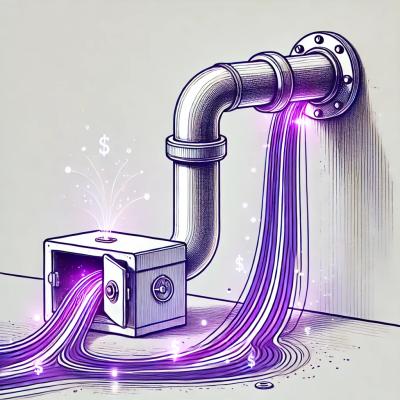
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@livechat/widget-react
Advanced tools
This library allows to render and interact with the LiveChat Chat Widget inside a React application
This library allows to render and interact with the LiveChat Chat Widget inside a React application.
Using npm:
npm install @livechat/widget-react
or using yarn:
yarn add @livechat/widget-react
import { LiveChatWidget, EventHandlerPayload } from '@livechat/widget-react'
function App() {
function handleNewEvent(event: EventHandlerPayload<'onNewEvent'>) {
console.log('LiveChatWidget.onNewEvent', event)
}
return (
<LiveChatWidget
license="12345678"
visibility="maximized"
onNewEvent={handleNewEvent}
/>
)
}
All properties described below are used for initialization on the first render and later updates of the chat widget with new values on change.
Prop | Type |
---|---|
license | string (required) |
customerName | string |
group | string |
customerEmail | string |
chatBetweenGroups | boolean |
sessionVariables | Record<string, string> |
visibility | 'maximized' | 'minimized' | 'hidden' |
customIdentityProvider | () => CustomerAuth |
CustomerAuth:
parameters | type | description |
---|---|---|
getFreshToken | () => Promise | Should resolve with freshly requested customer access token. |
getToken | () => Promise | Should resolve with currently stored customer access token. |
hasToken | () => Promise | Should resolve with a boolean value representing if a token has been already acquired. |
invalidate | () => Promise | Should handle token invalidation and/or clearing the locally cached value. |
All event handlers listed below are registered if provided for the first time. They unregister on the component cleanup or the property value change. Descriptions of all events are available after clicking on the associated links.
This package exports a set of React Hooks that allows consuming reactive data from the chat widget in any place of the application as long as the LiveChatWidget
component is rendered in the tree.
Access the current chat widget availability
or visibility
state if the chat widget is loaded.
import { useWidgetState } from '@livechat/widget-react'
function App() {
const widgetState = useWidgetState()
if (widgetState) {
return (
<div>
<span>{widgetState.availability}</span>
<span>{widgetState.visibility}</span>
</div>
)
}
}
Check if the chat widget is ready using the boolean flag isWidgetReady
.
import { useWidgetIsReady } from '@livechat/widget-react'
function App() {
const isWidgetReady = useWidgetIsReady()
return <div>Chat Widget is {isWidgetReady ? 'loaded' : 'loading...'}</div>
}
Access the chatId
and threadId
of the chat if there's one currently available.
import { useWidgetChatData } from '@livechat/widget-react'
function App() {
const chatData = useWidgetChatData()
if (chatData) {
return (
<div>
<span>{chatData.chatId}</span>
<span>{chatData.threadId}</span>
</div>
)
}
}
Access the current greeting id
and uniqueId
if one is currently displayed (received and not hidden).
import { useWidgetGreeting } from '@livechat/widget-react'
function App() {
const greeting = useWidgetGreeting()
if (greeting) {
return (
<div>
<span>{greeting.id}</span>
<span>{greeting.uniqueId}</span>
</div>
)
}
}
Access the id
, isReturning
, status
, and sessionVariables
of the current customer if the chat widget is loaded.
import { useWidgetCustomerData } from '@livechat/widget-react'
function App() {
const customerData = useWidgetCustomerData()
if (customerData) {
return (
<div>
<span>{customerData.id}</span>
<span>{customerData.isReturning}</span>
<span>{customerData.status}</span>
<ul>
{Object.entries(customerData.sessionVariables).map(([key, value]) => (
<li key={key}>{value}</li>
))}
</ul>
</div>
)
}
}
In order to make Custom Identity Provider work, you'll have to properly implement and provide a set of following methods:
getToken
- resolving Chat Widget token. If you want to cache the token, this should return the cached token instead of a fresh request to https://accounts.livechat.com/customer/token endpoint.getFreshToken
- resolving Chat Widget token. This should always make a call for a fresh token from https://accounts.livechat.com/customer/token endpoint.hasToken
- resolving boolean. It determines whether a token has been acquired.invalidate
- resolving nothing. When called, it should remove the current token. There is no need to do anything else as a new token will be requested by getFreshToken afterwards.import { LiveChatWidget } from '@livechat/widget-react'
const customIdentityProvider = () => {
const baseAPI = 'YOUR_API_URL'
const userId = '30317220-c72d-11ed-2137-0242ac120002'
const getToken = async () => {
const response = await fetch(`${baseAPI}/getToken/${userId}`)
const token = await response.json()
console.log('getToken', token)
return token
}
const getFreshToken = async () => {
const response = await fetch(`${baseAPI}/getFreshToken/${userId}`)
const token = await response.json()
console.log('getFreshToken, token')
return token
}
const hasToken = async () => {
const response = await fetch(`${baseAPI}/hasToken/${userId}`)
const data = await response.json()
return data
}
const invalidateToken = async () => {
const response = await fetch(`${baseAPI}/invalidate/${userId}`)
const data = await response.json()
console.log(data)
}
return {
getToken,
getFreshToken,
hasToken,
invalidate: invalidateToken,
}
}
function App() {
return (
<LiveChatWidget
license="12345678"
visibility="maximized"
customIdentityProvider={customIdentityProvider}
/>
)
}
For more information about Custom Identity Provider, check out https://developers.livechat.com/docs/extending-chat-widget/custom-identity-provider
Pull requests are welcome. For major changes, please open an issue first, so we can discuss what you would like to change. Follow a Contributing guide for more details.
The code and documentation in this project are released under the MIT License.
FAQs
This library allows to render and interact with the LiveChat Chat Widget inside a React application
We found that @livechat/widget-react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.