOptions = {}
) : Promise<GiplWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
GiplWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
GiplWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function hdf5ReadImage(
serializedImage: File | BinaryFile,
options: Hdf5ReadImageOptions = {}
) : Promise<Hdf5ReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
Hdf5ReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
Hdf5ReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function hdf5WriteImage(
image: Image,
serializedImage: string,
options: Hdf5WriteImageOptions = {}
) : Promise<Hdf5WriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
Hdf5WriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
Hdf5WriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function jpegReadImage(
serializedImage: File | BinaryFile,
options: JpegReadImageOptions = {}
) : Promise<JpegReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
JpegReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
JpegReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function jpegWriteImage(
image: Image,
serializedImage: string,
options: JpegWriteImageOptions = {}
) : Promise<JpegWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
JpegWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
JpegWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function lsmReadImage(
serializedImage: File | BinaryFile,
options: LsmReadImageOptions = {}
) : Promise<LsmReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
LsmReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
LsmReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function lsmWriteImage(
image: Image,
serializedImage: string,
options: LsmWriteImageOptions = {}
) : Promise<LsmWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
LsmWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
LsmWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function metaReadImage(
serializedImage: File | BinaryFile,
options: MetaReadImageOptions = {}
) : Promise<MetaReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
MetaReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
MetaReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function metaWriteImage(
image: Image,
serializedImage: string,
options: MetaWriteImageOptions = {}
) : Promise<MetaWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
MetaWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
MetaWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function mghReadImage(
serializedImage: File | BinaryFile,
options: MghReadImageOptions = {}
) : Promise<MghReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
MghReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
MghReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function mghWriteImage(
image: Image,
serializedImage: string,
options: MghWriteImageOptions = {}
) : Promise<MghWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
MghWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
MghWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function mincReadImage(
serializedImage: File | BinaryFile,
options: MincReadImageOptions = {}
) : Promise<MincReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
MincReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
MincReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function mincWriteImage(
image: Image,
serializedImage: string,
options: MincWriteImageOptions = {}
) : Promise<MincWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
MincWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
MincWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function mrcReadImage(
serializedImage: File | BinaryFile,
options: MrcReadImageOptions = {}
) : Promise<MrcReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
MrcReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
MrcReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function mrcWriteImage(
image: Image,
serializedImage: string,
options: MrcWriteImageOptions = {}
) : Promise<MrcWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
MrcWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
MrcWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function niftiReadImage(
serializedImage: File | BinaryFile,
options: NiftiReadImageOptions = {}
) : Promise<NiftiReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
NiftiReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
NiftiReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function niftiWriteImage(
image: Image,
serializedImage: string,
options: NiftiWriteImageOptions = {}
) : Promise<NiftiWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
NiftiWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
NiftiWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function nrrdReadImage(
serializedImage: File | BinaryFile,
options: NrrdReadImageOptions = {}
) : Promise<NrrdReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
NrrdReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
NrrdReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function nrrdWriteImage(
image: Image,
serializedImage: string,
options: NrrdWriteImageOptions = {}
) : Promise<NrrdWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
NrrdWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
NrrdWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function pngReadImage(
