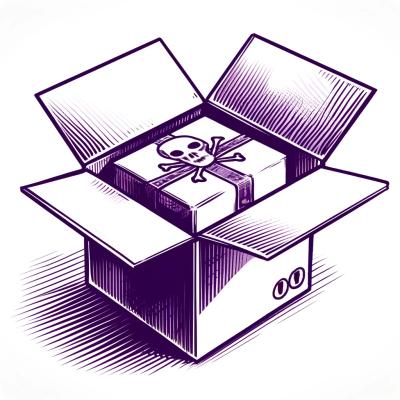
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@pdf-lib/upng
Advanced tools
A small, fast and advanced PNG / APNG encoder and decoder
This project is a fork of UPNG.js
and was created for use in pdf-lib
. The maintainer of the original repo does not publish it to NPM. That is the primary purpose of this fork. In addition, an index.d.ts
file has been added (copied directly from @types/upng-js
) to makes TypeScript definitions available without requiring additional packages to be installed.
UPNG.toRGBA8
// Import the UPNG class
import UPNG from '@pdf-lib/upng';
// Create a UPNG object
const pngImage = UPNG.decode(/* Uint8Array containing bytes of PNG image */);
// `pixels` is a 1D array (in rgba order) of decoded pixel data
const pixels = pngImage.UPNG.toRGBA8();
To install the latest stable version:
# With npm
npm install --save @pdf-lib/upng
# With yarn
yarn add @pdf-lib/upng
This assumes you're using npm or yarn as your package manager.
You can also download @pdf-lib/upng
as a UMD module from unpkg. The UMD builds have been compiled to ES5, so they should work in any modern browser. UMD builds are useful if you aren't using a package manager or module bundler. For example, you can use them directly in the <script>
tag of an HTML page.
The following builds are available:
When using a UMD build, you will have access to a global window.UPNG
variable. This variable contains the UPNG
class exported by @pdf-lib/upng
. For example:
// NPM module
import UPNG from '@pdf-lib/upng';
const pngImage = UPNG.decode(/* ... */)
// UMD module
var pngImage = window.UPNG.decode(/* ... */)
UPNG.js supports APNG and the interface expects "frames". Regular PNG is just a single-frame animation (single-item array).
UPNG.encode(imgs, w, h, cnum, [dels])
imgs
: array of frames. A frame is an ArrayBuffer containing the pixel data (RGBA, 8 bits per channel)w
, h
: width and height of the imagecnum
: number of colors in the result; 0: all colors (lossless PNG)dels
: array of millisecond delays for each frame (only when 2 or more frames)UPNG.js can do a lossy minification of PNG files, similar to TinyPNG and other tools. It performed quantization with k-means algorithm in the past, but now we use K-d trees.
Lossy compression is allowed by the last parameter cnum
. Set it to zero for a lossless compression, or write the number of allowed colors in the image. Smaller values produce smaller files. Or just use 0 for lossless / 256 for lossy.
// Read RGBA from canvas and encode with UPNG
var dta = ctx.getImageData(0,0,200,300).data; // ctx is Context2D of a Canvas
// dta = new Uint8Array(200 * 300 * 4); // or generate pixels manually
var png = UPNG.encode([dta.buffer], 200, 300, 0); console.log(new Uint8Array(png));
UPNG.encodeLL(imgs, w, h, cc, ac, depth, [dels])
- low-level encodeimgs
: array of frames. A frame is an ArrayBuffer containing the pixel data (corresponding to following parameters)w
, h
: width and height of the imagecc
, ac
: number of color channels (1 or 3) and alpha channels (0 or 1)depth
: bit depth of pixel data (1, 2, 4, 8, 16)dels
: array of millisecond delays for each frame (only when 2 or more frames)This function does not do any optimizations, it just stores what you give it. There are two cases when it is useful:
Supports all color types (including Grayscale and Palettes), all channel depths (1, 2, 4, 8, 16), interlaced images etc. Opens PNGs which other libraries can not open (tested with PngSuite).
UPNG.decode(buffer)
buffer
: ArrayBuffer containing the PNG filewidth
: the width of the imageheight
: the height of the imagedepth
: number of bits per channelctype
: color type of the file (Truecolor, Grayscale, Palette ...)frames
: additional info about frames (frame delays etc.)tabs
: additional chunks of the PNG filedata
: pixel data of the imagePNG files may have a various number of channels and a various color depth. The interpretation of data
depends on the current color type and color depth (see the PNG specification).
UPNG.toRGBA8(img)
img
: PNG image object (returned by UPNG.decode())var img = UPNG.decode(buff); // put ArrayBuffer of the PNG file into UPNG.decode
var rgba = UPNG.toRGBA8(img)[0]; // UPNG.toRGBA8 returns array of frames, size: width * height * 4 bytes.
PNG format uses the Inflate algorithm. Right now, UPNG.js calls Pako.js for the Inflate and Deflate method.
UPNG.js contains a very good Quantizer of 4-component 8-bit vectors (i.e. pixels). It can be used to generate nice color palettes (e.g. Photopea uses UPNG.js to make palettes for GIF images).
Quantization consists of two important steps: Finding a nice palette and Finding the closest color in the palette for each sample (non-trivial for large palettes). UPNG perfroms both steps.
var res = UPNG.quantize(data, psize);
data
: ArrayBuffer of samples (byte length is a multiple of four)psize
: Palette size (how many colors you want to have)The result object "res" has following properties:
abuf
: ArrayBuffer corresponding to data
, where colors are remapped by a paletteinds
: Uint8Array : the index of a color for each sample (only when psize
<=256)plte
: Array : the Palette - a list of colors, plte[i].est.q
and plte[i].est.rgba
is the color valuedata
.FAQs
Small, fast and advanced PNG / APNG encoder and decoder
The npm package @pdf-lib/upng receives a total of 847,610 weekly downloads. As such, @pdf-lib/upng popularity was classified as popular.
We found that @pdf-lib/upng demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.