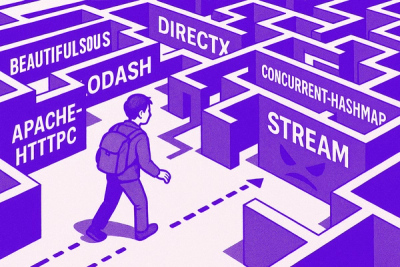
Research
NPM targeted by malware campaign mimicking familiar library names
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
@mdaemon/observable
Advanced tools
A function for creating an observable object, array, boolean, string, or number.
The observable
function provides methods for setting, getting, observing, and stopping observation of any of the following value types: object
, array
, boolean
, string
, and number
.
$ npm install @mdaemon/observable --save
const observe = require("@mdaemon/observable/dist/observable.cjs");
import observe from "@mdaemon/observable/dist/observable.mjs";
<script type="text/javascript" src="/path_to_modules/dist/observable.umd.js">
You can use observe to keep track of a value from multiple contexts
import observe from "@mdaemon/observable/dist/observable.mjs";
// observeTheseValues.js
const observedNumber = observe("numberName", 20);
export observedNumber;
// note that objects are clones, so this object will not be changed by changes to the observedObject
const obj = {};
const observedObject = observe("objectName", obj);
export observedObject;
const observedArray = observe("arrayName", []);
export observedArray;
const observedBoolean = observe("boolName", true);
export observedBoolean;
const observedString = observe("stringName","test");
export observedString;
// index.js
import {
observedNumber, observedObject,
observedArray, observedBoolean,
observedString
} from "observeTheseValues.js";
// change the value and return changed true/false
let changed = observedNumber(30);
console.log(changed); // true
console.log(observedNumber(30)); // false
// get the value
console.log(observedNumber() === 30); // true
// watch for value changes
const stopObservingValue = observedNumber((newValue, oldValue) => {
console.log("new", newValue);
console.log("old", oldValue);
console.log(newValue === oldValue);
});
// change the value for observation
observedNumber(3);
// new 3
// old 30
// false
// stop observing changes
stopObservingValue();
// change the value again
observedNumber(60); // nothing logged
// observe also finds changes that are part of objects
const stopObservingObject = observedObject((newValue, oldValue) => {
console.log("new", newValue);
});
console.log(observedObject({ test: 10 })); // true
// { test: 10 }
// to remove a property from an object, set it to undefined
observedObject({ test: undefined });
// { }
// from 2.0 you can also get an already observed value using the name of the value passed to the original
const str = observe("stringName");
console.log(str()); // "test"
You can destroy an observable instance by passing a special string to the observe function. This will remove the observable from the internal list, allowing it to be garbage collected if there are no other references to it.
// Destroy the observable instance
str("destroy-observable-stringName");
// Attempting to get the value of the destroyed observable will now return undefined
const str = observe("stringName");
console.log(str()); // undefined
Published under the LGPL-2.1 license.
Published by
MDaemon Technologies, Ltd.
Simple Secure Email
https://www.mdaemon.com
FAQs
A function for creating an observable object, array, boolean, string, or number.
The npm package @mdaemon/observable receives a total of 12 weekly downloads. As such, @mdaemon/observable popularity was classified as not popular.
We found that @mdaemon/observable demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
Research
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.