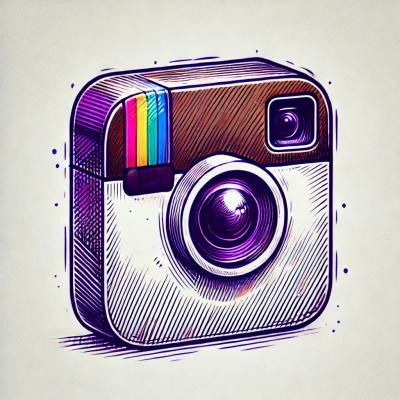
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
@microfox/rest-sdk
Advanced tools
A powerful, type-safe HTTP client for TypeScript applications with support for multiple response types, retry mechanisms, and extensive configuration options.
A powerful, type-safe HTTP client for TypeScript applications with support for multiple response types, retry mechanisms, and extensive configuration options.
npm install @microfox/rest-sdk
# or
yarn add @microfox/rest-sdk
import { createRestSDK } from '@microfox/rest-sdk';
// Create SDK instance
const api = createRestSDK({
baseUrl: 'https://api.example.com',
});
// Make requests
const data = await api.get('/users').json();
// GET request
const users = await api.get('/users').json();
// POST request with JSON body
const newUser = await api
.post('/users', {
name: 'John Doe',
email: 'john@example.com',
})
.json();
// PUT request
const updated = await api
.put('/users/1', {
name: 'Jane Doe',
})
.json();
// DELETE request
await api.delete('/users/1').json();
// JSON response
const jsonData = await api.get('/data').json();
// Text response
const textData = await api.get('/text').text();
// Binary data
const binaryData = await api.get('/file').blob();
// Form data
const formData = await api.get('/form').formData();
// Access headers
const headers = await api.get('/data').headers();
// Get raw response
const response = await api.get('/data').raw();
const response = await api
.get('/users', {
// Query parameters
query: {
page: 1,
limit: 10,
filter: ['active', 'verified'],
},
// Custom headers
headers: {
'X-Custom-Header': 'value',
},
// Content type
contentType: 'application/json',
// Retry configuration
retry: {
attempts: 3,
delay: 1000,
backoff: 2,
},
// Request configuration
cache: 'no-cache',
credentials: 'include',
mode: 'cors',
})
.json();
// Form Data
const formData = new FormData();
formData.append('file', fileBlob);
formData.append('name', 'test.txt');
const uploadResult = await api
.post('/upload', formData, {
contentType: 'multipart/form-data',
})
.json();
// URL-encoded form
const formResult = await api
.post(
'/submit',
{
key: 'value',
},
{
contentType: 'application/x-www-form-urlencoded',
},
)
.json();
// Binary data
const binaryResult = await api
.post('/binary', binaryBlob, {
contentType: 'application/octet-stream',
})
.json();
try {
const data = await api.get('/data').json();
} catch (error) {
if (APICallError.isInstance(error)) {
// Handle API errors (4xx, 5xx)
console.error('API Error:', {
message: error.message,
statusCode: error.statusCode,
url: error.url,
});
} else if (MicrofoxSDKError.isInstance(error)) {
// Handle SDK-specific errors
console.error('SDK Error:', {
name: error.name,
message: error.message,
});
} else {
// Handle other errors
console.error('Unknown error:', error);
}
}
interface User {
id: number;
name: string;
email: string;
}
interface CreateUser {
name: string;
email: string;
}
// Type-safe requests
const user = await api.get<User>('/users/1').json();
const newUser = await api
.post<User, CreateUser>('/users', {
name: 'John',
email: 'john@example.com',
})
.json();
const authenticatedApi = createRestSDK({
baseUrl: 'https://api.example.com',
headers: {
Authorization: `Bearer ${token}`,
Accept: 'application/json',
},
});
const createApiClient = (environment: 'development' | 'production') => {
const config = {
development: {
baseUrl: 'https://dev-api.example.com',
timeout: 10000,
},
production: {
baseUrl: 'https://api.example.com',
timeout: 5000,
},
};
return createRestSDK(config[environment]);
};
Thrown when the server returns a non-2xx status code.
Properties:
message
: Error messagestatusCode
: HTTP status codeurl
: Request URLresponseHeaders
: Response headersrequestBodyValues
: Request body (if any)Thrown for SDK-specific errors (timeout, invalid response type, etc.).
Properties:
name
: Error namemessage
: Error messageContributions are welcome! Please read our Contributing Guide for details on our code of conduct and the process for submitting pull requests.
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
A powerful, type-safe HTTP client for TypeScript applications with support for multiple response types, retry mechanisms, and extensive configuration options.
The npm package @microfox/rest-sdk receives a total of 80 weekly downloads. As such, @microfox/rest-sdk popularity was classified as not popular.
We found that @microfox/rest-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.