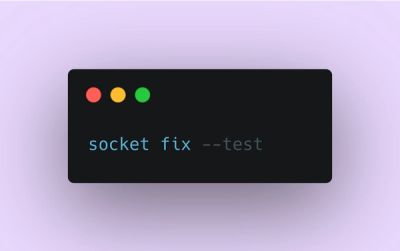
Product
Introducing Socket Fix for Safe, Automated Dependency Upgrades
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
@prodfox/react-pdf-editor
Advanced tools
View, edit, and Chat-with-your-PDF with AI.
Add View to your app for free. Learn pricing for all features here
https://www.signeasynow.com/edit-your-pdf
Want to see the source code for the above demo? Find it here.
Copy-paste the pdf-ui
folder here into your own public
folder.
Install
npm install --save @prodfox/react-pdf-editor
or
yarn add @prodfox/react-pdf-editor
import { useRef } from 'react';
import { useCreateIframeAndLoadViewer } from "@prodfox/react-pdf-editor";
function App() {
const containerRef = useRef(null);
const { download } = useCreateIframeAndLoadViewer({
container: containerRef,
licenseKey: "sandbox",
locale: "en",
tools: {
editing: [
"extract",
"remove",
"move"
],
thumbnails: [
"zoom",
"expand"
],
general: [
"thumbnails",
"download",
"search",
"panel-toggle",
"zoom"
],
},
files: [
{
url: "https://pdftron.s3.amazonaws.com/downloads/pl/demo-annotated.pdf",
name: "my-file1.pdf"
},
{
url: "https://pdftron.s3.amazonaws.com/downloads/pl/demo-annotated.pdf",
name: "my-file2.pdf"
}
],
});
return (
<div>
<button onClick={download}>Download</button>
<div className="container" id="pdf" ref={containerRef}>
</div>
</div>
);
}
export default App;
Required
The HTML element to attach the PDF viewer to.
Object
{}
Control what tools are available in the UI. Available keys are thumbnails
, general
, editing
, ...
useCreateIframeAndLoadViewer({
tools: {
thumbnails: ...,
general: ...,
editing: ...,
},
...other parameters
});
Object
[]
Field | Description |
---|---|
zoom | Enable zoom in/out of the document in view |
search | Enable search functions |
download | Enable downloading the document |
thumbnails | Enable a thumbnails panel |
panel-toggle | Enable the left-side panel to be togglable |
chat | Enable AI conversations with your PDF (user ID is required after 10 questions) |
useCreateIframeAndLoadViewer({
tools: {
general: [
"zoom",
"search",
"download",
"thumbnails",
"panel-toggle"
],
},
...other parameters
});
Object
[]
Field | Description |
---|---|
zoom | Enable a slider above thumbnails to increase/decrease the size of the thumbnails |
expand | Enable the thumbnails bar to be expandable to the full screen |
useCreateIframeAndLoadViewer({
tools: {
thumbnails: [
"zoom",
"expand"
],
},
...other parameters
});
Object
[]
Field | Description |
---|---|
remove | Enable the ability to remove pages |
rotation | Enable the rotation of individual pages |
extraction | Enabling extracting out a set of pages into one document |
move | Re-arrange pages in a document |
useCreateIframeAndLoadViewer({
tools: {
editing: [
"remove",
"rotation",
"extraction",
"move"
],
},
...other parameters
});
string
en
Optional
Options:
en
- English
es
- Spanish
ru
- Russian
(Reach out if you need a particular language added)
Function
optional
Callback when a file fails to upload
useCreateIframeAndLoadViewer({
onFileFailed: (errorMessage) => {
// handle the failure as you need
}
});
string
optional
Defaults to regular
. Set it to split
to enable being able to select split markers to be then used for splitting a document into several documents.
Combine several files into one
const { combineFiles } = useCreateIframeAndLoadViewer({
...
});
combineFiles();
Listen for when the pages are loaded for the active document
const { pagesLoaded } = useCreateIframeAndLoadViewer({
...
});
if (pagesLoaded) {
// logic here
}
Download
const { download } = useCreateIframeAndLoadViewer({
...
});
download();
Listen for when the PDF editor is ready to accept commands
const { isReady } = useCreateIframeAndLoadViewer({
...
});
if (isReady) {
// logic here
}
Toggle displaying the full screen thumbnail view
const { toggleFullScreenThumbnails } = useCreateIframeAndLoadViewer({
...
});
toggleFullScreenThumbnails(true) // set this to true or false to open/close it.
Control the thumbnail zoom level. Ranges from 0 to 1.
const { setThumbnailZoom } = useCreateIframeAndLoadViewer({
...
});
setThumbnailZoom(0.5)
Toggle displaying the search bar on the right
const { toggleSearchbar } = useCreateIframeAndLoadViewer({
...
});
toggleSearchbar(true) // set this to true or false to open/close it.
Delete the AI conversation chat history
const { removeChatHistory } = useCreateIframeAndLoadViewer({
...
});
removeChatHistory()
Get the 0-indexed array of selected pages
const { selectedPages } = useCreateIframeAndLoadViewer({
...
});
Extract the selected pages
const { extractPages } = useCreateIframeAndLoadViewer({
...
});
extractPages()
Split the document into several documents based on the split markers the user selected.
const { splitPages } = useCreateIframeAndLoadViewer({
...
});
splitPages()
FAQs
## Core
We found that @prodfox/react-pdf-editor demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Product
We’re excited to announce a powerful new capability in Socket: historical data and enhanced analytics.