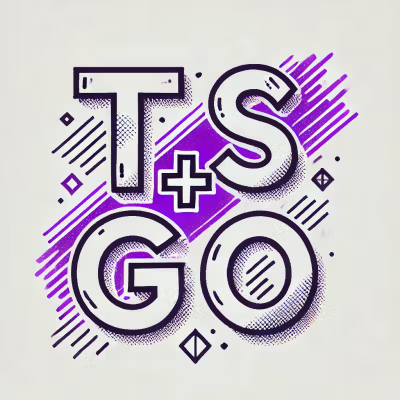
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
@ixnode/geo-sphere
Advanced tools
A Node.js package for rendering interactive world maps with Mercator projection, customizable languages, and advanced interactivity features such as zoom, pan, and click events.
@ixnode/geo-sphere
is a powerful and flexible Node.js package for rendering interactive maps. It supports various
projections (currently only Mercator projection - more are planned), languages, and advanced interactivity, allowing
you to display geographical points and create a fully interactive mapping experience.
cz
|de
|en
|es
|fr
|hr
|it
|pl
|sv
.npm install @ixnode/geo-sphere
or
yarn add @ixnode/geo-sphere
import React from 'react';
import { WorldMap } from '@ixnode/geo-sphere';
import '@ixnode/geo-sphere/dist/styles.css';
const App = () => (
<WorldMap
height={500}
width={1000}
country="de"
language="en"
/>
);
export default App;
import React from 'react';
import { WorldMap, CountryData } from '@ixnode/geo-sphere';
import '@ixnode/geo-sphere/dist/styles.css';
const App = () => (
<WorldMap
height={500}
width={1000}
country="de"
language="en"
dataSource="medium"
onClickCountry={(data: CountryData) => { console.log(data); }}
/>
);
export default App;
The callback function logs something like (according to the clicked country and the given language):
{
"id": "de",
"name": "Germany",
"latitude": 50.304018990688554,
"longitude": 7.5794992771470975,
"screenPosition": {
"x": 401,
"y": 333
},
"svgPosition": {
"x": 843746,
"y": 6499094
}
}
Property | Description |
---|---|
id | The id of clicked element. |
name | The translated name of clicked element. |
latitude | The latitude value. Clicked on the map. |
longitude | The longitude value. Clicked on the map. |
screenPosition.x | The x position of the last click. Related to the screen. |
screenPosition.y | The y position of the last click. Related to the screen. |
svgPosition.x | The x position of the last click. Related to the whole svg map. |
svgPosition.y | The x position of the last click. Related to the whole svg map. |
import React from 'react';
import { WorldMap, PlaceData } from '@ixnode/geo-sphere';
import '@ixnode/geo-sphere/dist/styles.css';
const App = () => (
<WorldMap
height={500}
width={1000}
country="de"
language="en"
dataSource="medium"
onClickPlace={(data: PlaceData) => { console.log(data); }}
/>
);
export default App;
The callback function logs something like (according to the clicked place and the given language):
{
"id": "place-berlin",
"latitude": 52.5235,
"longitude": 13.4115,
"name": "Berlin",
"population": 3662381,
"country": {
"id": "de",
"name": "Germany"
},
"state": {
"id": "de-be",
"name": "Berlin",
"area": 891.1,
"population": 3662381
},
"screenPosition": {
"x": 629,
"y": 295
},
"svgPosition": {
"x": 1580.7000732421875,
"y": 6909.73095703125
}
}
Property | Description |
---|---|
id | The id of clicked element. |
name | The translated name of clicked element. |
population | The population of clicked element. |
latitude | The latitude value. Clicked on the map. |
longitude | The longitude value. Clicked on the map. |
screenPosition.x | The x position of the last click. Related to the screen. |
screenPosition.y | The y position of the last click. Related to the screen. |
svgPosition.x | The x position of the last click. Related to the whole svg map. |
svgPosition.y | The x position of the last click. Related to the whole svg map. |
Property | Type | Default | Description |
---|---|---|---|
dataSource | 'tiny'|'low'|'medium' | 'low' | The data source to be used. |
country | string|null | null | The country that is marked. |
width | number | 1000 | The width of the map in pixels. Only used for ratio. The svg is always 100% of parent element. |
height | number | 500 | The height of the map in pixels. Only used for ratio. The svg is always 100% of parent element. |
language | 'cz' |'de' |'en' |'es' |'fr' |'hr' |'it' |'pl' |'sv' | 'en' | The language to be used. |
onClickCountry | (data: CountryData) => void|null | null | An optional country click handler. |
onClickPlace | (data: PlaceData) => void|null | null | An optional place click handler. |
onHoverCountry | (data: CountryData) => void|null | null | An optional country hover handler. |
onHoverPlace | (data: PlaceData) => void|null | null | An optional place hover handler. |
debug | boolean | false | Flag to enable or disable the debug mode. |
logo | boolean | true | Flag to enable or disable the logo. |
country
)Use ISO 3166-1 alpha-2
code to select a country. Examples:
Country | ISO code |
---|---|
United Kingdom of Great Britain and Northern Ireland | gb |
United States of America | us |
Germany | de |
Sweden | se |
etc. |
See https://en.wikipedia.org/wiki/ISO_3166-1_alpha-2 for more information.
language
)Currently supported languages:
Language | Description |
---|---|
cz | Czech |
de | German |
en | English |
es | Spanish |
fr | French |
hr | Croatian |
it | Italian |
pl | Polish |
sv | Swedish |
To build the project locally:
npm run build
View and develop components in isolation:
npm run storybook
Open: http://localhost:6006/
npx tsc --noEmit
npm run build
node dist/index.js
or to ignore possible warnings:
node --no-warnings dist/index.js
node dist/index.cjs
Update the version in the package.json, e.g., from 1.0.0 to 1.0.1, to create a new release:
npm version patch
Alternatively:
npm version minor
for new features.npm version major
for breaking changes.npm publish --access public
Check the package on npm: https://www.npmjs.com/package/@ixnode/geo-sphere.
This project is licensed under the MIT License. See the LICENSE file for details.
Contributions are welcome! Feel free to submit issues or pull requests to improve this project.
Special thanks to the open-source community for providing amazing tools like React, TypeScript, and Storybook.
FAQs
A Node.js package for rendering interactive world maps with Mercator projection, customizable languages, and advanced interactivity features such as zoom, pan, and click events.
The npm package @ixnode/geo-sphere receives a total of 100 weekly downloads. As such, @ixnode/geo-sphere popularity was classified as not popular.
We found that @ixnode/geo-sphere demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.