@nestia/sdk
Advanced tools
Comparing version 1.0.4 to 1.0.5
@@ -0,0 +0,0 @@ import { INestiaSdkConfiguration } from "@nestia/sdk"; |
@@ -0,0 +0,0 @@ #!/usr/bin/env node |
@@ -0,0 +0,0 @@ "use strict"; |
@@ -43,2 +43,4 @@ "use strict"; | ||
for (const route of routeList) { | ||
if (route.tags.find((tag) => tag.name === "internal")) | ||
continue; | ||
const path = MapUtil_1.MapUtil.take(pathDict, get_path(route.path, route.parameters), () => ({})); | ||
@@ -45,0 +47,0 @@ path[route.method.toLowerCase()] = generate_route(checker, collection, tupleList, route); |
import * as nestia from "./module"; | ||
export * from "./module"; | ||
export default nestia; |
@@ -25,5 +25,9 @@ "use strict"; | ||
}; | ||
var __exportStar = (this && this.__exportStar) || function(m, exports) { | ||
for (var p in m) if (p !== "default" && !Object.prototype.hasOwnProperty.call(exports, p)) __createBinding(exports, m, p); | ||
}; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
const nestia = __importStar(require("./module")); | ||
__exportStar(require("./module"), exports); | ||
exports.default = nestia; | ||
//# sourceMappingURL=index.js.map |
{ | ||
"name": "@nestia/sdk", | ||
"version": "1.0.4", | ||
"version": "1.0.5", | ||
"description": "Nestia SDK and Swagger generator", | ||
@@ -13,2 +13,3 @@ "main": "lib/index.js", | ||
"dev": "rimraf lib && ttsc --watch", | ||
"package": "npm publish --access public", | ||
"prettier": "prettier --write ./**/*.ts", | ||
@@ -15,0 +16,0 @@ "test": "node lib/test" |
@@ -1,2 +0,2 @@ | ||
# Nestia SDK | ||
# Nestia SDK Generator | ||
[](https://github.com/samchon/nestia/blob/master/LICENSE) | ||
@@ -14,4 +14,7 @@ [](https://www.npmjs.com/package/@nestia/sdk) | ||
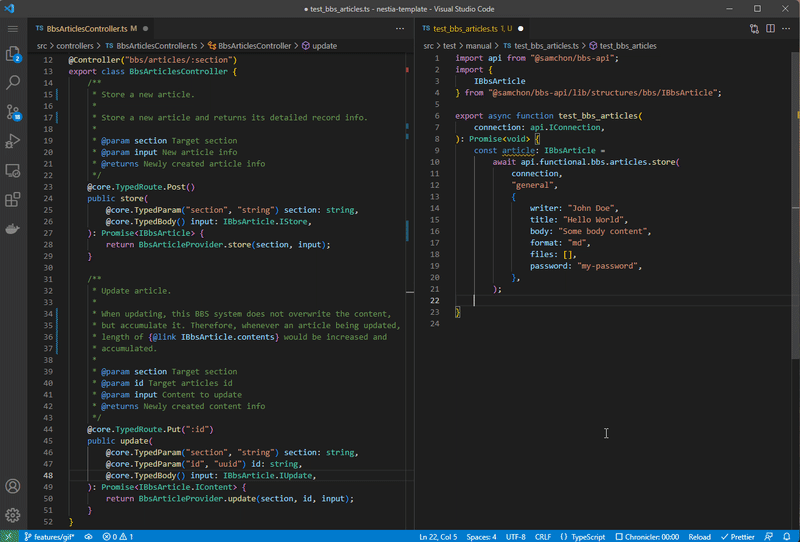 | ||
## Setup | ||
@@ -66,3 +69,3 @@ ### Boilerplate Project | ||
If you've configured `nestia.config.ts` file, you can omit all options like below. About the `nestia.config.ts` file, read [Guide Documents - Configuration](https://github.com/samchon/nestia/wiki/Configuration) | ||
If you've configured `nestia.config.ts` file, you can omit all options like below. About the `nestia.config.ts` file, read [Guide Documents -> SDK Generator -> Configuration](https://github.com/samchon/nestia/wiki/SDK-Generator#configuration) | ||
@@ -74,58 +77,9 @@ ```bash | ||
If you want to know more, visit [Guide Documents](https://github.com/samchon/nestia/wiki). | ||
## Demonstration | ||
When you generate SDK library through `npx nestia sdk` command, `@nestia/sdk` will generate below code, by analyzing your backend source code in the compilation level. | ||
```typescript | ||
import { Fetcher, IConnection } from "@nestia/fetcher"; | ||
import { IBbsArticle } from "../../../structures/IBbsArticle"; | ||
/** | ||
* Store a new content. | ||
* | ||
* @param input Content to store | ||
* @returns Newly archived article | ||
*/ | ||
export function store( | ||
connection: api.IConnection, | ||
input: IBbsArticle.IStore | ||
): Promise<IBbsArticle> { | ||
return Fetcher.fetch( | ||
connection, | ||
store.ENCRYPTED, | ||
store.METHOD, | ||
store.path(), | ||
input | ||
); | ||
} | ||
export namespace store { | ||
export const METHOD = "POST" as const; | ||
export function path(): string { | ||
return "/bbs/articles"; | ||
} | ||
} | ||
``` | ||
With SDK library, client developers would get take advantages of TypeScript like below. | ||
