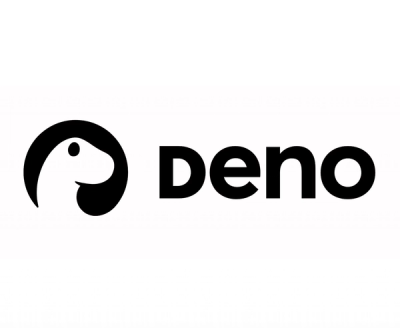
Security News
Deno 2.4 Brings Back deno bundle, Improves Dependency Management and Observability
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
@react-querybuilder/antd
Advanced tools
Official react-querybuilder compatibility package for Ant Design.
npm i react-querybuilder @react-querybuilder/antd @ant-design/icons antd
# OR yarn add / pnpm add / bun add
To configure the query builder to use Ant Design-compatible components, place QueryBuilderAntD
above QueryBuilder
in the component hierarchy.
import { QueryBuilderAntD } from '@react-querybuilder/antd';
import { useState } from 'react';
import { type Field, QueryBuilder, type RuleGroupType } from 'react-querybuilder';
const fields: Field[] = [
{ name: 'firstName', label: 'First Name' },
{ name: 'lastName', label: 'Last Name' },
];
export function App() {
const [query, setQuery] = useState<RuleGroupType>({ combinator: 'and', rules: [] });
return (
<QueryBuilderAntD>
<QueryBuilder fields={fields} defaultQuery={query} onQueryChange={setQuery} />
</QueryBuilderAntD>
);
}
[!NOTE]
You may also want to reduce the width of the value editor component (which is 100% by default) with the following CSS rule.
.queryBuilder .ant-input { width: auto; }
QueryBuilderAntD
is a React context provider that assigns the following props to all descendant QueryBuilder
elements. The props can be overridden on the QueryBuilder
or used directly without the context provider.
Export | QueryBuilder prop |
---|---|
antdControlElements | controlElements |
antdTranslations | translations |
AntDActionElement | controlElements.actionElement |
AntDDragHandle | controlElements.dragHandle |
AntDNotToggle | controlElements.notToggle |
AntDShiftActions | controlElements.shiftActions |
AntDValueEditor | controlElements.valueEditor |
AntDValueSelector | controlElements.valueSelector |
[!TIP]
By default, this package uses icons from
@ant-design/icons
for button labels. To reset button labels to their default strings, usedefaultTranslations
fromreact-querybuilder
.
<QueryBuilderAntD translations={defaultTranslations}>
[v8.7.1] - 2025-06-09
rqb-base-color
. rqb-background-color
is rqb-base-color
with 20% opacity (effectively the same color as before, just calculated differently).maxLevels
prop to limit the addition of new rule groups beyond the specified nesting level. Default is Infinity
(allows unlimited levels), and values other than 1 or greater will be ignored.FAQs
Custom Ant Design components for react-querybuilder
The npm package @react-querybuilder/antd receives a total of 3,432 weekly downloads. As such, @react-querybuilder/antd popularity was classified as popular.
We found that @react-querybuilder/antd demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.