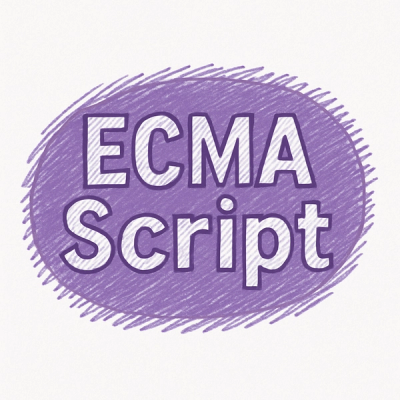
Security News
ECMAScript 2025 Finalized with Iterator Helpers, Set Methods, RegExp.escape, and More
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
@protobuf-ts/plugin
Advanced tools
The protocol buffer compiler plugin "protobuf-ts" generates TypeScript, gRPC-web, Twirp, and more.
@protobuf-ts/plugin is a TypeScript plugin for Protocol Buffers (protobuf). It generates TypeScript code from .proto files, enabling developers to work with protobuf messages in a type-safe manner. The plugin supports both client and server-side code generation, making it versatile for various use cases.
TypeScript Code Generation
This feature allows you to generate TypeScript code from .proto files. The generated code includes type definitions and service clients, making it easier to work with protobuf messages in a type-safe manner.
```json
{
"scripts": {
"generate": "protoc --plugin=protoc-gen-ts=./node_modules/.bin/protoc-gen-ts --ts_out=./generated -I ./protos ./protos/*.proto"
}
}
```
Client-Side Code Generation
This feature generates client-side code for interacting with protobuf services. The generated client can be used to make RPC calls to a server that implements the protobuf service.
```typescript
import { MyServiceClient } from './generated/my-service.client';
const client = new MyServiceClient('http://localhost:8080');
client.myMethod({ myField: 'value' }).then(response => {
console.log(response);
});
```
Server-Side Code Generation
This feature generates server-side code for implementing protobuf services. The generated server can be used to handle RPC calls from clients.
```typescript
import { MyServiceServer } from './generated/my-service.server';
class MyServiceImpl implements MyServiceServer {
myMethod(request) {
return { myField: 'response' };
}
}
```
protobufjs is a popular library for working with Protocol Buffers in JavaScript and TypeScript. It provides a runtime library and a code generator. Unlike @protobuf-ts/plugin, protobufjs focuses more on runtime parsing and serialization rather than generating TypeScript code.
ts-proto is another TypeScript code generator for Protocol Buffers. It generates idiomatic TypeScript code from .proto files, similar to @protobuf-ts/plugin. However, ts-proto aims to be more lightweight and closer to the original protobuf definitions.
grpc-tools is a set of tools for working with gRPC and Protocol Buffers. It includes a code generator for various languages, including TypeScript. While it provides similar functionality to @protobuf-ts/plugin, grpc-tools is more focused on gRPC and may not be as TypeScript-centric.
The protocol buffer compiler plugin for TypeScript: protobuf-ts
Installation:
# with npm:
npm install -D @protobuf-ts/plugin
# with yarn:
yarn add --dev @protobuf-ts/plugin
This will install the plugin as a development dependency.
Basic usage:
npx protoc --ts_out . --proto_path protos protos/my.proto
With some options:
npx protoc \
--ts_out . \
--ts_opt long_type_string \
--ts_opt optimize_code_size \
--proto_path protos \
protos/my.proto
protoc
is the protocol buffer compiler. protobuf-ts
installs it automatically.
Plugin parameters are documented in the MANUAL.
For a quick overview of protobuf-ts, check the repository README.
FAQs
The protocol buffer compiler plugin "protobuf-ts" generates TypeScript, gRPC-web, Twirp, and more.
The npm package @protobuf-ts/plugin receives a total of 217,699 weekly downloads. As such, @protobuf-ts/plugin popularity was classified as popular.
We found that @protobuf-ts/plugin demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.
Research
North Korean threat actors linked to the Contagious Interview campaign return with 35 new malicious npm packages using a stealthy multi-stage malware loader.