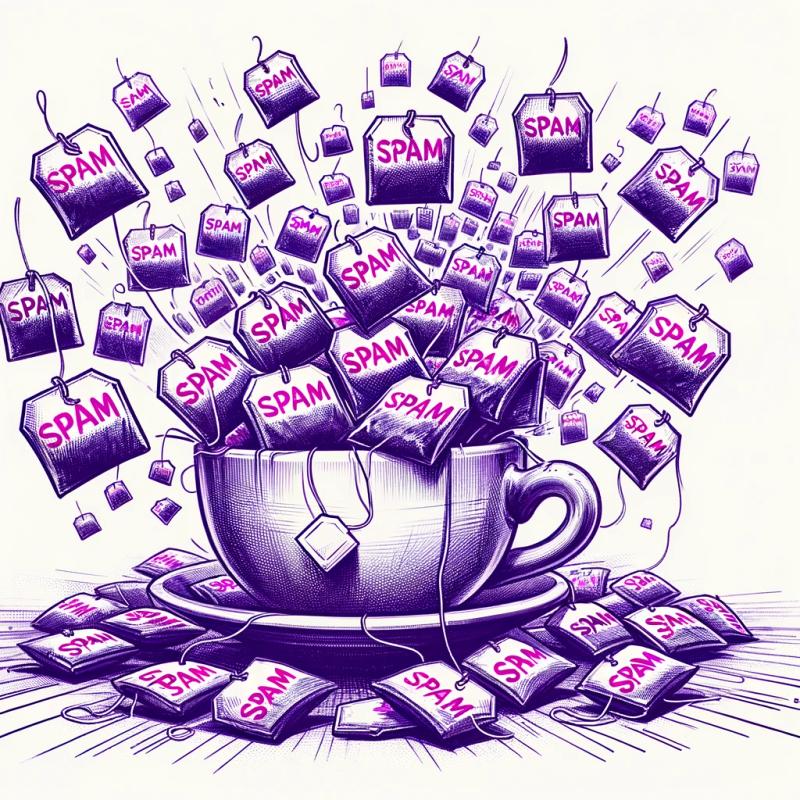
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@ngx-utilities/http-retry
Advanced tools
Readme
A configurable Angular HTTP interceptor to retry GET requests and respond to errors and flaky connections.
To install this library, run:
npm install @ngx-utilities/http-retry --save
-or- yarn add @ngx-utilities/http-retry
and then import HttpRetryModule
in your Angular AppModule
:
// app.module.ts
import { HttpRetryModule } from '@ngx-utilities/http-retry';
@NgModule({
imports: [
HttpRetryModule.forRoot()
],
providers: [
networkErrorRetryStrategyProvider,
serverUnavailableRetryStrategyProvider
],
bootstrap: [AppComponent]
})
export class AppModule { }
You configure http-retry
by providing (via Angular dependency injection) a collection of
injectable classes that implement the HttpRequestRetryStrategy
interface. These "retry strategies"
tell http-retry
which status codes to retry, how many times to retry, and when to stop
retrying. The global HTTP interceptor provided by HttpRetryModule
will do nothing if you do not
provide any retry strategies.
In addition to being thrown by the http request observable like normal, the last HttpErrorResponse
received when http-retry
stops retrying a request is passed to the retry strategy's onFailure
method and emitted on the HttpRetryService
's httpRetryFailures
observable.
HttpRequestRetryStrategy
// network-error.retry-strategy.ts
import { Injectable, Provider } from '@angular/core';
import { HttpRequestRetryStrategy, HTTP_REQUEST_RETRY_STRATEGIES } from '@ngx-utilities/http-retry';
import { NetworkStatusService } from './../services/network-status.service';
@Injectable()
export class NetworkErrorRetryStrategy implements HttpRequestRetryStrategy {
// status code 0 means there was a network error (e.g. a timeout)
readonly statuses = [0];
readonly maxCount = 3;
delayFn(retryNumber: number) {
// retry immediately, wait 3 seconds and try again, and then stop due the max count
return retryNumber === 1 ? 0 : 3000;
}
onFailure(error: HttpErrorResponse) {
console.log('network error', error.status, error.url);
}
}
export const networkErrorRetryStrategyProvider: Provider = {
provide: HTTP_REQUEST_RETRY_STRATEGIES,
useClass: NetworkErrorRetryStrategy,
multi: true
};
// server-unavailable.retry-strategy.ts
@Injectable()
export class ServerUnavailableRetryStrategy implements HttpRequestRetryStrategy {
// retry if the server is temporarily unavailable (e.g. for maintenance)
readonly statuses = [502, 503];
readonly maxCount = 10;
delayFn() {
return 3000;
}
onFailure(error: HttpErrorResponse) {
console.log('server unavailable error', error.status, error.url);
}
}
export const serverUnavailableRetryStrategyProvider: Provider = {
provide: HTTP_REQUEST_RETRY_STRATEGIES,
useClass: ServerUnavailableRetryStrategy,
multi: true
};
HttpRetryService
(optional)// my.component.ts
import { HttpRetryService } from '@ngx-utilities/http-retry';
export class MyComponent implements OnInit {
constructor(private readonly httpRetryService: HttpRetryService) { }
ngOnInit() {
this.httpRetryService.httpRetryFailures.subscribe(error => {
console.log('global http retry error listener', error.status, error.url);
});
}
}
The core functionality of this library is exposed as a RxJS operator that takes an an array of
retry strategies which can be instances of classes or plain objects that implement the
HttpRequestRetryStrategy
interface. You can use this operator directly if you do not wish to use
the global interceptor provided by HttpRetryModule
.
import { httpRequestRetry } from '@ngx-utilities/http-retry';
export class MyComponent implements OnInit {
constructor(private readonly httpClient: HttpClient) { }
ngOnInit() {
this.httpClient.get('/some/url').pipe(httpRequestRetry(retryStrategies)).subscribe();
}
}
http-retry
will add a header named X-Request-Attempt-Number
to each request it sends that
with the number of the current attempt. This was added primarily for testing. But it's plausible
that this could be useful information to track on the server in some scenarios, so this header is
added in production. A future version may allow disabling this option if needed.
MIT © Kevin Phelps
FAQs
[](https://www.npmjs.com/package/@ngx-utilities/http-retry)
The npm package @ngx-utilities/http-retry receives a total of 6 weekly downloads. As such, @ngx-utilities/http-retry popularity was classified as not popular.
We found that @ngx-utilities/http-retry demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.