serializedImage: File | BinaryFile,
options: PngReadImageOptions = {}
) : Promise<PngReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
PngReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
PngReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function pngWriteImage(
image: Image,
serializedImage: string,
options: PngWriteImageOptions = {}
) : Promise<PngWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
PngWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
PngWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function scancoReadImage(
serializedImage: File | BinaryFile,
options: ScancoReadImageOptions = {}
) : Promise<ScancoReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
ScancoReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
ScancoReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function scancoWriteImage(
image: Image,
serializedImage: string,
options: ScancoWriteImageOptions = {}
) : Promise<ScancoWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
ScancoWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
ScancoWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function tiffReadImage(
serializedImage: File | BinaryFile,
options: TiffReadImageOptions = {}
) : Promise<TiffReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
TiffReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
TiffReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function tiffWriteImage(
image: Image,
serializedImage: string,
options: TiffWriteImageOptions = {}
) : Promise<TiffWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
TiffWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
TiffWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function vtkReadImage(
serializedImage: File | BinaryFile,
options: VtkReadImageOptions = {}
) : Promise<VtkReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
VtkReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
VtkReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function vtkWriteImage(
image: Image,
serializedImage: string,
options: VtkWriteImageOptions = {}
) : Promise<VtkWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
VtkWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
VtkWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function wasmReadImage(
serializedImage: File | BinaryFile,
options: WasmReadImageOptions = {}
) : Promise<WasmReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
WasmReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
WasmReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function wasmWriteImage(
image: Image,
serializedImage: string,
options: WasmWriteImageOptions = {}
) : Promise<WasmWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
WasmWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
WasmWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Read an image file format and convert it to the itk-wasm file format
async function wasmZstdReadImage(
serializedImage: File | BinaryFile,
options: WasmZstdReadImageOptions = {}
) : Promise<WasmZstdReadImageResult>
Parameter | Type | Description |
---|---|---|
serializedImage | *File | BinaryFile* |
WasmZstdReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
WasmZstdReadImageResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
webWorker | Worker | WebWorker used for computation. |
Write an itk-wasm file format converted to an image file format
async function wasmZstdWriteImage(
image: Image,
serializedImage: string,
options: WasmZstdWriteImageOptions = {}
) : Promise<WasmZstdWriteImageResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
WasmZstdWriteImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
webWorker | null or Worker or boolean | WebWorker for computation. Set to null to create a new worker. Or, pass an existing worker. Or, set to false to run in the current thread / worker. |
noCopy | boolean | When SharedArrayBuffer's are not available, do not copy inputs. |
WasmZstdWriteImageResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
webWorker | Worker | WebWorker used for computation. |
Set base URL for WebAssembly assets when vendored.
function setPipelinesBaseUrl(
baseUrl: string | URL
) : void
Get base URL for WebAssembly assets when vendored.
function getPipelinesBaseUrl() : string | URL
Import:
import {
readImageNode,
writeImageNode,
bioRadReadImageNode,
bioRadWriteImageNode,
bmpReadImageNode,
bmpWriteImageNode,
fdfReadImageNode,
fdfWriteImageNode,
gdcmReadImageNode,
gdcmWriteImageNode,
geAdwReadImageNode,
geAdwWriteImageNode,
ge4ReadImageNode,
ge4WriteImageNode,
ge5ReadImageNode,
ge5WriteImageNode,
giplReadImageNode,
giplWriteImageNode,
hdf5ReadImageNode,
hdf5WriteImageNode,
jpegReadImageNode,
jpegWriteImageNode,
lsmReadImageNode,
lsmWriteImageNode,
metaReadImageNode,
metaWriteImageNode,
mghReadImageNode,
mghWriteImageNode,
mincReadImageNode,
mincWriteImageNode,
mrcReadImageNode,
mrcWriteImageNode,
niftiReadImageNode,
niftiWriteImageNode,
nrrdReadImageNode,
nrrdWriteImageNode,
pngReadImageNode,
pngWriteImageNode,
scancoReadImageNode,
scancoWriteImageNode,
tiffReadImageNode,
tiffWriteImageNode,
vtkReadImageNode,
vtkWriteImageNode,
wasmReadImageNode,
wasmWriteImageNode,
wasmZstdReadImageNode,
wasmZstdWriteImageNode,
} from "@itk-wasm/image-io"
Read an image file format and convert it to an itk-wasm Image.
