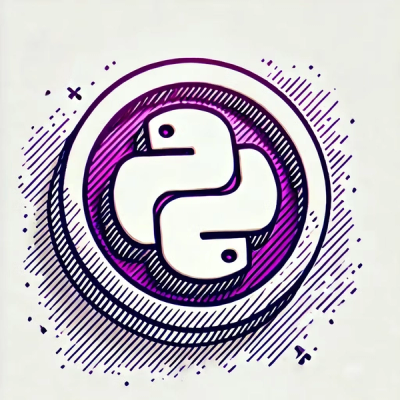
Product
Socket Now Supports pylock.toml Files
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
@nandorojo/react-native-paper-dates
Advanced tools
Performant Date Picker for React Native Paper
View video in better frame on YouTube
Web demo: reactnativepaperdates.com
We want developers to be able to build software faster using modern tools like GraphQL, Golang and React Native.
Give us a follow on Twitter: RichardLindhout, web_ridge
Instead of spending all your money on a M1 Mac, give it to us so we can buy one.
Yarn
yarn add react-native-paper-dates
npm
npm install react-native-paper-dates --save
import * as React from 'react'
import { Button } from 'react-native-paper'
import { DatePickerModal } from 'react-native-paper-dates'
function SingleDatePage() {
const [visible, setVisible] = React.useState(false)
const onDismiss = React.useCallback(() => {
setVisible(false)
}, [setVisible])
const onChange = React.useCallback(({ date }) => {
setVisible(false)
console.log({ date })
}, [])
const date = new Date()
return (
<>
<DatePickerModal
mode="single"
visible={visible}
onDismiss={onDismiss}
date={date}
onConfirm={onChange}
saveLabel="Save" // optional
label="Select date" // optional
animationType="slide" // optional, default is 'slide' on ios/android and 'none' on web
locale={'en'} // optional, default is automically detected by your system
/>
<Button onPress={()=> setVisible(true)}>
Pick date
</Button>
</>
)
}
import * as React from 'react'
import { Button } from 'react-native-paper'
import { DatePickerModal } from 'react-native-paper-dates'
export default function RangeDatePage() {
const [visible, setVisible] = React.useState(false)
const onDismiss = React.useCallback(() => {
setVisible(false)
}, [setVisible])
const onChange = React.useCallback(({ startDate, endDate }) => {
setVisible(false)
console.log({ startDate, endDate })
}, [])
return (
<>
<DatePickerModal
mode="range"
visible={visible}
onDismiss={onDismiss}
startDate={undefined}
endDate={undefined}
onConfirm={onChange}
saveLabel="Save" // optional
label="Select period" // optional
startLabel="From" // optional
endLabel="To" // optional
animationType="slide" // optional, default is slide on ios/android and none on web
locale={'en'} // optional, default is automically detected by your system
/>
<Button onPress={()=> setVisible(true)}>
Pick range
</Button>
</>
)
}
import * as React from 'react'
import { Button } from 'react-native-paper'
import { TimePickerModal } from 'react-native-paper-dates'
export default function TimePickerPage() {
const [visible, setVisible] = React.useState(false)
const onDismiss = React.useCallback(() => {
setVisible(false)
}, [setVisible])
const onConfirm = React.useCallback(
({ hours, minutes }) => {
setVisible(false);
console.log({ hours, minutes });
},
[setVisible]
);
return (
<>
<TimePickerModal
visible={visible}
onDismiss={onDismiss}
onConfirm={onConfirm}
hours={12} // default: current hours
minutes={14} // default: current minutes
label="Select time" // optional, default 'Select time'
cancelLabel="Cancel" // optional, default: 'Cancel'
confirmLabel="Ok" // optional, default: 'Ok'
animationType="fade" // optional, default is 'none'
locale={'en'} // optional, default is automically detected by your system
/>
<Button onPress={()=> setVisible(true)}>
Pick time
</Button>
</>
)
}
Things on our roadmap have labels with enhancement
.
https://github.com/web-ridge/react-native-paper-dates/issues
keyboardDismissMode="on-drag"
keyboardShouldPersistTaps="handled"
contentInsetAdjustmentBehavior="always"
This is to prevent the need to press 2 times before save or close button in modal works (1 press for closing keyboard, 1 press for confirm/close) React Native Issue: #10138
You will need to add a polyfill for the Intl API on Android if:
Install polyfills with Yarn
yarn add react-native-localize @formatjs/intl-pluralrules @formatjs/intl-getcanonicallocales @formatjs/intl-listformat @formatjs/intl-displaynames @formatjs/intl-locale @formatjs/intl-datetimeformat @formatjs/intl-numberformat @formatjs/intl-relativetimeformat
or npm
npm install react-native-localize @formatjs/intl-pluralrules @formatjs/intl-getcanonicallocales @formatjs/intl-listformat @formatjs/intl-displaynames @formatjs/intl-locale @formatjs/intl-datetimeformat @formatjs/intl-numberformat @formatjs/intl-relativetimeformat --save
In your app starting entrypoint e.g. ./index.js
or even better use a index.android.js
to prevent importing on iOS/web put the following code. (don't forget to import the languages you want to support, in the example only english language is supported)
// on top of your index.android.js file
const isAndroid = require('react-native').Platform.OS === 'android';
const isHermesEnabled = !!global.HermesInternal;
// in your index.js file
if (isHermesEnabled || isAndroid) {
require('@formatjs/intl-getcanonicallocales/polyfill');
require('@formatjs/intl-locale/polyfill');
require('@formatjs/intl-pluralrules/polyfill');
require('@formatjs/intl-pluralrules/locale-data/en.js'); // USE YOUR OWN LANGUAGE OR MULTIPLE IMPORTS YOU WANT TO SUPPORT
require('@formatjs/intl-displaynames/polyfill');
require('@formatjs/intl-displaynames/locale-data/en.js'); // USE YOUR OWN LANGUAGE OR MULTIPLE IMPORTS YOU WANT TO SUPPORT
require('@formatjs/intl-listformat/polyfill');
require('@formatjs/intl-listformat/locale-data/en.js'); // USE YOUR OWN LANGUAGE OR MULTIPLE IMPORTS YOU WANT TO SUPPORT
require('@formatjs/intl-numberformat/polyfill');
require('@formatjs/intl-numberformat/locale-data/en.js'); // USE YOUR OWN LANGUAGE OR MULTIPLE IMPORTS YOU WANT TO SUPPORT
require('@formatjs/intl-relativetimeformat/polyfill');
require('@formatjs/intl-relativetimeformat/locale-data/en.js'); // USE YOUR OWN LANGUAGE OR MULTIPLE IMPORTS YOU WANT TO SUPPORT
require('@formatjs/intl-datetimeformat/polyfill');
require('@formatjs/intl-datetimeformat/locale-data/en.js'); // USE YOUR OWN LANGUAGE OR MULTIPLE IMPORTS YOU WANT TO SUPPORT
require('@formatjs/intl-datetimeformat/add-golden-tz.js');
// https://formatjs.io/docs/polyfills/intl-datetimeformat/#default-timezone
let RNLocalize = require('react-native-localize');
if ('__setDefaultTimeZone' in Intl.DateTimeFormat) {
Intl.DateTimeFormat.__setDefaultTimeZone(RNLocalize.getTimeZone());
}
}
See the contributing guide to learn how to contribute to the repository and the development workflow.
MIT
FAQs
Performant Date Picker for React Native Paper
The npm package @nandorojo/react-native-paper-dates receives a total of 181 weekly downloads. As such, @nandorojo/react-native-paper-dates popularity was classified as not popular.
We found that @nandorojo/react-native-paper-dates demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.