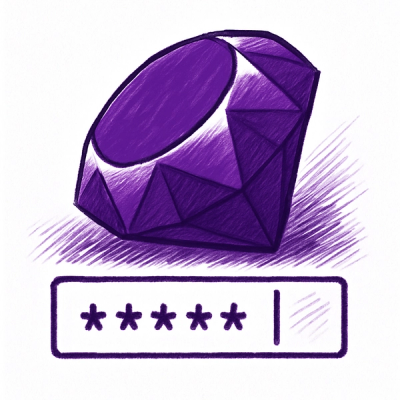
Research
/Security News
60 Malicious Ruby Gems Used in Targeted Credential Theft Campaign
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
@microsoft/recognizers-text-suite
Advanced tools
recognizers-text-suite provides robust recognition and resolution of numbers, units, date/time, and more; expressed in multiple languages.
Recognizers are organized into groups and designed to be used in C#, Node.js, Python and Java to help you build great applications! To use the samples, install the recognizers-text-suite
package, or clone our GitHub repository using Git.
git clone https://github.com/Microsoft/Recognizers-Text.git
cd Recognizers-Text
You can choose between build the solution manually or through an automatized build.cmd file.
Open a terminal and run the following commands:
cd JavaScript
npm install
npm run build
npm run test
Launch Build.cmd
file.
-- Note: the initial versions of the packages are available with the @preview tag. --
Install all the Recognizer's by launching the following command:
npm install @microsoft/recognizers-text-suite
Or, if you prefer to use a single type of recognizer:
Get only the numbers Recognizer's features:
npm install @microsoft/recognizers-text-number
Get only the numbers with units Recognizer's features:
npm install @microsoft/recognizers-text-number-with-unit
Get only the date and time Recognizer's features:
npm install @microsoft/recognizers-text-date-time
Get only the sequence Recognizer's features:
npm install @microsoft/recognizers-text-sequence
Get only the choice Recognizer's features:
npm install @microsoft/recognizers-text-choice
Once the proper package is installed, you'll need to reference the package:
var Recognizers = require('@microsoft/recognizers-text-suite');
var NumberRecognizers = require('@microsoft/recognizers-text-number');
var NumberWithUnitRecognizers = require('@microsoft/recognizers-text-number-with-unit');
var DateTimeRecognizers = require('@microsoft/recognizers-text-date-time');
var SequenceRecognizers = require('@microsoft/recognizers-text-sequence');
var ChoiceRecognizers = require('@microsoft/recognizers-text-choice');
This is the preferred way if you need to parse multiple inputs based on the same context (e.g.: language and options):
var recognizer = new NumberRecognizers.NumberRecognizer(Recognizers.Culture.English);
var model = recognizer.getNumberModel();
var result = model.parse('Twelve');
Or, for less verbosity, you use the helper methods:
var result = Recognizers.recognizeNumber("Twelve", Recognizers.Culture.English);
Internally, both methods will cache the instance models to avoid extra costs.
Numbers
This recognizer will find any number from the input. E.g. "I have two apples" will return "2".
Recognizers.recognizeNumber('I have two apples', Recognizers.Culture.English)
Or you can obtain a model instance using:
new NumberRecognizers.NumberRecognizer(Recognizers.Culture.English).getNumberModel()
Ordinal Numbers
This recognizer will find any ordinal number. E.g. "eleventh" will return "11".
Recognizers.recognizeOrdinal('eleventh', Recognizers.Culture.English)
Or you can obtain a model instance using:
new NumberRecognizers.NumberRecognizer(Recognizers.Culture.English).getOrdinalModel()
Percentages
This recognizer will find any number presented as percentage. E.g. "one hundred percents" will return "100%".
Recognizers.recognizePercentage('one hundred percents', Recognizers.Culture.English)
Or you can obtain a model instance using:
new NumberRecognizers.NumberRecognizer(Recognizers.Culture.English).getPercentageModel()
Ages
This recognizer will find any age number presented. E.g. "After ninety five years of age, perspectives change" will return "95 Year".
