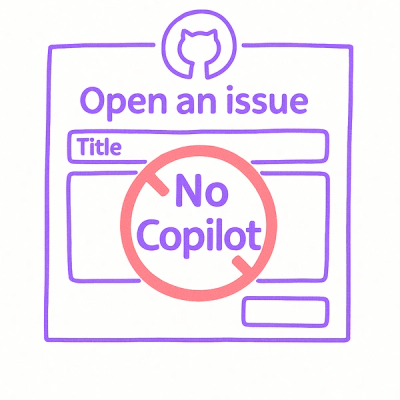
Security News
Open Source Maintainers Demand Ability to Block Copilot-Generated Issues and PRs
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
@koga73/react-overlay
Advanced tools
A simple responsive modal system for the web. Fully accessible. Easy to style.
A React implementation of https://github.com/koga73/overlay
Overlay has been designed to be completely accessible. Features are as follows:
npm install @koga73/react-overlay
JSX
import ReactOverlay from "@koga73/react-overlay";
SCSS
@import "@koga73/react-overlay";
This component can be used in a controlled or uncontrolled manner - meaning the parent controls the Overlay open state (controlled) or not (uncontrolled)
Use the controlled implementation if you want your parent to maintain the visibility of the Overlay
import React, {useState} from "react";
import ReactOverlay from "@koga73/react-overlay";
//Be sure to import Overlay styles in here: @import "@koga73/react-overlay"
//Or customize, styles can be found here: https://github.com/koga73/overlay/tree/master/css
import "./example.scss";
() => {
const [stateIsOpen, setStateIsOpen] = useState(); //You can pass in an initial value here
return (
<div>
<div data-overlay-page-wrap>
<h1>Page content</h1>
<button onClick={evt => this.setStateIsOpen(true)}>Show Overlay</button>
</div>
<div style={{display: "none"}}>
<ReactOverlay
id="myOverlay"
isOpen={isOpen}
onRequestClose={detail => this.setStateIsOpen(false)}
>
<p>Some content</p>
</ReactOverlay>
</div>
</div>
);
};
Use the uncontrolled implementation if you don't care about maintaining the Overlay shown state
import React from "react";
import ReactOverlay from "@koga73/react-overlay";
//Be sure to import Overlay styles in here: @import "@koga73/react-overlay"
//Or customize, styles can be found here: https://github.com/koga73/overlay/tree/master/css
import "./example.scss";
class App extends React.Component {
render() {
return (
<div>
<div data-overlay-page-wrap>
<h1>Page content</h1>
<button onClick={evt => ReactOverlay.show("myOverlay")}>Show Overlay</button>
</div>
<div style={{display: "none"}}>
<ReactOverlay id="myOverlay">
<p>Some content</p>
</ReactOverlay>
</div>
</div>
);
}
}
The following props are parsed out and any other props get passed-through
The following callbacks are parsed out and any other callbacks get passed-through
By default a static instance is created on the window named ReactOverlay. You can override the default values below, just call update after. Note, ReactOverlay instances will use these defaults if not defined on the instance.
Note that if using React-DOM 18, you should specify the react root as the static container. This is because React-DOM 18 uses portals to render the Overlay outside of the root element.
import React from "react";
import ReactDOM from "react-dom/client";
import ReactOverlay from "@koga73/react-overlay";
const rootEl = document.getElementById("root");
ReactDOM.createRoot(rootEl).render(<Example />);
//Specify the root element as the container when using React-DOM 18
ReactOverlay.update({container: rootEl});
FAQs
A simple responsive modal system for the web. Fully accessible. Easy to style.
The npm package @koga73/react-overlay receives a total of 5 weekly downloads. As such, @koga73/react-overlay popularity was classified as not popular.
We found that @koga73/react-overlay demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
Research
Security News
Malicious Koishi plugin silently exfiltrates messages with hex strings to a hardcoded QQ account, exposing secrets in chatbots across platforms.
Research
Security News
Malicious PyPI checkers validate stolen emails against TikTok and Instagram APIs, enabling targeted account attacks and dark web credential sales.