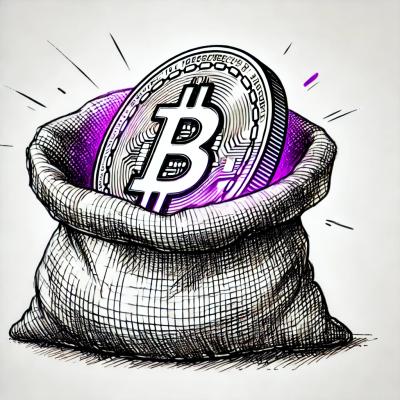
Research
Security News
Malicious npm Packages Use Telegram to Exfiltrate BullX Credentials
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
@messageformat/date-skeleton
Advanced tools
A parser & formatter for ICU DateFormat skeleton strings
@messageformat/date-skeleton is an npm package that provides utilities for formatting dates using skeletons, which are patterns that describe the desired components and their order in a date format. This package is useful for internationalization (i18n) and localization (l10n) of date formats.
Basic Date Formatting
This feature allows you to format a date using a skeleton pattern. In this example, the skeleton 'yMMMd' formats the date to 'Oct 1, 2023'.
const { DateSkeleton } = require('@messageformat/date-skeleton');
const date = new Date('2023-10-01T12:00:00Z');
const skeleton = new DateSkeleton('yMMMd');
console.log(skeleton.format(date)); // Output: Oct 1, 2023
Custom Time Formatting
This feature allows you to format the time part of a date using a skeleton pattern. In this example, the skeleton 'Hm' formats the time to '12:00'.
const { DateSkeleton } = require('@messageformat/date-skeleton');
const date = new Date('2023-10-01T12:00:00Z');
const skeleton = new DateSkeleton('Hm');
console.log(skeleton.format(date)); // Output: 12:00
Localized Date Formatting
This feature allows you to format a date using a skeleton pattern with localization. In this example, the skeleton 'yMMMd' with the locale set to 'fr' (French) formats the date to '1 oct. 2023'.
const { DateSkeleton } = require('@messageformat/date-skeleton');
const date = new Date('2023-10-01T12:00:00Z');
const skeleton = new DateSkeleton('yMMMd', { locale: 'fr' });
console.log(skeleton.format(date)); // Output: 1 oct. 2023
date-fns is a modern JavaScript date utility library that provides comprehensive, yet simple, functions for working with dates. It offers a wide range of date manipulation and formatting functions, and it is known for its modularity and performance. Compared to @messageformat/date-skeleton, date-fns provides more general-purpose date utilities but may require more configuration for internationalization.
Moment.js is a widely-used JavaScript library for parsing, validating, manipulating, and formatting dates. It provides extensive support for internationalization and localization. While Moment.js is more feature-rich and easier to use for complex date manipulations, it is also larger in size compared to @messageformat/date-skeleton, which is more focused on skeleton-based date formatting.
Luxon is a modern JavaScript library for working with dates and times, built by one of the Moment.js developers. It offers a more modern API and better support for internationalization and localization. Luxon is a good alternative to @messageformat/date-skeleton for developers looking for a more comprehensive date-time library with built-in i18n support.
Tools for working with ICU DateFormat skeletons.
import {
DateFormatError,
DateToken, // TS only
getDateFormatter,
getDateFormatterSource,
parseDateTokens
} from '@messageformat/date-skeleton';
The package is released as an ES module only. If using from a CommonJS context, you may need to import()
it, or use a module loader like esm.
Uses Intl.DateTimeFormat internally. Position-dependent ICU DateFormat patterns are not supported, as they cannot be represented with Intl.DateTimeFormat options.
Class | Description |
---|---|
DateFormatError | Parent class for errors. |
Function | Description |
---|---|
getDateFormatter(locales, tokens, onError) | Returns a date formatter function for the given locales and date skeleton |
getDateFormatter(locales, tokens, timeZone, onError) | Returns a date formatter function for the given locales and date skeleton with time zone overrride. |
getDateFormatterSource(locales, tokens, onError) | Returns a string of JavaScript source that evaluates to a date formatter function with the same (date: Date | number) => string signature as the function returned by getDateFormatter(). |
getDateFormatterSource(locales, tokens, timeZone, onError) | Returns a string of JavaScript source that evaluates to a date formatter function with the same (date: Date | number) => string signature as the function returned by getDateFormatter() with time zone override . |
parseDateTokens(src) | Parse an ICU DateFormat skeleton string into a DateToken array. |
Type Alias | Description |
---|---|
DateToken | An object representation of a parsed date skeleton token |
Messageformat is an OpenJS Foundation project, and we follow its Code of Conduct.
FAQs
A parser & formatter for ICU DateFormat skeleton strings
The npm package @messageformat/date-skeleton receives a total of 233,568 weekly downloads. As such, @messageformat/date-skeleton popularity was classified as popular.
We found that @messageformat/date-skeleton demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.
Security News
AI-generated slop reports are making bug bounty triage harder, wasting maintainer time, and straining trust in vulnerability disclosure programs.