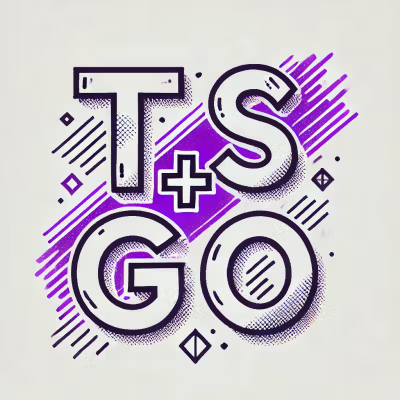
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
@sergiobanhos/whatsapp-api-sdk
Advanced tools
SDK for WhatsApp Business API, Whatsapp Cloud API and Instagram API using Node.js and TypeScript
Here’s a professional and polished README for your WhatsApp SDK, including all the essential sections to guide users:
The WhatsApp & Instagram API SDK is a powerful and easy-to-use Node.js library for interacting with both the official WhatsApp Cloud API and Instagram Graph API. Designed for scalability and ease of use, it supports message sending, webhook integration, interactive buttons, Instagram messaging, comments, and more—all with TypeScript typings for robust development.
Install the SDK using npm:
npm install @sergiobanhos/whatsapp-api-sdk
import { WhatsappCloudApiClient, WhatsappBusinessApiClient } from '@sb/whatsapp-api-sdk';
const { wa, waba } = getWhatsappApi({
whatsapp: {
accessToken: 'your-meta-access-token',
phoneNumberId: 'your-phone-number-id',
whatsappBusinessId: 'your-business-account-id'
}
});
// The wa client is for general WhatsApp Cloud API operations
// The waba client is for WhatsApp Business API operations
import { InstagramApiClient } from '@sb/whatsapp-api-sdk';
const insta = new InstagramApiClient({
token: 'your-instagram-access-token',
phoneNumberId: 'your-instagram-user-id',
onError: (error) => console.error('Error:', JSON.stringify(error, null, 4)),
});
// Using WhatsApp Cloud API
await wa.sendTextMessage('<CUSTOMER_PHONE_NUMBER>', 'Hello from WhatsApp API SDK!');
// Using Instagram API
await insta.sendMessage('<INSTAGRAM_USER_ID>', 'Hello from Instagram API SDK!');
await client.sendButtonMessage('<CUSTOMER_PHONE_NUMBER>', {
body: 'Choose an option:',
buttons: [
{ type: 'reply', id: '1', title: 'Option 1' },
{ type: 'reply', id: '2', title: 'Option 2' },
],
});
await client.sendCTAMessage('<CUSTOMER_PHONE_NUMBER>', {
header: { type: 'text', text: 'Header Text' },
body: 'Visit our website:',
footer: 'Footer Text',
buttons: [
{ type: 'url', url: 'https://example.com', title: 'Go to Website' },
],
});
The SDK provides a built-in webhook handler using Express:
import express from 'express';
import WhatsAppClient from '@sb/whatsapp-api-sdk';
const app = express();
const client = new WhatsAppClient({
accessToken: 'your-meta-access-token',
verifyToken: 'your-webhook-verify-token',
});
client.setupWebhookRoutes(app);
app.listen(3000, () => {
console.log('Webhook server running on port 3000');
});
Option | Type | Description |
---|---|---|
accessToken | string | Your Meta API access token. |
verifyToken | string | Token used for webhook validation. |
onError | Function | Callback for handling errors from the WhatsApp Cloud API. |
webhookCallback | Function | Callback triggered when webhook events are received. |
Method | Description |
---|---|
sendTextMessage | Sends a simple text message. |
sendButtonMessage | Sends a message with interactive buttons. |
sendCTAMessage | Sends a Call-to-Action message with links. |
setupWebhookRoutes | Sets up webhook validation and POST routes. |
| Method | Description |
|-----------------------|------------------------------------------------------||
| sendMessage
| Sends a message to an Instagram user |
| getComments
| Retrieves comments from an Instagram post |
| replyToComment
| Replies to a specific Instagram comment |
| getUserProfile
| Gets information about an Instagram user |
| getPost
| Retrieves information about an Instagram post |
To test the library locally, follow these steps:
git clone https://github.com/sergipe085/whatsapp-api-sdk.git
npm install
npm test
This project is licensed under the MIT License.
For any questions or issues, please open an issue or contact us at sergiobanhosf@gmail.com.
Special thanks to the WhatsApp Cloud API team and the open-source community for their support and documentation.
Let me know if you'd like to add custom badges, extended documentation, or other sections!
FAQs
SDK for WhatsApp Business API, Whatsapp Cloud API and Instagram API using Node.js and TypeScript
The npm package @sergiobanhos/whatsapp-api-sdk receives a total of 40 weekly downloads. As such, @sergiobanhos/whatsapp-api-sdk popularity was classified as not popular.
We found that @sergiobanhos/whatsapp-api-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.