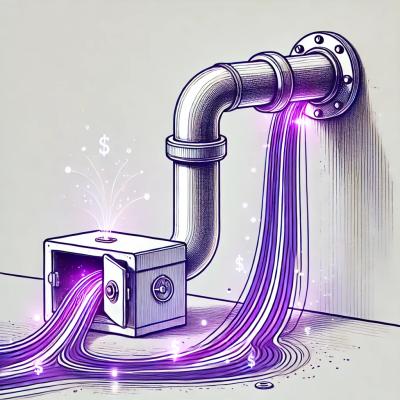
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@microsoft/vscode-azureresources-api
Advanced tools
Type declarations and client library for the Azure Resources extension API
Provides typings and utilities for the VS Code Azure Resources API. This API is used to integrate "client" Azure extensions into views provided by the Azure Resources "host" extension for VS Code.
The Azure Resources extension for VS Code contributes the Azure resources view and the Workspace resources view. The Azure view lets users browse and work with supported resources in Azure. The Workspace resources view is home to development related resources like an emulated database, or a functions project detected in an open workspace folder.
In the past, each supported Azure resource had a dedicated view in the Azure view container. In the updated design, the Azure resources and Workspace resources views contain all resources. To facilitate this design, each Azure product extension contributes to a single host extension.
The Azure Resources extension provides small generic features for all Azure resource types. Users can then install Azure product extensions to enable rich, product-specific features.
The Azure Resources extension exposes an API which enables any number of client extensions to contribute UI and functionality to the Azure and Workspace resources views.
The Azure Resources extension contributes two extendable views. The Resources view, and the Workspace view. In the Resources view, clients can customize resource tree items, and have full control over resource tree item children.
For the Workspace view, clients can contribute root level tree items to the view. Clients have full control over these tree items and their children.
The VS Code API provides a TreeDataProvider
interface for controlling tree views. In order to make the Azure trees extensible, the host extension splits tree views into "branches", where each branch is controlled by a branch data provider. Clients can register a BranchDataProvider
for a resource type. The registered branch data provider is then responsible for providing the tree items for that branch in the tree view. BranchDataProvider
is an extension of VS Code's TreeDataProvider
.
Note: clients registering resource providers must declare that they do so in their extension manifest. See Extension Manifest
/**
* The base interface for visualizers of Azure and workspace resources.
*/
export interface BranchDataProvider<TResource extends ResourceBase, TModel extends ResourceModelBase> extends vscode.TreeDataProvider<TModel> {
/**
* Get the children of `element`.
*
* @param element The element from which the provider gets children. Unlike a traditional tree data provider, this will never be `undefined`.
*
* @return Children of `element`.
*/
getChildren(element: TModel): vscode.ProviderResult<TModel[]>;
/**
* Called to get the provider's model element for a specific resource.
*
* @remarks getChildren() assumes that the provider passes a known (TModel) model item, or undefined when getting the "root" children.
* However, branch data providers have no "root" so this function is called for each matching resource to obtain a starting branch item.
*
* @returns The provider's model element for `resource`.
*/
getResourceItem(element: TResource): TModel | Thenable<TModel>;
}
Clients register resource providers to add resources to a view. Currently the API is limited to registering Workspace resource providers because a default Azure resource provider is built into the Azure Resources extension.
Note: clients registering resource providers must declare that they do so in their extension manifest. See Extension Manifest
/**
* The base interface for providers of Azure and workspace resources.
*/
export interface ResourceProvider<TResourceSource, TResource extends ResourceBase> {
/**
* Fired when the provider's resources have changed.
*/
readonly onDidChangeResource?: vscode.Event<TResource | undefined>;
/**
* Called to supply the resources used as the basis for the resource views.
*
* @param source The source from which resources should be generated.
*
* @returns The resources to be displayed in the resource view.
*/
getResources(source: TResourceSource): vscode.ProviderResult<TResource[]>;
}
Client extensions define an x-azResources
object on inside of contributes
in the extension manifest package.json
file.
This object informs the host extension when to activate the client extension and what contributions the client extension makes to the shared views.
azure?: {
/**
* List of Azure resource types this extension registers a BranchDataProvider for.
*/
branches: {
/**
* The resource type the BranchDataProvider is registered for.
*/
type: AzExtResourceType
}[];
};
/**
* List of Workspace resource types this extension registers a BranchDataProvider for.
