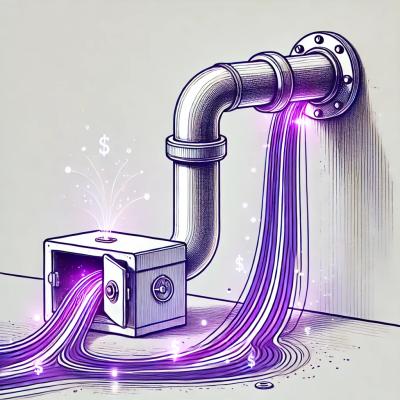
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@ministryofjustice/express-pug-pdf
Advanced tools
Generates PDF from PUG templates in Express routes
Serve PDF documents in express generated from a Pug template
npm install @ministryofjustice/express-pug-pdf --save
Specify the location of your views directory
const pugPdf = require('@ministryofjustice/express-pug-pdf')
app.use(pugPdf({ views: path.join(__dirname, 'views') }))
Render a PDF from a pug template by specifying a template name and passing the data. In this example, the template views/helloWorld.pug will be used
app.use('/pdf', (req, res) => {
res.pugpdf('helloWorld', { message: 'Hello World!' });
})
Using pug templates from the views directory
html
body
h1= message
To set the filename for the PDF when downloaded, pass the options object. The default filename is document.pdf
app.use('/pdf', (req, res) => {
res.pugpdf('helloWorld', { message: 'Hello World!' }, { filename: 'helloWorld.pdf' });
})
To customise the PDF document, pass additional pdfOptions. The PDF creation uses https://www.npmjs.com/package/html-pdf. pdfOptions are passed through to html-pdf
const options = {
filename: 'helloWorld.pdf',
pdfOptions: {
format: 'A4',
border: {
top: '40px',
bottom: '20px',
left: '40px',
right: '20px',
},
},
}
app.use('/pdf', (req, res) => {
res.pugpdf('helloWorld', { message: 'Hello World!' }, options);
})
express-pug-pdf uses Pug to render the Pug template into HTML. Then it passes the HTML to html-pdf to generate the PDF. The PDF is returned in the response as binary data with content type application/pdf
html-pdf uses PhantomJS to generate the PDF. PhantomJS needs to be able to see any stylesheets linked in your template. This means using an absolute url
doctype html
html(lang="en")
head
link(href= "http://domain/path/styles.css", media="print", rel="stylesheet", type="text/css")
body
block content
div
h1.myStyle
A styled Heading
Or set the host (and port in dev environments) dynamically from environment config
link(href= "#{domain}/path/styles.css", media="print", rel="stylesheet", type="text/css")
Note that PhantomJS will use "print" media CSS rules when rendering PDF. Instead of @page CSS rules, use the PhantomJS paperSize options to control margins - http://phantomjs.org/api/webpage/property/paper-size.html
run node examples/helloWorld/index.js
then browse to http://localhost:3001/pdf
FAQs
Generates PDF from PUG templates in Express routes
We found that @ministryofjustice/express-pug-pdf demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.