If you want to learn how to distribute SDK library, visit and read [Guide Documents - Distribution](https://github.com/samchon/nestia/wiki/Distribution). | ||
```typescript | ||
import api from "@bbs-api"; | ||
import typia from "typia"; | ||
export async function test_bbs_article_store(connection: api.IConnection) { | ||
const article: IBbsArticle = await api.functional.bbs.articles.store( | ||
connection, | ||
{ | ||
name: "John Doe", | ||
title: "some title", | ||
content: "some content", | ||
} | ||
); | ||
typia.assert(article); | ||
console.log(article); | ||
} | ||
``` | ||
- Generators | ||
- [Swagger Documents](https://github.com/samchon/nestia/wiki/SDK-Generator#swagger-documents) | ||
- [SDK Library](https://github.com/samchon/nestia/wiki/SDK-Generator#sdk-library) | ||
- Advanced Usage | ||
- [Comment Tags](https://github.com/samchon/nestia/wiki/SDK-Generator#comment-tags) | ||
- [Configuration](https://github.com/samchon/nestia/wiki/SDK-Generator#configuration) |
@@ -0,0 +0,0 @@ import { HashMap } from "tstl/container/HashMap"; |
@@ -0,0 +0,0 @@ import ts from "typescript"; |
@@ -0,0 +0,0 @@ import { HashMap } from "tstl/container/HashMap"; |
@@ -0,0 +0,0 @@ import path from "path"; |
@@ -0,0 +0,0 @@ import { equal } from "tstl/ranges/module"; |
@@ -0,0 +0,0 @@ import fs from "fs"; |
@@ -0,0 +0,0 @@ export namespace CommandParser { |
@@ -0,0 +0,0 @@ import { WorkerServer } from "tgrid/protocols/workers/WorkerServer"; |
@@ -0,0 +0,0 @@ export namespace NestiaConfigCompilerOptions { |
@@ -0,0 +0,0 @@ import cli from "cli"; |
@@ -5,2 +5,3 @@ import fs from "fs"; | ||
import { Singleton } from "tstl/thread/Singleton"; | ||
import { assert } from "typia"; | ||
@@ -7,0 +8,0 @@ |
@@ -0,0 +0,0 @@ #!/usr/bin/env node |
@@ -0,0 +0,0 @@ import fs from "fs"; |
import { Vector } from "tstl/container/Vector"; | ||
import { Pair } from "tstl/utility/Pair"; | ||
import ts from "typescript"; | ||
import { Escaper } from "typia/lib/utils/Escaper"; | ||
@@ -5,0 +6,0 @@ |
@@ -0,0 +0,0 @@ import fs from "fs"; |
@@ -6,2 +6,3 @@ import fs from "fs"; | ||
import ts from "typescript"; | ||
import { IJsonApplication, IJsonSchema } from "typia"; | ||
@@ -41,2 +42,4 @@ import { CommentFactory } from "typia/lib/factories/CommentFactory"; | ||
for (const route of routeList) { | ||
if (route.tags.find((tag) => tag.name === "internal")) continue; | ||
const path: ISwaggerDocument.IPath = MapUtil.take( | ||
@@ -43,0 +46,0 @@ pathDict, |
import * as nestia from "./module"; | ||
export * from "./module"; | ||
export default nestia; |
@@ -0,0 +0,0 @@ import ts from "typescript"; |
export * from "./INestiaConfig"; | ||
export * from "./NestiaSdkApplication"; |
@@ -0,0 +0,0 @@ import fs from "fs"; |
@@ -0,0 +0,0 @@ import { ParamCategory } from "./ParamCategory"; |
@@ -0,0 +0,0 @@ import ts from "typescript"; |
@@ -0,0 +0,0 @@ import { IJsonComponents, IJsonSchema } from "typia"; |
@@ -0,0 +0,0 @@ import ts from "typescript"; |
@@ -0,0 +0,0 @@ export type MethodType = "GET" | "POST" | "PUT" | "PATCH" | "DELETE"; |
export type ParamCategory = "param" | "query" | "body"; |
@@ -0,0 +0,0 @@ import { hash } from "tstl/functional/hash"; |
@@ -0,0 +0,0 @@ export namespace ArrayUtil { |
@@ -0,0 +0,0 @@ import path from "path"; |
@@ -0,0 +0,0 @@ export namespace MapUtil { |
@@ -0,0 +0,0 @@ export type StripEnums<T extends Record<string, any>> = { |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
5586
310873
82