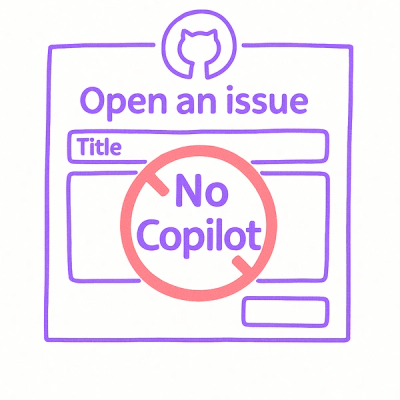
Security News
Open Source Maintainers Demand Ability to Block Copilot-Generated Issues and PRs
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
@masumdev/rn-qrcode
Advanced tools
A powerful and easy-to-use QR code scanning and generation library for React Native applications.
A powerful and easy-to-use QR code scanning and generation library for React Native applications.
Make sure you have these peer dependencies installed in your React Native project:
{
"react": ">=18.3.1",
"react-native": ">=0.76.9",
"react-native-svg": ">=15.8.0"
}
npm install react-native-svg
or
yarn add react-native-svg
or
bun add react-native-svg
or
pnpm add react-native-svg
npm install @masumdev/rn-qrcode
or
yarn add @masumdev/rn-qrcode
or
bun add @masumdev/rn-qrcode
or
pnpm add @masumdev/rn-qrcode
import { QRCode } from '@masumdev/rn-qrcode';
const GeneratorComponent = () => {
return <QRCode variant="BASIC" value="https://github.com/masumrpg" size={300} backgroundColor="white" errorCorrectionLevel="H" includeBackground />;
};
import { QRCode } from '@masumdev/rn-qrcode';
import { View } from 'react-native';
export default function App() {
// Rain Effect
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<QRCode
value="Rain Qr Code"
size={qrSize}
color="#2074a7"
version={2}
eye={{
topLeft: {
shape: 'square',
size: {
center: 1.2,
inner: 1.3,
},
radius: {
radiusOuter: 20,
radiusInner: 13,
radiusCenter: 10,
},
},
topRight: {
shape: 'square',
size: {
center: 1.2,
inner: 1.3,
},
radius: {
radiusOuter: 20,
radiusInner: 13,
radiusCenter: 10,
},
},
bottomLeft: {
shape: 'square',
size: {
center: 1.2,
inner: 1.3,
},
radius: {
radiusOuter: 20,
radiusInner: 13,
radiusCenter: 10,
},
},
}}
piece={{
shape: 'rain',
size: 1,
}}
includeBackground
/>
</View>
);
}
Core properties for customizing the QR code appearance and behavior.
Prop | Type | Default | Description |
---|---|---|---|
value | string | - | The content to be encoded in the QR code. Can be any text, URL, or data string. |
variant | QRCodeVariant | 'BASIC' | The design variant of the QR code. Choose from predefined templates. |
size | number | 256 | The size of the QR code in pixels. Determines both width and height. |
color | string | '#000' | The main color of the QR code elements. Accepts any valid CSS color value. |
backgroundColor | string | 'transparent' | The background color behind the QR code. Set to 'transparent' for no background. |
gradient | QRCodeGradientConfig | - | Advanced gradient configuration for creating beautiful color transitions. |
logo | LogoOptions | - | Options for adding a centered logo image with customizable size and styling. |
piece | PieceOptions | - | Customize the shape and style of individual QR code elements. |
eye | EyeOptions | - | Style the three corner finder patterns (eyes) of the QR code. |
includeBackground | boolean | false | When true, adds a wrapper background element behind the QR code. |
version | QRCodeVersion | - | Set a specific QR code version (1-40). Higher versions can store more data. |
maxVersion | QRCodeVersion | - | The maximum allowed QR code version. Useful for limiting complexity. |
imageClip | ImageProps | - | Props for adding an image as a clip mask to the QR code pattern, SVG Props |
errorCorrectionLevel | QRCodeErrorCorrectionLevel | 'M' | Error correction capability: L (7%), M (15%), Q (25%), or H (30%). |
Choose from a range of predefined templates to quickly style your QR code.
