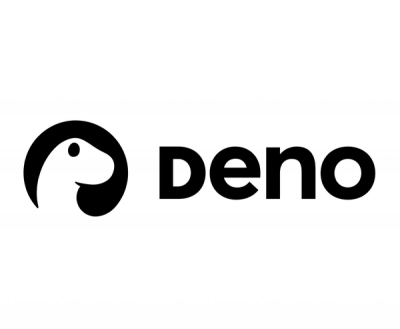
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@sean_kenny/coloured-bitmap-to-geojson-polygons-js
Advanced tools
A library for convering colour segmented bitmaps to a GeoJSON file of polygons.
This is a TypeScript library which contains a function which converts .bmp
bitmap files into a GeoJSON file.
$ npm install --save-dev @sean_kenny/coloured-bitmap-to-geojson-polygons-js
# or
$ yarn add -D @sean_kenny/coloured-bitmap-to-geojson-polygons-js
An output can then be generated by calling the exportColouredBitmapToGeoJSONPolygons
function, which is the only function in the library. colourToPropertiesMap
is an argument which can be used to attach properties (besides colourHexCode
) to regions in your bitmap of a particular colour. Keys in colourToPropertiesMap
must be lowercase (Ex. ff0000
rather than FF0000
).
const { outputGeoJSON, bmpFileMetadata } = await exportColouredBitmapToGeoJSONPolygons({
inputFilePath: '/path/to/your/file/your_file.bmp',
colourToPropertiesMap: {
"#ff0000": {
zone: "The red zone"
},
"#00ff00": {
zone: "The green zone"
},
}
})
bmpFileMetadata
will contain some data in the following format:
{
"header": {
"signature": "BM",
"fileSizeBytes": 120054,
"reservedField": "00000000",
"dataOffsetBytes": 54
},
"infoHeader": {
"bitsPerPixel": "TWENTY_FOUR_BIT_RGB",
"bmpHeightPx": 200,
"bmpWidthPx": 200,
"compressionType": "BI_RGB",
"horizontalPixelsPerMetre": 0,
"imageCompressedSizeBytes": 0,
"infoHeaderSizeBytes": 40,
"numberOfColoursUsed": 0,
"numberOfImportantColours": 0,
"numberOfPlanes": 1,
"verticalPixelsPerMetre": 0
},
"colourMap": {}
}
And outputGeoJSON
will contain some data in the following format:
{
"type": "FeatureCollection",
"features": [
{
"type": "Feature",
"geometry": {
"type": "Polygon",
"coordinates": [
[
[-180, 90],
[-180, -90],
...
]
]
},
"properties": {
"colourHexCode": "#ff0000",
"data": { "zone": "The red zone" }
}
},
{
"type": "Feature",
"geometry": {
"type": "Polygon",
"coordinates": [
[
[-176.4, 90],
[-176.4, 89.1],
...
]
]
},
"properties": {
"colourHexCode": "#00ff00",
"data": { "zone": "The green zone" }
}
},
{
"type": "Feature",
"geometry": {
"type": "Polygon",
"coordinates": [
[
[1.8000000000000114, 1.7999999999999972],
[1.8000000000000114, 0],
...
]
]
},
"properties": {
"colourHexCode": "#0000ff",
"data": null
}
}
]
}
domainBounds
is an additional optional argument to exportColouredBitmapToGeoJSONPolygons
which allows you to specify where the corners of your output GeoJSON file should be on the globe. The default domainBounds
values is { latitudeLowerBound: -90, latitudeUpperBound: 90, longitudeLowerBound: -180, longitudeUpperBound: 180 }
which covers the entire globe. The image below shows the effect of a domainBounds
value of { domainLatitudeLowerBound: -30, domainLatitudeUpperBound: 60, domainLongitudeLowerBound: -20, domainLongitudeUpperBound: 20}
on a .bmp
file.
Original BMP file | Output GeoJSON file |
---|---|
![]() | ![]() |
Original BMP file | Output GeoJSON file |
---|---|
![]() | ![]() |
![]() | ![]() |
There's some interesting reading about the exact structure of a bitmap file here which is what a lot of this library is based on.
All files whose name follows the pattern provinces-XXXXXXX.bmp
which are used for testing are pulled directly from the ab937cf899b75f74662a17574bd0d46072793beb
version of Anbennar which was published to Bitbucket on August 23rd 2024. All credit for those bitmaps goes to their creator. ``bmp-24.bmp` was generously made public by the University of South Carolina.
You're welcome to use this library for anything you'd like. Please keep in mind this hasn't been thoroughly tested with a large variety of bitmap files so this may or may not work for your use case. Please reach out if you have a bitmap file which is not processed properly by this library and I'd be happy to work on something to make it work for you.
FAQs
A library for convering colour segmented bitmaps to a GeoJSON file of polygons.
We found that @sean_kenny/coloured-bitmap-to-geojson-polygons-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.