async function readImageNode(
serializedImage: string,
options: ReadImageOptions = {}
) : Promise<Image>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format. |
ReadImageOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
componentType | typeof IntTypes or typeof FloatTypes | Component type, from itk-wasm IntTypes, FloatTypes, for the output pixel components. Defaults to the input component type. |
pixelType | typeof PixelTypes | Pixel type, from itk-wasm PixelTypes, for the output pixels. Defaults to the input pixel type. |
Write an itk-wasm Image converted to an image file format
async function writeImageNode(
image: Image,
serializedImage: string,
options: WriteImageOptions = {}
) : void
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function bioRadReadImageNode(
serializedImage: string,
options: BioRadReadImageNodeOptions = {}
) : Promise<BioRadReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
BioRadReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
BioRadReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function bioRadWriteImageNode(
image: Image,
serializedImage: string,
options: BioRadWriteImageNodeOptions = {}
) : Promise<BioRadWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
BioRadWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
BioRadWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function bmpReadImageNode(
serializedImage: string,
options: BmpReadImageNodeOptions = {}
) : Promise<BmpReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
BmpReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
BmpReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function bmpWriteImageNode(
image: Image,
serializedImage: string,
options: BmpWriteImageNodeOptions = {}
) : Promise<BmpWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
BmpWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
BmpWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function fdfReadImageNode(
serializedImage: string,
options: FdfReadImageNodeOptions = {}
) : Promise<FdfReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
FdfReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
FdfReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function fdfWriteImageNode(
image: Image,
serializedImage: string,
options: FdfWriteImageNodeOptions = {}
) : Promise<FdfWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
FdfWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
FdfWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function gdcmReadImageNode(
serializedImage: string,
options: GdcmReadImageNodeOptions = {}
) : Promise<GdcmReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
GdcmReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
GdcmReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function gdcmWriteImageNode(
image: Image,
serializedImage: string,
options: GdcmWriteImageNodeOptions = {}
) : Promise<GdcmWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
GdcmWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
GdcmWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function geAdwReadImageNode(
serializedImage: string,
options: GeAdwReadImageNodeOptions = {}
) : Promise<GeAdwReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
GeAdwReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
GeAdwReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function geAdwWriteImageNode(
image: Image,
serializedImage: string,
options: GeAdwWriteImageNodeOptions = {}
) : Promise<GeAdwWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
GeAdwWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
GeAdwWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function ge4ReadImageNode(
serializedImage: string,
options: Ge4ReadImageNodeOptions = {}
) : Promise<Ge4ReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
Ge4ReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
Ge4ReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function ge4WriteImageNode(
image: Image,
serializedImage: string,
options: Ge4WriteImageNodeOptions = {}
) : Promise<Ge4WriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
Ge4WriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
Ge4WriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function ge5ReadImageNode(
serializedImage: string,
options: Ge5ReadImageNodeOptions = {}
) : Promise<Ge5ReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
Ge5ReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
Ge5ReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function ge5WriteImageNode(
image: Image,
serializedImage: string,
options: Ge5WriteImageNodeOptions = {}
) : Promise<Ge5WriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
Ge5WriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
Ge5WriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function giplReadImageNode(
serializedImage: string,
options: GiplReadImageNodeOptions = {}
) : Promise<GiplReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
GiplReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
GiplReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function giplWriteImageNode(
image: Image,
serializedImage: string,
options: GiplWriteImageNodeOptions = {}
) : Promise<GiplWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
GiplWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
GiplWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function hdf5ReadImageNode(
serializedImage: string,
options: Hdf5ReadImageNodeOptions = {}
) : Promise<Hdf5ReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
Hdf5ReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
Hdf5ReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function hdf5WriteImageNode(
image: Image,
serializedImage: string,
options: Hdf5WriteImageNodeOptions = {}
) : Promise<Hdf5WriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
Hdf5WriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
Hdf5WriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function jpegReadImageNode(
serializedImage: string,
options: JpegReadImageNodeOptions = {}
) : Promise<JpegReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
JpegReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
JpegReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function jpegWriteImageNode(
image: Image,
serializedImage: string,
options: JpegWriteImageNodeOptions = {}
) : Promise<JpegWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
JpegWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
JpegWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function lsmReadImageNode(
serializedImage: string,
options: LsmReadImageNodeOptions = {}
) : Promise<LsmReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
LsmReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
LsmReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function lsmWriteImageNode(
image: Image,
serializedImage: string,
options: LsmWriteImageNodeOptions = {}
) : Promise<LsmWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
LsmWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
LsmWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function metaReadImageNode(
serializedImage: string,
options: MetaReadImageNodeOptions = {}
) : Promise<MetaReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
MetaReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
MetaReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function metaWriteImageNode(
image: Image,
serializedImage: string,
options: MetaWriteImageNodeOptions = {}
) : Promise<MetaWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
MetaWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
MetaWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function mghReadImageNode(
serializedImage: string,
options: MghReadImageNodeOptions = {}
) : Promise<MghReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
MghReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
MghReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function mghWriteImageNode(
image: Image,
serializedImage: string,
options: MghWriteImageNodeOptions = {}
) : Promise<MghWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
MghWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
MghWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function mincReadImageNode(
serializedImage: string,
options: MincReadImageNodeOptions = {}
) : Promise<MincReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
MincReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
MincReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function mincWriteImageNode(
image: Image,
serializedImage: string,
options: MincWriteImageNodeOptions = {}
) : Promise<MincWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
MincWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
MincWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function mrcReadImageNode(
serializedImage: string,
options: MrcReadImageNodeOptions = {}
) : Promise<MrcReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
MrcReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
MrcReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function mrcWriteImageNode(
image: Image,
serializedImage: string,
options: MrcWriteImageNodeOptions = {}
) : Promise<MrcWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
MrcWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
MrcWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function niftiReadImageNode(
serializedImage: string,
options: NiftiReadImageNodeOptions = {}
) : Promise<NiftiReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
NiftiReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
NiftiReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function niftiWriteImageNode(
image: Image,
serializedImage: string,
options: NiftiWriteImageNodeOptions = {}
) : Promise<NiftiWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
NiftiWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
NiftiWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function nrrdReadImageNode(
serializedImage: string,
options: NrrdReadImageNodeOptions = {}
) : Promise<NrrdReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
NrrdReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
NrrdReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function nrrdWriteImageNode(
image: Image,
serializedImage: string,
options: NrrdWriteImageNodeOptions = {}
) : Promise<NrrdWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
NrrdWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
NrrdWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function pngReadImageNode(
serializedImage: string,
options: PngReadImageNodeOptions = {}
) : Promise<PngReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
PngReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
PngReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function pngWriteImageNode(
image: Image,
serializedImage: string,
options: PngWriteImageNodeOptions = {}
) : Promise<PngWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
PngWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
PngWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function scancoReadImageNode(
serializedImage: string,
options: ScancoReadImageNodeOptions = {}
) : Promise<ScancoReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
ScancoReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
ScancoReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function scancoWriteImageNode(
image: Image,
serializedImage: string,
options: ScancoWriteImageNodeOptions = {}
) : Promise<ScancoWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
ScancoWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
ScancoWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function tiffReadImageNode(
serializedImage: string,
options: TiffReadImageNodeOptions = {}
) : Promise<TiffReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
TiffReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
TiffReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function tiffWriteImageNode(
image: Image,
serializedImage: string,
options: TiffWriteImageNodeOptions = {}
) : Promise<TiffWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
TiffWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
TiffWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function vtkReadImageNode(
serializedImage: string,
options: VtkReadImageNodeOptions = {}
) : Promise<VtkReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
VtkReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
VtkReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function vtkWriteImageNode(
image: Image,
serializedImage: string,
options: VtkWriteImageNodeOptions = {}
) : Promise<VtkWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
VtkWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
VtkWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function wasmReadImageNode(
serializedImage: string,
options: WasmReadImageNodeOptions = {}
) : Promise<WasmReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
WasmReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
WasmReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function wasmWriteImageNode(
image: Image,
serializedImage: string,
options: WasmWriteImageNodeOptions = {}
) : Promise<WasmWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
WasmWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
WasmWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
Read an image file format and convert it to the itk-wasm file format
async function wasmZstdReadImageNode(
serializedImage: string,
options: WasmZstdReadImageNodeOptions = {}
) : Promise<WasmZstdReadImageNodeResult>
Parameter | Type | Description |
---|---|---|
serializedImage | string | Input image serialized in the file format |
WasmZstdReadImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only read image metadata -- do not read pixel data. |
WasmZstdReadImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldRead | JsonCompatible | Whether the input could be read. If false, the output image is not valid. |
image | Image | Output image |
Write an itk-wasm file format converted to an image file format
async function wasmZstdWriteImageNode(
image: Image,
serializedImage: string,
options: WasmZstdWriteImageNodeOptions = {}
) : Promise<WasmZstdWriteImageNodeResult>
Parameter | Type | Description |
---|---|---|
image | Image | Input image |
serializedImage | string | Output image serialized in the file format. |
WasmZstdWriteImageNodeOptions
interface:
Property | Type | Description |
---|---|---|
informationOnly | boolean | Only write image metadata -- do not write pixel data. |
useCompression | boolean | Use compression in the written file |
WasmZstdWriteImageNodeResult
interface:
Property | Type | Description |
---|---|---|
couldWrite | JsonCompatible | Whether the input could be written. If false, the output image is not valid. |
serializedImage | BinaryFile | Output image serialized in the file format. |
FAQs
Input and output for scientific and medical image file formats.
The npm package @itk-wasm/image-io receives a total of 442 weekly downloads. As such, @itk-wasm/image-io popularity was classified as not popular.
We found that @itk-wasm/image-io demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.