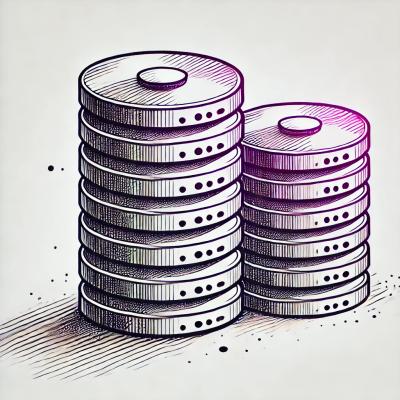
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
@maxweek/react-scroller
Advanced tools
Make your blocks scrolling easy, with a custom scroll-bar, based on native browser scroll
Make your blocks scrolling easy, with a custom scroll-bar, based on native browser scroll. It is for simple and progressive applications, works on all modern browsers. It has a minimal load on the system, and has maximum performance, expandable and updatable
npm i @maxweek/react-scroller
import Scroller from "@maxweek/react-scroller";
import "@maxweek/react-scroller/css";
const YourComponent = () => (
<Scroller>
{/* Your content */}
</Scroller>
);
import Scroller, { IScrollerRef, IScroller, IScrollerProperties } from "@maxweek/react-scroller";
import "@maxweek/react-scroller/css";
import { useRef } from "react";
const YourComponent = () => {
// Ref
const scrollerRef = useRef<IScrollerRef>(null);
// Methods
const scrollToStart = () => {
scrollerRef.current?.scrollToStart(); // scroll to start
};
const scrollToEnd = () => {
scrollerRef.current?.scrollToEnd(); // scroll to end
};
const scrollTo = () => {
scrollerRef.current?.scrollTo(100); // scroll to 100px
};
const update = () => {
scrollerRef.current?.update(); // update scroll calculations
};
const getScrollerRef = () => {
let ref = scrollerRef.current?.scrollRef; // get ref of main scroller box to control manually
};
const getProperties = () => {
if (!scrollerRef.current) return;
let properties: IScrollerProperties = scrollerRef.current?.getProperties(); // get properties of scroller object
};
// Scroller
return (
<Scroller
ref={scrollerRef}
needBar={true}
barAltPosition={false}
vertical={true}
horizontal={true}
grab={true}
borderFade={true}
autoHide={false}
borderPadding={true}
grabCursor={true}
showWhenMinimal={true}
className={'your-scroller-class'}
barClassName={'your-scroller-bar-class'}
barRollerClassName={'your-scroller-bar-roller-class'}
contentClassName={'your-scroller-content-class'}
onScroll={(x, y) => console.log(`scroll progress x: ${x.progress}, y: ${y.progress}`)}
onReachStart={(type) => console.log(`reach start ${type}`)}
onReachEnd={(type) => console.log(`reach end ${type}`)}
>
{/* Your content */}
</Scroller>
);
};
Full usage you can see on https://github.com/maxweek/react-scroller
import { IScroller } from "@maxweek/react-scroller";
import "@maxweek/react-scroller/css";
const props: Partial<IScroller> = {
needBar: true,
barAltPosition: false,
vertical: true,
horizontal: true,
grab: true,
autoHide: false,
borderFade: true,
borderPadding: true,
grabCursor: true,
showWhenMinimal: true,
className: 'your-scroller-class',
barClassName: 'your-scroller-bar-class',
barRollerClassName: 'your-scroller-bar-roller-class',
contentClassName: 'your-scroller-content-class',
onScroll: (x, y) => console.log(`scroll progress x: ${x.progress}, y: ${y.progress}`),
onReachStart: (type) => console.log(`reach start ${type}`),
onReachEnd: (type) => console.log(`reach end ${type}`)
};
vertical
: Enables vertical scrolling. Default is false
.horizontal
: Enables horizontal scrolling. Default is false
.both
: Enables both vertical and horizontal scrolling. Default is false
.const config = {
// ...existing config options...
vertical: true, // Enable vertical scrolling
horizontal: true, // Enable horizontal scrolling
both: true, // Enable both axis scrolling
};
PropName | Type | Default | Description |
---|---|---|---|
children | ReactNode | React child | |
ref? | IScrollerRef | Ref to control the element | |
needBar? | boolean | false | Enables scrollbar |
barAltPosition? | boolean | false | Changes scrollbar position, default at right - changes to left, when horizontal enabled - changes bottom to top |
vertical? | boolean | false | Enables vertical scrolling |
horizontal? | boolean | false | Enables horizontal scrolling |
grab? | boolean | false | Enables grabbing your scroll content |
borderFade? | boolean | false | Adds fading in directions of scroll by masking |
borderPadding? | boolean | false | Adds padding in directions of scroll |
autoHide? | boolean | false | Hides scrollbar if it is not hovered |
grabCursor? | boolean | false | Enables grab cursor on hover |
showWhenMinimal? | boolean | true | Enables bar on hover, when the scroll height is smaller than box height |
className? | string | '' | CSS Class for scroller box |
barClassName? | string | '' | CSS Class for scrollbar |
barRollerClassName? | string | '' | CSS Class for scrollbar roller |
contentClassName? | string | '' | CSS Class for content wrapper |
onScroll? | event | (x: IScrollerProgress, y: IScrollerProgress) => {} | Event on 'scroll', `x` and `y` props are the interpolation of scroll progress from 0 to 1 |
onReachStart? | event | (type: 'x' | 'y') => {} | Event on 'scroll' reaches start |
onReachEnd? | event | (type: 'x' | 'y') => {} | Event on 'scroll' reaches end |
import { IScrollerRef, IScroller, IScrollerProperties } from "@maxweek/react-scroller";
import { useRef } from "react";
const scrollerRef = useRef<IScrollerRef>(null);
// Methods
const scrollToStart = () => {
scrollerRef.current?.scrollToStart(2000); // scroll to start
};
const scrollToEnd = () => {
scrollerRef.current?.scrollToEnd(2000); // scroll to end
};
const scrollTo = () => {
scrollerRef.current?.scrollTo(100, 2000); // scroll to 100px
};
const update = () => {
scrollerRef.current?.update(); // update scroll calculations
};
const getScrollerRef = () => {
let ref = scrollerRef.current?.scrollRef; // get ref of main scroller box to control manually
};
const getProperties = () => {
if (!scrollerRef.current) return;
let properties: IScrollerProperties = scrollerRef.current?.getProperties(); // get properties of scroller object
};
Your issues on GitHub
GitHub: https://github.com/maxweek/react-scroller
Thank you for using my package!
Max Nedelko 2024
"touch", "scrollbar", "horizontal", "scroller", "scroll", "react"
FAQs
Make your blocks scrolling easy, with a custom scroll-bar, based on native browser scroll
We found that @maxweek/react-scroller demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.