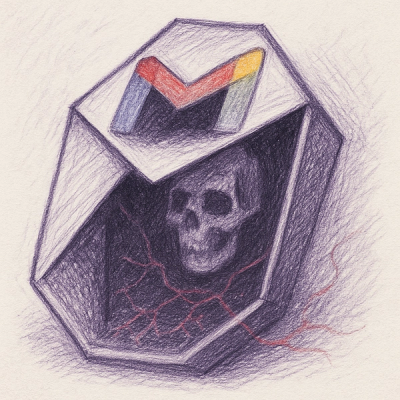
Research
Using Trusted Protocols Against You: Gmail as a C2 Mechanism
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.
@sotatech/query3
Advanced tools
@sotatech/query3
A powerful and flexible query handler for MongoDB models using Mongoose.
@sotatech/query3
simplifies handling advanced queries, pagination, filtering, and aggregation pipelines in a clean and type-safe manner.
populate
for relational data.npm install @sotatech/query3
Import Query3
and integrate it with your Mongoose model.
import { Query3 } from '@sotatech/query3';
import { UserModel } from './models/user.model'; // Your Mongoose model
import qs from 'qs';
const queryString = qs.stringify({
limit: 10,
offset: 0,
sort: { age: -1 },
age: { $gte: 18 },
});
const query = new Query3(UserModel);
const result = await query.query(queryString, {
populate: [{ path: 'role', select: 'name' }],
omitFields: ['password'], // Exclude sensitive fields
allowedOperators: ['$gte', '$lte', '$eq'], // Restrict allowed operators
});
console.log(result);
/**
* {
* records: [
* { name: 'John Doe', age: 30, role: { name: 'Admin' } },
* { name: 'Jane Doe', age: 25, role: { name: 'User' } }
* ],
* pagination: {
* totalRows: 50,
* totalPages: 5
* }
* }
*/
new Query3<T>(model: Model<T>)
T
: The type of the Mongoose model being queried.model
: The Mongoose model instance.const query = new Query3(UserModel);
query(queryString: string, options?: Query3Options<T>)
Executes a query on the provided model.
queryString
: A JSON string representing the query parameters (e.g., filter
, limit
, offset
, etc.).options
(optional): Custom options for the query.Query3Options<T>
Option | Type | Description |
---|---|---|
populate | PopulateOptions or Array<PopulateOptions> | Defines relationships to populate. |
omitFields | Array<keyof T> | Fields to omit from the results. |
queryMongoose | FilterQuery<T> | Additional Mongoose filters to apply. |
allowedOperators | Array<string> | Restrict allowed MongoDB operators. |
const result = await query.query(queryString, {
populate: [{ path: 'role', select: 'name' }],
omitFields: ['password'],
queryMongoose: { isActive: true },
allowedOperators: ['$gte', '$lte'],
});
aggregateQuery(pipeline: PipelineStage[]): Promise<any[]>
Executes an aggregation pipeline on the model.
pipeline
: An array of MongoDB aggregation stages.const pipeline = [
{ $match: { age: { $gte: 18 } } },
{ $group: { _id: '$role', count: { $sum: 1 } } },
];
const result = await query.aggregateQuery(pipeline);
console.log(result);
/**
* [
* { _id: 'admin', count: 5 },
* { _id: 'user', count: 10 }
* ]
*/
omitFields(data: T[], fields: (keyof T)[]): T[]
Removes specified fields from the result set.
data
: The array of documents to process.fields
: The list of fields to remove.const data = [
{ name: 'John Doe', password: '123456', age: 30 },
{ name: 'Jane Doe', password: 'abcdef', age: 25 },
];
const result = query.omitFields(data, ['password']);
console.log(result);
/**
* [
* { name: 'John Doe', age: 30 },
* { name: 'Jane Doe', age: 25 }
* ]
*/
The queryString
parameter accepts a JSON string with the following properties:
Property | Type | Description |
---|---|---|
filter | object | MongoDB query filters. |
limit | number (default: 20) | Maximum number of records to return. |
offset | number (default: 0) | Number of records to skip (for pagination). |
sort | object | Sorting configuration (e.g., { age: -1 } ). |
{
"limit": 10,
"offset": 0,
"sort": { "createdAt": -1 },
"age": { "$gte": 18 }
}
If an unsupported operator is used, an error will be thrown.
const queryString = qs.stringify({
age: { $invalidOperator: 30 },
});
try {
await query.query(queryString, { allowedOperators: ['$gte', '$lte'] });
} catch (error) {
console.error(error.message); // "Operator '$invalidOperator' is not allowed."
}
Contributions are welcome! To contribute:
feature/your-feature
).This project is licensed under the MIT License.
For issues or questions, please open an issue on GitHub.
Happy Coding! 🚀
FAQs
A flexible query handler for MongoDB using Mongoose
The npm package @sotatech/query3 receives a total of 4 weekly downloads. As such, @sotatech/query3 popularity was classified as not popular.
We found that @sotatech/query3 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.
Product
We redesigned Socket's first logged-in page to display rich and insightful visualizations about your repositories protected against supply chain threats.
Product
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.