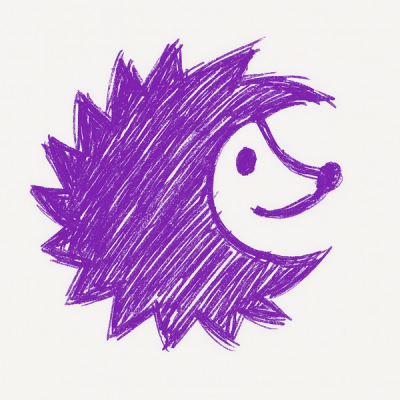
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
@splunkdev/cloud-auth
Advanced tools
DEPRECATED - Library to help authenticating web applications in Splunk Cloud.
The below documentation has been preserved for posterity.
$ yarn add @splunkdev/cloud-auth
# or
$ npm install --save @splunkdev/cloud-auth
"auth": {
"clientId": "your_client_id",
}
import AuthClient from '@splunkdev/cloud-auth/AuthClient';
import { auth as authConfig } from '../config/config.json';
export default new AuthClient({
...authConfig,
redirectUri: window.location.origin, // eslint-disable-line
});
// ...
import React, { Component } from 'react';
class App extends Component {
state = {
loggedIn: false,
error: null,
};
componentDidMount() {
this.authenticate();
}
authenticate = async () => {
try {
// authClient will redirect to login page if user is not authenticated.
const loggedIn = await authClient.checkAuthentication();
this.setState({
loggedIn,
});
} catch (e) {
this.setState({
loggedIn: false,
error: e.message,
});
}
};
render() {
const { error, loggedIn } = this.state;
if (error) {
return <div>Error: {error}</div>;
}
if (!loggedIn) {
return <div>Loading...</div>;
}
return <div>My App...</div>;
}
}
Configuration Options for AuthClient
:
{
// Client ID is used to identify the app registred with the App Registry
clientId: "...", // required
// The redirect URI is used to redirect the user back to the web app after
// login. This redirectUri must be registered with the App Registry
redirectUri: window.location.origin, // required
// If enabled, then the @splunkdev/cloud-auth lib will restore the path of the web app
// after redirecting to login page
restorePathAfterLogin: true,
// This function is called (if provided) when the user was redirected back
// from login, after the auth callback was successfully applied.
// This function can be used to integrate with third-party client-side
// routers, such as react-router intead of calling `history.replaceState`.
onRestorePath: function(path) { /* ... */ },
// If enabled, the user is automatically redirected to login page when
// the AuthClient instance is created or when checkAuthentication is called
// and the user is no already logged in.
// This is enabled by default but can be disabled by setting it to `false`.
autoRedirectToLogin: true,
// The url that is redirected to when using token.getWithRedirect.
// This must be pre-registered as part of client registration. If no redirectUri is provided, defaults to the current origin.
redirectUri: "...",
// authorizeUrl to perform the authorization flow. Defaults to Splunk authorize server.
authorizeUrl: "..."
// maxClockSkew specifies the duration buffer in seconds for token expiration
// (now > actualExpiration - maxClockSkew) will be considered expired
//
// Default value is 600
maxClockSkew: 600
// autoTokenRenewalBuffer specifies the duration buffer in seconds for token auto renewal.
// (now > actualExpiration - autoTokenRenewalBuffer) will trigger an auto renewal
//
// Default value is 120
autoTokenRenewalBuffer: 120
}
FAQs
DEPRECATED - Library to help authenticating web applications in Splunk Cloud.
We found that @splunkdev/cloud-auth demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.