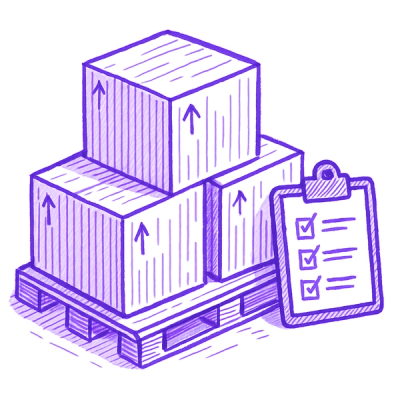
Security News
Open Source Maintainers Feeling the Weight of the EU’s Cyber Resilience Act
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
@protobuf-ts/protoc
Advanced tools
@protobuf-ts/protoc is a TypeScript library that provides tools for working with Protocol Buffers (protobuf). It allows you to generate TypeScript code from .proto files, making it easier to work with protobuf in TypeScript projects.
Generate TypeScript Code from .proto Files
This feature allows you to generate TypeScript code from .proto files. The generated code can then be used in your TypeScript projects to work with protobuf messages.
const { protoc } = require('@protobuf-ts/protoc');
protoc({
protoFiles: ['path/to/your/file.proto'],
outDir: 'path/to/output/directory'
}).then(() => {
console.log('TypeScript code generated successfully');
}).catch(err => {
console.error('Error generating TypeScript code:', err);
});
Compile Protobuf Definitions
This feature allows you to compile protobuf definitions from .proto files. The compiled definitions can be used to serialize and deserialize protobuf messages.
const { compile } = require('@protobuf-ts/protoc');
compile({
protoFiles: ['path/to/your/file.proto'],
outDir: 'path/to/output/directory'
}).then(() => {
console.log('Protobuf definitions compiled successfully');
}).catch(err => {
console.error('Error compiling protobuf definitions:', err);
});
protobufjs is a popular library for working with Protocol Buffers in JavaScript and TypeScript. It provides similar functionality to @protobuf-ts/protoc, including the ability to parse .proto files and generate JavaScript/TypeScript code. However, protobufjs is more widely used and has a larger community.
google-protobuf is the official Protocol Buffers library for JavaScript. It provides tools for working with protobuf messages, including serialization and deserialization. While it does not offer TypeScript code generation out of the box, it is a reliable and well-maintained library for working with protobuf in JavaScript.
ts-proto is a TypeScript-first library for working with Protocol Buffers. It generates idiomatic TypeScript code from .proto files, making it easy to work with protobuf messages in TypeScript projects. Compared to @protobuf-ts/protoc, ts-proto focuses more on TypeScript-specific features and type safety.
Installs the protocol buffer compiler "protoc" for you.
Installation (not necessary if you use the protobuf-ts plugin):
# with npm:
npm install @protobuf-ts/protoc
# with yarn:
yarn add @protobuf-ts/protoc
Now you can run protoc as usual, you just have to prefix your command with
npx
or yarn
:
# with npm:
npx protoc --version
# with yarn:
yarn protoc --version
If you do not already have protoc in your $PATH
, this will automatically
download the latest release of protoc for your platform from the github
release page, then run the executable with your arguments.
This package is not compatible with Yarn berry. Please use node-protoc.
Add the following to your package json:
"config": {
"protocVersion": "29.2"
}
$PATH
Add a protocVersion
to your package json, see above.
The script passes all given arguments to protoc and adds the following arguments:
--proto_path
that points to the include/
directory of the
downloaded release (skipped when found on $PATH
)--plugin
argument for all plugins found in node_modules/.bin/
--proto_path
argument for node_modules/@protobuf-ts/plugin
FAQs
Installs the protocol buffer compiler "protoc" for you.
The npm package @protobuf-ts/protoc receives a total of 295,595 weekly downloads. As such, @protobuf-ts/protoc popularity was classified as popular.
We found that @protobuf-ts/protoc demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.