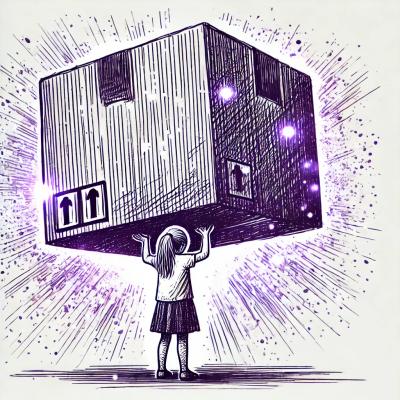
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
@node-ipc/js-queue
Advanced tools
Works great in node.js, webpack, browserify, or any other commonjs loader or compiler. To use in plain old vanilla browser javascript without common js just replace the requires in the examples with script tags. We show that below too.
js-queue
also exposes the easy-stack
stack via require('js-queue/stack.js')
this file exposes an ES6 stack which allows for Last In First Out (LIFO) queuing. This can come in handy depending on your application needs, check out the easy-stack javascript documentation it follows the js-queue
interface but is node 6 or greater as it uses ES6 classes.
npm install js-queue
npm info : See npm trends and stats for js-queue
GitHub info :
Package details websites :
This work is licenced via the MIT Licence.
key | type | paramaters | default | description |
---|---|---|---|---|
add | function | any number of functions | adds all parameter functions to queue and starts execution if autoRun is true, queue is not already running and queue is not forcibly stopped | |
next | function | executes next item in queue if queue is not forcibly stopped | ||
clear | function | removes remaining items in the queue | ||
contents | Array | Queue instance contents | ||
autoRun | Bool | true | should autoRun queue when new item added | |
stop | Bool | false | setting this to true will forcibly prevent the queue from executing |
var Queue=require('js-queue');
//create a new queue instance
var queue=new Queue;
for(var i=0; i<50; i++){
//add a bunch of stuff to the queue
queue.add(makeRequest);
}
function makeRequest(){
//do stuff
console.log('making some request');
this.next();
}
The only difference is including via a script tag instead of using require.
<html>
<head>
<!-- this is the only difference -->
<script src='./queue-vanilla.js'></script>
<script>
console.log('my awesome app script');
var queue=new Queue;
for(var i=0; i<50; i++){
queue.add(makeRequest);
}
function makeRequest(){
console.log('making some request');
this.next();
}
</script>
</head>
<body>
</body>
</html>
This allows you to start adding requests immediately and only execute if the websocket is connected. To use in plain browser based JS without webpack or browserify just replace the requires with the script tag.
var Queue=require('js-queue');
//ws-share just makes it easier to share websocket code and ensure you don't open a websocket more than once
var WS=require('ws-share');
//js-message makes it easy to create and parse normalized JSON messages.
var Message=require('js-message');
//create a new queue instance
var queue=new Queue;
//force stop until websocket opened
queue.stop=true;
var ws=null;
function startWS(){
//websocket.org rocks
ws=new WS('wss://echo.websocket.org/?encoding=text');
ws.on(
'open',
function(){
ws.on(
'message',
handleResponse
);
//now that websocket is opened allow auto execution
queue.stop=false;
queue.next();
}
);
ws.on(
'error',
function(err){
//stop execution of queue if there is an error because the websocket is likely closed
queue.stop=true;
//remove remaining items in the queue
queue.clear();
throw(err);
}
);
ws.on(
'close',
function(){
//stop execution of queue when the websocket closed
queue.stop=true;
}
);
}
//simulate a lot of requests being queued up for the websocket
for(var i=0; i<50; i++){
queue.add(makeRequest);
}
var messageID=0;
function handleResponse(e){
var message=new Message;
message.load(e.data);
console.log(message.type,message.data);
}
function makeRequest(){
messageID++;
var message=new Message;
message.type='testMessage';
message.data=messageID;
ws.send(message.JSON);
this.next();
}
startWS();
var Queue=require('js-queue');
//MyAwesomeQueue inherits from Queue
MyAwesomeQueue.prototype = new Queue;
//Constructor will extend Queue
MyAwesomeQueue.prototype.constructor = MyAwesomeQueue;
function MyAwesomeQueue(){
//extend with some stuff your app needs,
//maybe npm publish your extention with js-queue as a dependancy?
Object.defineProperties(
this,
{
isStopped:{
enumerable:true,
get:checkStopped,
set:checkStopped
},
removeThirdItem:{
enumerable:true,
writable:false,
value:removeThird
}
}
);
//enforce Object.assign for extending by locking down Class structure
//no willy nilly cowboy coding
Object.seal(this);
function checkStopped(){
return this.stop;
}
function removeThird(){
//get the queue content
var list=this.contents;
//modify the queue content
list.splice(2,1);
//save the modified queue content
this.contents=list;
return this.contents;
}
}
FAQs
Simple JS queue with auto run for node and browsers
The npm package @node-ipc/js-queue receives a total of 426,691 weekly downloads. As such, @node-ipc/js-queue popularity was classified as popular.
We found that @node-ipc/js-queue demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.