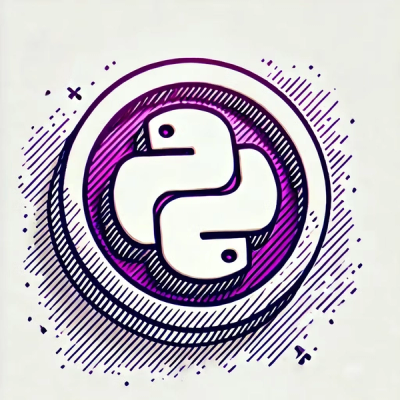
Product
Socket Now Supports pylock.toml Files
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
@n1ru4l/graphql-live-query
Advanced tools
[](https://www.npmjs.com/package/@n1ru4l/graphql-live-query) [](https://www.npmjs.com/package/@n1ru4l/
@n1ru4l/graphql-live-query is a package that enables live queries in GraphQL. It allows clients to receive real-time updates when the result of a query changes, providing a more dynamic and interactive experience.
Live Queries
This feature allows you to set up live queries in your GraphQL server. The code sample demonstrates how to integrate live queries using the @n1ru4l/graphql-live-query package.
const { execute, subscribe } = require('@n1ru4l/graphql-live-query');
const { makeExecutableSchema } = require('@graphql-tools/schema');
const { useLiveQuery } = require('@n1ru4l/graphql-live-query');
const typeDefs = `
type Query {
hello: String
}
`;
const resolvers = {
Query: {
hello: () => 'Hello world!',
},
};
const schema = makeExecutableSchema({ typeDefs, resolvers });
const liveQueryStore = new LiveQueryStore();
const executeWithLiveQueries = useLiveQuery({
execute,
subscribe,
liveQueryStore,
});
const result = await executeWithLiveQueries({
schema,
document: parse('{ hello }'),
contextValue: {},
variableValues: {},
operationName: null,
});
console.log(result);
Live Query Store
The Live Query Store is used to manage and store live queries. The code sample shows how to create a Live Query Store, add a live query to it, and notify subscribers of changes.
const { LiveQueryStore } = require('@n1ru4l/graphql-live-query');
const liveQueryStore = new LiveQueryStore();
// Add a live query to the store
const queryId = liveQueryStore.add({
document: parse('{ hello }'),
variables: {},
contextValue: {},
rootValue: {},
operationName: null,
});
// Notify subscribers of changes
liveQueryStore.invalidate(queryId);
graphql-subscriptions is a package that provides a way to implement GraphQL subscriptions using PubSub. It allows clients to subscribe to specific events and receive real-time updates. Unlike @n1ru4l/graphql-live-query, which focuses on live queries, graphql-subscriptions is more event-driven and requires explicit subscription to events.
apollo-server is a popular GraphQL server implementation that supports subscriptions out of the box. It uses WebSocket for real-time communication and can be integrated with various PubSub mechanisms. While it provides a comprehensive solution for GraphQL servers, including subscriptions, it does not specifically focus on live queries like @n1ru4l/graphql-live-query.
Primitives for adding GraphQL live query operation support to any GraphQL server.
For a usage of those utility functions check out InMemoryLiveQueryStore
(https://github.com/n1ru4l/graphql-live-queries/tree/main/packages/in-memory-live-query-store/src/InMemoryLiveQueryStore.ts).
yarn add -E @n1ru4l/graphql-live-query
GraphQLLiveDirective
Add the @live
directive to your schema.
import { GraphQLSchema, specifiedDirectives } from "graphql";
import { GraphQLLiveDirective } from "@n1ru4l/graphql-live-query";
import { query, mutation, subscription } from "./schema";
const schema = new GraphQLSchema({
query,
mutation,
subscription,
directives: [
GraphQLLiveDirective,
/* Keep @defer/@stream/@if/@skip */ ...specifiedDirectives,
],
});
Note: If you are using a SDL first approach for defining your schema (such as advocated by makeExecutableSchema
) you must add the directly to your type-definitions. In order to be as up to date as possible we recommend using graphql-tools/utils
astFromDirective
together with print
exported from graphql
for generating the SDL from GraphQLLiveDirective
.
Example (on CodeSandbox ):
import { makeExecutableSchema } from "@graphql-tools/schema";
import { astFromDirective } from "@graphql-tools/utils";
import { GraphQLLiveDirective } from "@n1ru4l/graphql-live-query";
import { print, GraphQLSchema } from "graphql";
const typeDefinitions = /* GraphQL */ `
type Query {
ping: Boolean
}
`;
const resolvers = {
Query: {
ping: () => true
}
};
const liveDirectiveTypeDefs = print(
astFromDirective(GraphQLLiveDirective)
);
export const schema = makeExecutableSchema({
typeDefs: [typeDefinitions, liveDirectiveTypeDefs],
resolvers
});
isLiveQueryOperationDefinitionNode
Determine whether a DefinitionNode
is a LiveQueryOperationDefinitionNode
.
import { parse, getOperationAST } from "graphql";
import { isLiveQueryOperationDefinitionNode } from "@n1ru4l/graphql-live-query";
const liveQueryOperationDefinitionNode = getOperationAST(
parse(/* GraphQL */ `
query @live {
me {
id
login
}
}
`)
);
isLiveQueryOperationDefinitionNode(liveQueryOperationDefinitionNode); // true
const queryOperationDefinitionNode = getOperationAST(
parse(/* GraphQL */ `
query {
me {
id
login
}
}
`)
);
isLiveQueryOperationDefinitionNode(queryOperationDefinitionNode); // false
const conditionalLiveQueryDefinitionNode = getOperationAST(
parse(/* GraphQL */ `
query($isClient: Boolean = false) @live(if: $isClient) {
me {
id
login
}
}
`)
);
isLiveQueryOperationDefinitionNode(conditionalLiveQueryDefinitionNode); // false
isLiveQueryOperationDefinitionNode(
conditionalLiveQueryDefinitionNode,
/* variables */ {
isClient: false,
}
); // false
isLiveQueryOperationDefinitionNode(
conditionalLiveQueryDefinitionNode,
/* variables */ {
isClient: true,
}
); // true
NoLiveMixedWithDeferStreamRule
Validation rule for raising a GraphQLError for a operation that use @live
mixed with @defer
and @stream
.
import { parse, validate, specifiedRules } from "graphql";
import { NoLiveMixedWithDeferStreamRule } from "@n1ru4l/graphql-live-query";
import schema from "./schema";
const document = parse(/* GraphQL */ `
query @live {
users @stream {
id
login
}
}
`);
const [error] = validate(schema, document, [
/* default validation rules */ ...specifiedRules,
NoLiveMixedWithDeferStreamRule,
]);
console.log(error); // [GraphQLError: Cannot mix "@stream" with "@live".]
FAQs
[](https://www.npmjs.com/package/@n1ru4l/graphql-live-query) [](https://www.npmjs.com/package/@n1ru4l/
The npm package @n1ru4l/graphql-live-query receives a total of 139,062 weekly downloads. As such, @n1ru4l/graphql-live-query popularity was classified as popular.
We found that @n1ru4l/graphql-live-query demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.