*/
workspace?: {
branches: {
/**
* The resource type the BranchDataProvider is registered for.
*/
type: string;
}[];
/**
* Whether this extension registers a WorkspaceResourceProvider.
*/
resources?: boolean;
};
/**
* Commands to add to the "Create Resource..." quick pick prompt.
*/
commands?: {
command: string;
title: string;
detail: string;
type?: string; // Optional: resource type associated with the command. Used to show an icon next to the command.
}[];
The contribution object from the Azure Functions extension is shown below as an example.
This extension declares that it registers a BranchDataProvider for the FunctionApp
resource type in the Azure resources view, and a BranchDataProvider for the func
resource type in the Workspace resources view. It also registers a WorkspaceResourceProvider. Finally, it contributes a command to the "Create Resource..." quick pick prompt for creating a Function App.
{
"contributes": {
"x-azResources": {
"azure": {
"branches": [
// extension registers a BranchDataProvider for Function Apps
{
"type": "FunctionApp"
}
]
},
"workspace": {
"branches": [
// extension registers a BranchDataProvider for workspace resources of type "func"
{
"type": "func"
}
],
// extension registers a WorkspaceResourceProvider
"resources": true
},
"commands": [
// extension contributes a command to the "Create Resource..." quick pick prompt
{
"command": "azureFunctions.createFunctionApp",
"title": "%azureFunctions.createFunctionApp%",
"detail": "%azureFunctions.createFunctionAppDetail%"
}
],
},
// other extension contributions
}
}
Example without comments (good for copying 😄)
"x-azResources": { "azure": { "branches": [ { "type": "FunctionApp" } ] }, "workspace": { "branches": [ { "type": "func" } ], "resources": true }, "commands": [ { "command": "azureFunctions.createFunctionApp", "title": "%azureFunctions.createFunctionApp%", "detail": "%azureFunctions.createFunctionAppDetail%" } ] }
On activation, client extensions can fetch an instance of the Azure Resources API using the getAzureResourcesExtensionApi
utility provided by the @microsoft/vscode-azureresources-api
package.
import { getAzureResourcesExtensionApi } from '@microsoft/vscode-azureresources-api';
export async function activate(context: vscode.ExtensionContext): Promise<void> {
const azureResourcesApi = await getAzureResourcesExtensionApi(context, '2.0.0');
// ...register providers
}
view/title
commandsClient extensions are encouraged to make commands accessible via the workspace view/title
menu. Commands should be grouped by extension into submenus. Submenu icons and titles should be the same as the client extension's icon and title. This is to prevent the view/title
menu from becoming too cluttered.
Here's an example of how the Azure Functions extension contributes commands to the workspace view/title
menu. See the Azure Functions package.json for reference.
"submenus": [
// define the submenu
{
"id": "azureFunctions.submenus.workspaceActions",
"icon": "resources/azure-functions.png",
"label": "Azure Functions"
}
],
"menus": {
// add commands to the submenu
"azureFunctions.submenus.workspaceActions": [
{
"command": "azureFunctions.createFunction",
"group": "1_projects@1"
},
{
"command": "azureFunctions.createNewProject",
"group": "1_projects@2"
},
{
"command": "azureFunctions.deploy",
"group": "2_deploy@1"
},
{
"command": "azureFunctions.createFunctionApp",
"group": "3_create@1"
},
{
"command": "azureFunctions.createFunctionAppAdvanced",
"group": "3_create@2"
}
],
// add the submenu to the workspace view/title menu
"view/title": [
{
"submenu": "azureFunctions.submenus.workspaceActions",
"when": "view == azureWorkspace",
"group": "navigation@1"
}
],
}
Client extensions must declare the Azure Resources extension as an extension dependency in their extension manifest package.json
file.
"extensionDependencies": [
"ms-azuretools.vscode-azureresources"
]
The following extensions are integrated with the Azure Resources API v2. They are great examples of how to use the API.
See vscode-azureresources-api.d.ts for the full API definition.
FAQs
Type declarations and client library for the Azure Resources extension API
The npm package @microsoft/vscode-azureresources-api receives a total of 2,002 weekly downloads. As such, @microsoft/vscode-azureresources-api popularity was classified as popular.
We found that @microsoft/vscode-azureresources-api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.