Value | Description | Visual Effect |
---|---|---|
'BASIC' | Simple, basic QR code | Minimalistic, clean |
'TRIANGLE' | Triangle-shaped QR code | Triangular, elegant |
'HEART' | Heart-shaped QR code | Hearty, romantic |
'DOT' | Dotted QR code pattern | Modern, minimalist |
'WITH_LOGO' | QR code with centered logo | Professional, branded |
'RAIN' | Rainy QR code with falling pieces | Rainy, atmospheric |
'LINEAR_GRADIENT' | Linear gradient colored QR code | Smooth, colorful |
'RADIAL_GRADIENT' | Radial gradient colored QR code | Dynamic, radiant |
'IMAGE_BACKGROUND' | QR code with image background | Creative, unique |
Create beautiful gradient effects for your QR code with these advanced configuration options.
Prop | Type | Default | Description |
---|---|---|---|
type | 'linear' | 'radial' | 'linear' | Choose between linear (straight line) or radial (circular) gradient patterns |
maskLogo | boolean | false | When true, prevents the gradient from overlaying the logo area |
direction | GradientDirection | 'to-right' | Sets the flow direction for linear gradients (see GradientDirection below) |
colors | GradientColor[] | - | Define multiple color stops with position and opacity for smooth transitions |
cx | string | '50%' | Center X position of radial gradient (e.g. '50%' for middle) |
cy | string | '50%' | Center Y position of radial gradient (e.g. '50%' for middle) |
r | string | '50%' | Radius of the radial gradient (e.g. '50%' for half of QR code size) |
fx | string | - | Focal point X position for radial gradient (creates elliptical effects) |
fy | string | - | Focal point Y position for radial gradient (creates elliptical effects) |
x1 | string | '0%' | Starting X position for linear gradient (e.g. '0%' for left edge) |
y1 | string | '0%' | Starting Y position for linear gradient (e.g. '0%' for top edge) |
x2 | string | '100%' | Ending X position for linear gradient (e.g. '100%' for right edge) |
y2 | string | '0%' | Ending Y position for linear gradient (e.g. '0%' for top edge) |
Specify the flow direction of linear gradients. This setting creates dynamic and visually appealing QR codes.
Value | Description | Visual Effect |
---|---|---|
'to-right' | Gradient flows from left to right | Horizontal transition β |
'to-left' | Gradient flows from right to left | Horizontal transition β |
'to-bottom' | Gradient flows from top to bottom | Vertical transition β |
'to-top' | Gradient flows from bottom to top | Vertical transition β |
'to-bottom-right' | Gradient flows diagonally from top-left to bottom-right | Diagonal transition β |
'to-bottom-left' | Gradient flows diagonally from top-right to bottom-left | Diagonal transition β |
'to-top-right' | Gradient flows diagonally from bottom-left to top-right | Diagonal transition β |
'to-top-left' | Gradient flows diagonally from bottom-right to top-left | Diagonal transition β |
Define smooth color transitions by specifying multiple color stops in your gradient. Each stop controls position, color, and transparency.
Prop | Type | Description | Example |
---|---|---|---|
offset | string | Position of the color stop along the gradient (0-100%) | '0%' for start, '50%' for middle, '100%' for end |
color | string | Any valid color value (hex, rgb, named colors) | '#FF5733', 'rgb(255,87,51)', 'coral' |
opacity | number | Transparency level between 0 (invisible) and 1 (solid) | 0.8 for 80% opacity |
Customize the appearance of a centered logo within your QR code. The logo can be an image from your local assets or a remote URL.
Prop | Type | Default | Description | Notes |
---|---|---|---|---|
source | ImageSource | - | Logo image source (local asset/URL) | See ImageSource below for format details |
size | number | 20% | Logo size as percentage of QR code width | Keep between 10-30% for best scanning |
backgroundColor | string | 'white' | Background color behind the logo | Use light colors for better contrast |
padding | number | 2 | Space around logo in pixels | Helps logo stand out from QR pattern |
borderRadius | number | 0 | Rounded corners for logo background | Higher values create circular shapes |
Specify the source of your logo image. The component supports both local project assets and remote image URLs.