Recognizers.recognizeAge('After ninety five years of age, perspectives change', Recognizers.Culture.English)
Or you can obtain a model instance using:
new NumberWithUnitRecognizers.NumberWithUnitRecognizer(Recognizers.Culture.English).getAgeModel()
Currencies
This recognizer will find any currency presented. E.g. "Interest expense in the 1988 third quarter was $ 75.3 million" will return "75300000 Dollar".
Recognizers.recognizeCurrency('Interest expense in the 1988 third quarter was $ 75.3 million', Recognizers.Culture.English)
Or you can obtain a model instance using:
new NumberWithUnitRecognizers.NumberWithUnitRecognizer(Recognizers.Culture.English).getCurrencyModel()
Dimensions
This recognizer will find any dimension presented. E.g. "The six-mile trip to my airport hotel that had taken 20 minutes earlier in the day took more than three hours." will return "6 Mile".
Recognizers.recognizeDimension('The six-mile trip to my airport hotel that had taken 20 minutes earlier in the day took more than three hours.', Recognizers.Culture.English)
Or you can obtain a model instance using:
new NumberWithUnitRecognizers.NumberWithUnitRecognizer(Recognizers.Culture.English).getDimensionModel()
Temperatures
This recognizer will find any temperature presented. E.g. "Set the temperature to 30 degrees celsius" will return "30 C".
Recognizers.recognizeTemperature('Set the temperature to 30 degrees celsius', Recognizers.Culture.English)
Or you can obtain a model instance using:
new NumberWithUnitRecognizers.NumberWithUnitRecognizer(Recognizers.Culture.English).getTemperatureModel()
DateTime
This recognizer will find any date, time, duration and date/time ranges, even if its write in colloquial language. E.g. "I'll go back 8pm today" will return "2017-10-04 20:00:00".
Recognizers.recognizeDateTime("I'll go back 8pm today", Recognizers.Culture.English)
Or you can obtain a model instance using:
new DateTimeRecognizers.DateTimeRecognizer(Recognizers.Culture.English).getDateTimeModel()
Phone Numbers
This model will find any patter of symbols detected as a phone number, even if its write in coloquial language. E.g. "My phone number is 1 (877) 609-2233." will return "1 (877) 609-2233".
Recognizers.recognizePhoneNumber("My phone number is 1 (877) 609-2233.", Culture.English)
Or you can obtain a model instance using:
new SequenceRecognizers.SequenceRecognizer(Culture.English).GetPhoneNumberModel()
IP Address
This model will find any Ipv4/Ipv6 presented. E.g. "My Ip is 8.8.8.8".
Recognizers.recognizeIpAddress("My Ip is 8.8.8.8")
Or you can obtain a model instance using:
new SequenceRecognizers.SequenceRecognizer(Culture.English).IpAddressModel()
Booleans
This recognizer will find any boolean value, even if its write with emoji. E.g. "π It's ok" will return "true".
Recognizers.recognizeBoolean("π It's ok", Recognizers.Culture.English)
Or you can obtain a model instance using:
new ChoiceRecognizers.ChoiceRecognizer(Recognizers.Culture.English).getBooleanModel()
The recognizers were designed to disjoint language's logic from the recognizer's core in order to grow without the obligation of change the supported platforms.
To achieve this, the recognizers contains the following folders:
FAQs
recognizers-text-suite provides robust recognition and resolution of numbers, units, date/time, and more; expressed in multiple languages.
The npm package @microsoft/recognizers-text-suite receives a total of 45,821 weekly downloads. As such, @microsoft/recognizers-text-suite popularity was classified as popular.
We found that @microsoft/recognizers-text-suite demonstrated a not healthy version release cadence and project activity because the last version was released a year ago.Β It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isnβt whitelisted.