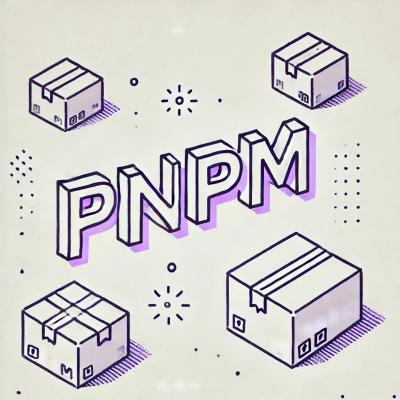
Security News
pnpm 10.12 Introduces Global Virtual Store and Expanded Version Catalogs
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.
@react-native-community/async-storage
Advanced tools
Asynchronous, persistent, key-value storage system for React Native.
An asynchronous, unencrypted, persistent, key-value storage system for React Native.
Head over to documentation to learn more.
Pull requests are welcome. Please open an issue first to discuss what you would like to change.
See the CONTRIBUTING file for more information.
MIT.
FAQs
Asynchronous, persistent, key-value storage system for React Native.
The npm package @react-native-community/async-storage receives a total of 63,313 weekly downloads. As such, @react-native-community/async-storage popularity was classified as popular.
We found that @react-native-community/async-storage demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.
Security News
Amaro 1.0 lays the groundwork for stable TypeScript support in Node.js, bringing official .ts loading closer to reality.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.