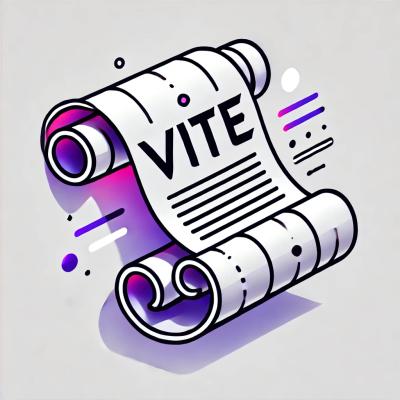
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
@nodesecure/conformance
Advanced tools
SPDX license conformance for NodeSecure
This package is available in the Node Package Repository and can be easily installed with npm or yarn.
$ npm i @nodesecure/conformance
# or
$ yarn add @nodesecure/conformance
Extract all licenses and their SPDX conformance from a given location
import * as conformance from "@nodesecure/conformance";
// Asynchronous
{
const licenses = await conformance.extractLicenses(
process.cwd()
);
console.log(licenses);
}
// Synchronous
{
const licenses = conformance.extractLicensesSync(
process.cwd()
);
console.log(licenses);
}
Or detect SPDX conformance from a given Expression
import { licenseIdConformance } from "@nodesecure/conformance";
const conformance = licenseIdConformance("MIT").unwrap();
console.log(conformance);
/*
{
uniqueLicenseIds: ["MIT"],
spdxLicenseLinks: ["https://spdx.org/licenses/MIT.html#licenseText"],
spdx: {
osi: true,
fsf: true,
fsfAndOsi: true,
includesDeprecated: false
}
}
*/
export interface SpdxLicenseConformance {
licenses: Record<string, string>;
spdx: {
osi: boolean;
fsf: boolean;
fsfAndOsi: boolean;
includesDeprecated: boolean;
};
}
Extract License name from a given file content. Return null
if it failed to detect the license.
Search and parse all licenses at the given location.
Return all licenses with their SPDX conformance.
interface SpdxFileLicenseConformance extends SpdxLicenseConformance {
fileName: string;
}
interface SpdxUnidentifiedLicense {
licenseId: string;
reason: string;
}
interface SpdxExtractedResult {
/**
* List of licenses, each with its SPDX conformance details.
* This array includes all licenses found, conforming to the SPDX standards.
*/
licenses: SpdxFileLicenseConformance[];
/**
* A unique list of license identifiers (e.g., 'MIT', 'ISC').
* This list does not contain any duplicate entries.
* It represents the distinct licenses identified.
*/
uniqueLicenseIds: string[];
/**
* List of licenses that do not conform to SPDX standards or have invalid/unidentified identifiers.
* This array includes licenses that could not be matched to valid SPDX identifiers.
*/
unidentifiedLicenseIds?: SpdxUnidentifiedLicense[];
}
Same as extractLicenses
but use synchronous FS API.
To update the src/data/spdx.ts
file just run the following npm script:
$ npm run spdx:refresh
It will fetch SPDX licenses here.
MIT
FAQs
SPDX license conformance for NodeSecure
The npm package @nodesecure/conformance receives a total of 207 weekly downloads. As such, @nodesecure/conformance popularity was classified as not popular.
We found that @nodesecure/conformance demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.