Type | Description | Example | Best Practices |
---|---|---|---|
number | Local image from project assets | require('./assets/logo.png') | Use for bundled images |
string | Remote image from URL | 'https://example.com/logo.png' | Ensure reliable hosting |
Important Guidelines:
Customize the appearance of individual QR code elements (the small squares that make up the pattern).
Prop | Type | Default | Description | Visual Impact |
---|---|---|---|---|
shape | 'square'|'dot'|'triangle'|'heart'|'rain' | 'square' | Shape of each QR code element | Changes overall pattern style |
size | number | 1 | Scale factor for element size (0.5-2.0) | Affects density and readability |
spacing | number | 0 | Gap between elements in pixels | Creates breathing room in pattern |
Style the three finder patterns (eyes) in the corners of the QR code. These are crucial for scanner orientation.
Prop | Type | Default | Description | Impact |
---|---|---|---|---|
topLeft | EyeShapeConfig | {} | Style for top-left corner pattern | Affects scanning reliability |
topRight | EyeShapeConfig | {} | Style for top-right corner pattern | Must remain distinct |
bottomLeft | EyeShapeConfig | {} | Style for bottom-left corner pattern | Helps orientation |
Detailed configuration for each QR code eye (finder pattern).
Prop | Type | Default | Description | Best Practice |
---|---|---|---|---|
shape | 'square'|'circle'|'rounded' | 'square' | Overall shape of the eye pattern | Keep distinctive |
size | { center: number, inner: number } | - | Size multiplier for center and inner components | Maintain proportions |
radius | { radiusOuter: number, radiusInner: number, radiusCenter: number } | - | Corner rounding for each part | Balance style with function |
color | string | - | Main color of the eye pattern | Use high contrast |
innerColor | string | - | Color of the inner eye component | Complement main color |
Control the size and data capacity of your QR code.
Type | Description | Use Case |
---|---|---|
number (1-40) | QR code version number | Higher versions = more data, larger size |
Version Guide:
Advanced SVG image properties for creating custom QR code patterns.
Prop | Type | Default | Description | Notes |
---|---|---|---|---|
x | number | - | Horizontal position of the image | Position from left |
y | number | - | Vertical position of the image | Position from top |
width | number | - | Width of the image | In pixels or percentage |
height | number | - | Height of the image | In pixels or percentage |
xlinkHref | ImageSource | string | - | Source of the image (legacy attribute) | Use href instead |
href | ImageSource | string | - | Source of the image | Preferred over xlinkHref |
preserveAspectRatio | string | - | How image should scale (e.g., 'xMidYMid meet') | Controls image fitting |
opacity | number | 1 | Transparency of the image (0-1) | 0 = transparent, 1 = solid |
onLoad | function | - | Callback when image loads | Handles load completion |
Balance between data redundancy and error correction capability.
Level | Error Correction Capacity | Best Used For |
---|---|---|
L | ~7% damage recovery | Clean environments, maximum data capacity |
M | ~15% damage recovery | Standard use, good balance |
Q | ~25% damage recovery | Public display, some wear expected |
H | ~30% damage recovery | Outdoor use, high reliability needed |
MIT
FAQs
A powerful and easy-to-use QR code scanning and generation library for React Native applications.
The npm package @masumdev/rn-qrcode receives a total of 16 weekly downloads. As such, @masumdev/rn-qrcode popularity was classified as not popular.
We found that @masumdev/rn-qrcode demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Β It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
Research
Security News
Malicious Koishi plugin silently exfiltrates messages with hex strings to a hardcoded QQ account, exposing secrets in chatbots across platforms.
Research
Security News
Malicious PyPI checkers validate stolen emails against TikTok and Instagram APIs, enabling targeted account attacks and dark web credential sales.