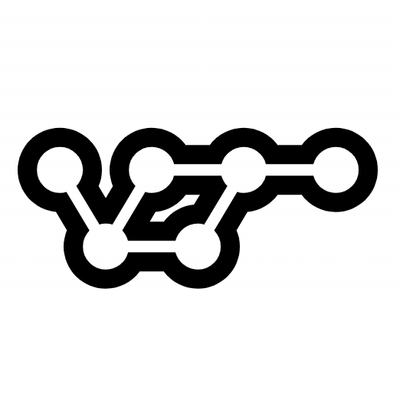
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@react-native-community/progress-bar-android
Advanced tools
Progress Bar component for React Native
Progress Bar Component for Android Devices
npm install @react-native-community/progress-bar-android --save
# or
yarn add @react-native-community/progress-bar-android
The package is automatically linked when building the app. All you need to do is:
npx pod-install
Run the following commands
$ react-native link @react-native-community/progress-bar-android
Libraries
➜ Add Files to [your project's name]
node_modules
➜ @react-native-community/progress-bar-android
and add RNCProgressBar.xcodeproj
libRNCProgressBar.a
to your project's Build Phases
➜ Link Binary With Libraries
Cmd+R
)android/app/src/main/java/[...]/MainActivity.java
import com.reactnativecommunity.androidprogressbar.RNCProgressBarPackage;
to the imports at the top of the filenew RNCProgressBarPackage()
to the list returned by the getPackages()
methodandroid/settings.gradle
:
include ':@react-native-community_progress-bar-android'
project(':@react-native-community_progress-bar-android').projectDir = new File(rootProject.projectDir, '../../node_modules/@react-native-community/progress-bar-android/android')
android/app/build.gradle
:
implementation project(':@react-native-community_progress-bar-android')
example
yarn install
yarn start
yarn android
import React from 'react';
import {View, StyleSheet, Text} from 'react-native';
import {ProgressBar} from '@react-native-community/progress-bar-android';
export default function App() {
return (
<View style={styles.container}>
<View style={styles.example}>
<Text>Circle Progress Indicator</Text>
<ProgressBar />
</View>
<View style={styles.example}>
<Text>Horizontal Progress Indicator</Text>
<ProgressBar styleAttr="Horizontal" />
</View>
<View style={styles.example}>
<Text>Colored Progress Indicator</Text>
<ProgressBar styleAttr="Horizontal" color="#2196F3" />
</View>
<View style={styles.example}>
<Text>Fixed Progress Value</Text>
<ProgressBar
styleAttr="Horizontal"
indeterminate={false}
progress={0.5}
/>
</View>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
example: {
marginVertical: 24,
},
});
Inherits View Props.
animating
Whether to show the ProgressBar (true, the default) or hide it (false).
Type | Required |
---|---|
bool | No |
color
Color of the progress bar.
Type | Required |
---|---|
color | No |
indeterminate
If the progress bar will show indeterminate progress. Note that this can only be false if styleAttr is Horizontal, and requires a progress
value.
Type | Required |
---|---|
indeterminateType | No |
progress
The progress value (between 0 and 1).
Type | Required |
---|---|
number | No |
styleAttr
Style of the ProgressBar. One of:
Type | Required |
---|---|
enum('Horizontal', 'Normal', 'Small', 'Large', 'Inverse', 'SmallInverse', 'LargeInverse') | No |
testID
Used to locate this view in end-to-end tests.
Type | Required |
---|---|
string | No |
FAQs
Progress Bar component for React Native
The npm package @react-native-community/progress-bar-android receives a total of 11,932 weekly downloads. As such, @react-native-community/progress-bar-android popularity was classified as popular.
We found that @react-native-community/progress-bar-android demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 31 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.