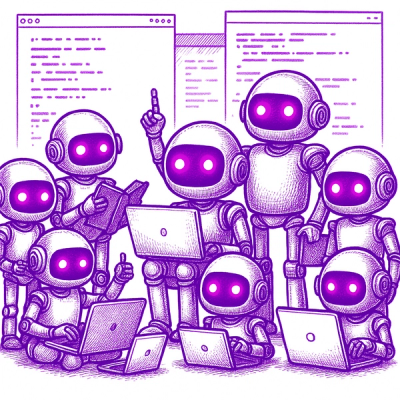
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
@next-testing/api
Advanced tools
Next API Test Suite - Simplifying the mocking and asserting of Next API Request & Responses
Simplifying the struggle of mocking and reimplementing NextAPIRequests and NextAPIResponses in order to test and validate NextJS serverless functions.
Choose your favorite package manager to install the next-testing library
npm install --save-dev @next-testing/api
yarn add -D @next-testing/api
pnpm add -D @next-testing/api
GET
Requestimport ServerlessFunctionApi from './pages/api/v1/myApi'
import { NextApiRequestBuilder, ResponseMock } from '@next-testing/api'
test("Get Request and Response Mock", () => {
const req = new NextApiRequestBuilder().setMethod('GET').build()
const res = ResponseMock<MyResult>()
ServerlessFunctionApi(req, res)
expect(res.getStatusCode()).toEqual(405)
expect(res.getBodyJson()).toStrictEqual({
success: false,
message: 'Method not allowed',
})
})
POST
Requestimport ServerlessFunctionApi from './pages/api/v1/myApi'
import { NextApiRequestBuilder, ResponseMock } from '@next-testing/api'
it('Post Request and Response Mock', async () => {
const req = new NextApiRequestBuilder().setMethod('POST').build()
const res = ResponseMock<MyResult>()
ServerlessFunctionApi(req, res)
expect(res.getStatusCode()).toEqual(401)
expect(res.getBodyJson()).toStrictEqual({
success: false,
message: 'access denied',
})
})
import ServerlessFunctionApi from './pages/api/v1/myApi'
import { NextApiRequestBuilder, ResponseMock } from '@next-testing/api'
it('Mock Request and Response with headers', async () => {
const req = new NextApiRequestBuilder()
.setMethod('POST')
.setHeaders({ authorization: 'Bearer ABC123' })
.build()
const res = ResponseMock<CronResult>()
ServerlessFunctionApi(req, res)
expect(res.getStatusCode()).toEqual(401)
expect(res.getBodyJson()).toStrictEqual({
success: false,
message: 'access denied',
})
})
import ServerlessFunctionApi from './pages/api/v1/myApi'
import { NextApiRequestBuilder, ResponseMock } from '@next-testing/api'
it('Mock Request and Response with headers', async () => {
const req = new NextApiRequestBuilder()
.setMethod('POST')
.setHeaders({ authorization: 'Bearer ABC123' })
.setCookies({ apiKey: "mytoken" })
.setBody({
posts: [{
content: "hello world"
}]
})
.build()
const res = ResponseMock<CronResult>()
ServerlessFunctionApi(req, res)
expect(res.getStatusCode()).toEqual(401)
expect(res.getBodyJson()).toStrictEqual({
success: false,
message: 'access denied',
})
})
If your handler depends on some parameters found in the path, eg. it's defined
in pages/api/[foo]/echo
, then you can specify it with setQuery()
.
import EchoHandler from './pages/api/[foo]/echo'
import { NextApiRequestBuilder, ResponseMock } from '@next-testing/api'
it('Mock Request and Response with route parameters', async () => {
const req = new NextApiRequestBuilder()
.setMethod('GET')
.setQuery({
foo: 'hello'
})
.build()
const res = ResponseMock()
EchoHandler(req, res)
expect(res.getStatusCode()).toEqual(200)
expect(res.getBodyJson()).toStrictEqual({
foo: 'hello',
})
})
FAQs
Next API Test Suite - Simplifying the mocking and asserting of Next API Request & Responses
The npm package @next-testing/api receives a total of 0 weekly downloads. As such, @next-testing/api popularity was classified as not popular.
We found that @next-testing/api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.