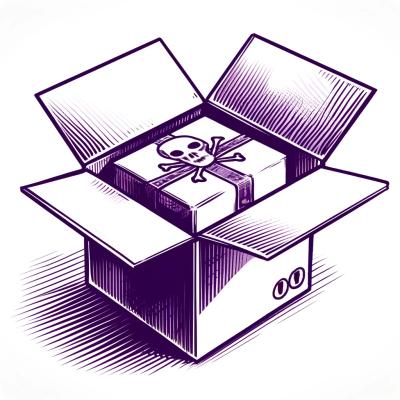
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@segment/sovran-react-native
Advanced tools
A cross-platform lightweight local state management system designed for React Native.
Redux is a great global state management tool for React, but it's not a good fit when you need to have local state management at different scope levels.
The main advantages of Sovran vs Redux are:
npm install @segment/sovran-react-native
To use in JS/TS you just need to use createStore
to create a sovran object.
import { createStore } from '@segment/sovran-react-native';
interface State {
count: number;
}
// Create the sovran store with our type and initial state
const store = createStore<State>({
count: 0,
});
createStore
accepts any object as a state.
You only need to pass the initial state as a parameter.
Returns a Store object:
The returned store object has the following methods:
Subscribes a listener to updates on the store:
const unsubscribe = store.subscribe((newState: State) => {
// do something with the new state
console.log(newState);
});
// When you want to get rid of the listener
unsubscribe();
Dispatches an action to modify the state, actions can be async:
Remember to keep actions as pure functions.
store.dispatch((state: State): State => {
return {
...state,
count: state.count + 1,
}
});
Returns the current state on the store:
const currentState: State = store.getState();
Sovran supports dispatching actions from native to RN. Very useful for brownfield apps.
To use this feature you first need to register which stores will listen to native updates and which actions can be sent from native:
import { createStore, registerBridgeStore } from '@segment/sovran-react-native';
interface MessageQueue {
messages: string[];
}
// Create the sovran store with our type and initial state
const messages = createStore<MessageQueue>({
messages: [],
});
// Action to add new events
const addMessage = (message: Message) => (state: MessageQueue) =>
{ messages: [...state.messages, message] };
// Register the store to listen to native events
registerBridgeStore({
// Sets which store object is listenning
store: messages,
// Defines which action types can be sent from native and how to handle them
actions: {
'add-message': addMessage,
},
});
Now you are ready to send updates from native code!
(Supports both Objective-C and Swift)
@import React;
@import sovran_react_native;
// ...
[Sovran dispatchWithAction:@"add-message" payload:@{ @"origin": @"Native", @"message": @"Hello from Objective-C!" }];
(Supports both Java and Kotlin)
import com.sovranreactnative.Sovran;
public class MainApplication extends Application implements ReactApplication {
private Sovran sovran = new Sovran();
private final ReactNativeHost mReactNativeHost =
new ReactNativeHost(this) {
// Due to how Android registers modules for RN make sure you instantiate the Sovran Module package first and use the same one when you register it in you MainApplication
@Override
protected List<ReactPackage> getPackages() {
@SuppressWarnings("UnnecessaryLocalVariable")
List<ReactPackage> packages = new PackageList(this).getPackages();
// Register the same sovran object
packages.add(sovran);
return packages;
}
// ...
Map<String, String> payload = new HashMap<>();
payload.put("origin", "Native");
payload.put("message", "Hello from Java/Kotlin!");
sovran.dispatch("add-message", payload);
//...
Check out the Example project for a more in depth use case in an RN project!
See the contributing guide to learn how to contribute to the repository and the development workflow.
MIT
FAQs
A cross-platform state management system
The npm package @segment/sovran-react-native receives a total of 64,255 weekly downloads. As such, @segment/sovran-react-native popularity was classified as popular.
We found that @segment/sovran-react-native demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 292 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.