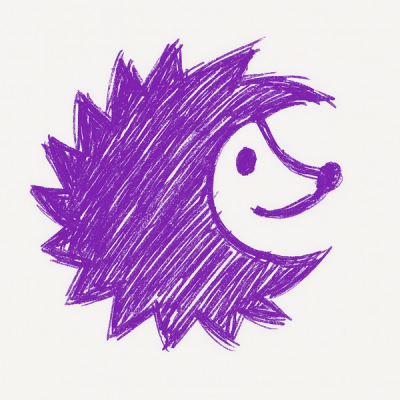
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
@momsfriendlydevco/angular-ui-query-builder
Advanced tools
MongoDB format query-builder UI component for Angular
MongoDB format query-builder UI component for Angular.
This component comes in two parts:
Demo.
npm install --save @momsfriendlydevco/angular-ui-query-builder
<script src="/libs/angular-ui-query-builder/dist/angular-ui-query-builder.min.js"/>
<link href="/libs/angular-ui-query-builder/dist/angular-ui-query-builder.min.css" rel="stylesheet" type="text/css"/>
angular.module()
call:var app = angular.module('app', ['angular-ui-query-builder'])
<ui-query-builder query="$ctrl.myQuery" spec="$ctrl.mySpec"></ui-query-builder>
A demo is also available. To use this follow the instructions in the demo directory.
Simply create a query object and link it up to the directive.
In a controller:
$scope.mySpec = {
_id: {type: 'objectId'},
name: {type: 'string'},
email: {type: 'string'},
status: {type: 'string', enum: ['pending', 'active', 'deleted']},
};
$scope.myQuery = {
status: 'active', // Assumes you have a status field
limit: 10,
skip: 0,
};
In a HTML template:
<ui-query-builder query="$ctrl.myQuery" spec="$ctrl.mySpec"></ui-query-builder>
... or see the Demo.
The ui-query-builder directive takes the following parameters:
Parameter | Type | Description |
---|---|---|
query | Object | The current query, this object will be mutated into / from a MongoDB compatible query |
spec | Object | A base specification of field types to use when providing the UI |
If using either the full JS release (angular-ui-query-builder.js
) or the table add-on (angular-ui-query-builder-tables.js
) additional functionality is provided for Tables including column setup, pagination and other functionality.
To use:
qb-table
directive to the table header with a pointer to the query object to mutateqb-col
directive to any table column to extend, include attributes like sortable
to add that functionalityqb-pagination
directive into the table footer to add pagination functionalityFor example:
<table class="table table-bordered table-striped table-hover" qb-table="query">
<thead>
<tr>
<th qb-col="name" sortable>Name</th>
<th qb-col="username" sortable>Username</th>
<th qb-col="email" sortable>Email</th>
</tr>
</thead>
<tbody>
<tr ng-repeat="row in data track by row.id">
<td>{{row.name}}</td>
<td>{{row.username}}</td>
<td>{{row.email}}</td>
</tr>
</tbody>
<tfoot>
<tr>
<td colspan="3">
<qb-pagination></qb-pagination>
</td>
</tr>
</tfoot>
</table>
For a more complex example see the demo.
Use on a <table/>
element to designate that it should be managed by this module.
Valid attributes are:
Attribute | Type | Description |
---|---|---|
qb-table | Object | The main query object to mutate when the table is interacted with |
sticky-thead | boolean | Indicates that the <thead/> portion of the table should remain on screen when scrolling |
sticky-tfoot | boolean | Indicates that the <tfoot/> portion of the table should remain on screen when scrolling |
Use on <td>
/ <th>
element within a <thead>
to provide column level interaction.
<table class="table table-bordered table-striped table-hover" qb-table="query">
<thead>
<tr>
<th qb-col="name" sortable>Name</th>
<th qb-col="username" sortable>Username</th>
<th qb-col="email" sortable>Email</th>
</tr>
</thead>
...
</table>
Valid attributes are:
Attribute | Type | Description |
---|---|---|
qb-col | string | The database field to link against in dotted notation |
sortable | boolean or string | Indicates that this column can be sorted, if blank or boolean true this simply uses the qb-col value as the field to sort by, if its a string it uses that field instead |
Use on <td>
/ <th>
element within a <thead>
/ <thead>
to provide column level interaction.
If this element is within <thead>
it adds table level meta functionality (such as controlling which rows are selected if selector
is truthy). If its within a <tbody>
it adds per-row functionality.
<table class="table table-bordered table-striped table-hover" qb-table="query">
<thead>
...
</thead>
<tbody>
<tr ng-repeat="row in data track by row.id">
<td qb-cell selector="row.selected"></td>
<td>{{row.name}}</td>
</tr>
</tbody>
</table>
Valid attributes are:
Attribute | Type | Description |
---|---|---|
selector | Object | Indicates the field to bind against + mutate when selection is toggled. This usually resembles something like row.selected |
onSelect | function | Function called with ({value}) when the selection value changes |
An element, usually found in the <tfoot>
section of a table which provides general pagination functionality.
<table class="table table-bordered table-striped table-hover" qb-table="query">
<thead>
...
</thead>
<tbody>
...
</tbody>
<tfoot>
<tr>
<td colspan="10">
<qb-pagination></qb-pagination>
</td>
</tr>
</tfoot>
</table>
This directive has no attributes.
NOTE: This is an optionally transcludable element. Any content within its tags will be inserted in the center of the pagination controls.
Simple shared resource that can be used to set qbTable settings globally in an App.
// Add a custom question into the exporter
app.config(qbTableSettingsProvider => {
qbTableSettingsProvider.export.questions.push({
id: 'docTitle',
type: 'text',
title: 'Document title',
default: 'Exported Data',
help: 'What your document will be called when exported',
});
});
Valid properties are:
Attribute | Type | Description |
---|---|---|
icons | object | Generic icon choices in your application. See the individual contents of this object for more details |
export | object | Options relevent to the qbExport directive |
export.formats | array | Collection of suported output formats. Each entity should have an id + title property |
export.questions | array | Additional questions to ask when exporting. Each question will be apptended onto the query posted to the server. Questions should have id , type and title properties with optional default and help fields |
NOTE: Since this is an Angular Provider this service is exposed as qbTableSettingsProvider
during the config stage and qbTableSettings
if you require it in a controller / component.
$or
/ $and
$eq
condition) to a multiple choice enum ($in
) when a comma is used in a string$in
type automatically$regexp
$length
FAQs
MongoDB format query-builder UI component for Angular
The npm package @momsfriendlydevco/angular-ui-query-builder receives a total of 30 weekly downloads. As such, @momsfriendlydevco/angular-ui-query-builder popularity was classified as not popular.
We found that @momsfriendlydevco/angular-ui-query-builder demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.