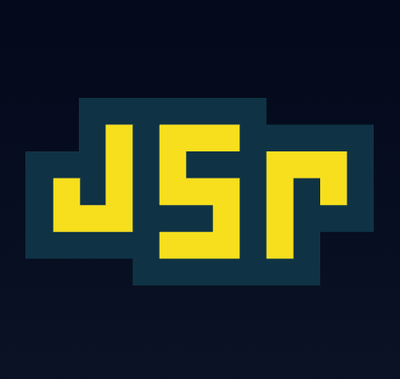
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@salesforce/command
Advanced tools
@salesforce/command is an npm package that provides a framework for building CLI commands for Salesforce. It is designed to help developers create commands that interact with Salesforce APIs and services, making it easier to automate tasks and integrate Salesforce with other systems.
Command Creation
This feature allows developers to create custom CLI commands by extending the SfdxCommand class. The example demonstrates a simple command that logs a message to the console.
const { SfdxCommand } = require('@salesforce/command');
class MyCommand extends SfdxCommand {
async run() {
this.ux.log('Hello, Salesforce!');
}
}
module.exports = MyCommand;
Parameter Handling
This feature provides a way to define and handle command-line parameters using the flags property. The example shows how to define a 'name' parameter and use it within the command.
const { flags, SfdxCommand } = require('@salesforce/command');
class MyCommand extends SfdxCommand {
static flagsConfig = {
name: flags.string({ char: 'n', description: 'name to print' })
};
async run() {
const name = this.flags.name || 'world';
this.ux.log(`Hello, ${name}!`);
}
}
module.exports = MyCommand;
Salesforce Authentication
This feature allows commands to authenticate and interact with Salesforce orgs. The example demonstrates how to create a connection and query Salesforce data.
const { SfdxCommand } = require('@salesforce/command');
const { Connection } = require('@salesforce/core');
class MyCommand extends SfdxCommand {
async run() {
const conn = await Connection.create({ authInfo: this.org.getConnection().getAuthInfo() });
const result = await conn.query('SELECT Id, Name FROM Account');
this.ux.logJson(result.records);
}
}
module.exports = MyCommand;
oclif is a framework for building command-line interfaces in Node.js. It is highly extensible and supports plugins, making it suitable for creating complex CLI applications. Compared to @salesforce/command, oclif is more general-purpose and not specifically tailored for Salesforce.
commander is a popular Node.js library for building command-line interfaces. It provides a simple and flexible API for defining commands and options. While it is not specifically designed for Salesforce, it can be used to create CLI tools for various purposes, including Salesforce automation.
yargs is another widely-used library for building command-line tools in Node.js. It offers a rich set of features for parsing arguments and generating help messages. Like commander, yargs is a general-purpose library and can be used to create CLI tools for different use cases, including Salesforce.
This library is deprecated. You should migrate to https://github.com/salesforcecli/sf-plugins-core.
A migration guide is provided in the sf
wiki https://github.com/salesforcecli/cli/wiki/Migrate-Plugins-Built-For-Sfdx
This package contains the base command class for Salesforce CLI, SfdxCommand
. Extend this class for convenient access to common Salesforce CLI parameters, a logger, CLI output formatting, scratch orgs, and Dev Hubs. This class extends @oclif/command and is available within a plug-in generated by Salesforce Plug-In Generator.
Commands that extend SfdxCommand
can only be used with Salesforce CLI version 6.8.2 or later. To check your Salesforce CLI version:
$ sfdx version
sfdx-cli/6.42.0-ae478b3cb8 (darwin-x64) node-v8.9.4
To learn more about the features of the Command Library see the Salesforce CLI Plug-In Developer Guide.
If you are interested in contributing, please take a look at the CONTRIBUTING guide.
If you are interested in building this package locally, please take a look at the DEVELOPING doc.
SfdxCommand
.Command
, which SfdxCommand
extends.Please report any issues here: https://github.com/forcedotcom/cli/issues
FAQs
Salesforce CLI base command class
The npm package @salesforce/command receives a total of 128,338 weekly downloads. As such, @salesforce/command popularity was classified as popular.
We found that @salesforce/command demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 54 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.