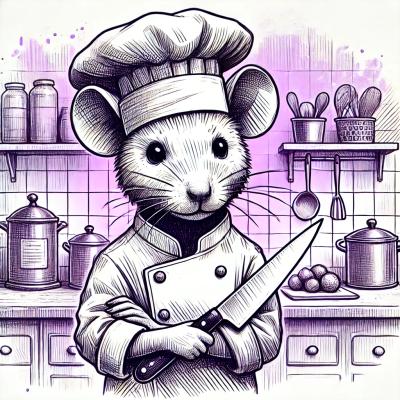
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@netlify/auth0
Advanced tools
The Netlify Auth0 wrapper makes it easy to use the Beta Auth0 integration on Netlify at runtime.
The Netlify Auth0 wrapper makes it easy to use the Beta Auth0 integration on Netlify at runtime.
It makes use of the serverless-jwt package, and injects it with the values defined in the UI by using the integration.
To add authentication and authorization checks to a handler, you can wrap it with the withAuth0
method.
// netlify/functions/user.ts
import type { Handler } from "@netlify/functions";
import { withAuth0 } from "@netlify/auth0";
export const handler: Handler = withAuth0(
async (event, context) => {
// The requesting user has the role "admin", so
// we can return the data
const users = [
//....
];
return {
statusCode: 200,
body: JSON.stringify(users),
};
},
{
auth0: {
// Will return 401 if the user isn't authenticated
required: true,
// Will return 403 if the authenticated user does
// not have the role "admin"
roles: ["admin"],
},
}
);
To call this handler, you should pass a JWT token in the Authorization
header with the prefix Bearer
/
// In the frontend....
const userResponse = await fetch("/.netlify/functions/users", {
method: "GET",
headers: {
"Authorization": `Bearer ${token}`
}
});
// ...
To chain with another integration, you can import the wrap
method from @netlify/integrations
. An example of this can be seen on the netlify docs.
Options can be set by passing in an object to the second parameter of the withAuth0
method. To enable the chaining mentioned above, the object must have a property auth0
which has the option values set.
Option | Type | Description |
---|---|---|
required | bool | If set to true , the handler will return 401 if no token is present or the token is invalid |
roles | string[] | If this array is truthy , it requires all roles to be present in the scope claim of the JWT |
FAQs
The Netlify Auth0 wrapper makes it easy to use the Beta Auth0 integration on Netlify at runtime.
The npm package @netlify/auth0 receives a total of 17 weekly downloads. As such, @netlify/auth0 popularity was classified as not popular.
We found that @netlify/auth0 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 20 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.