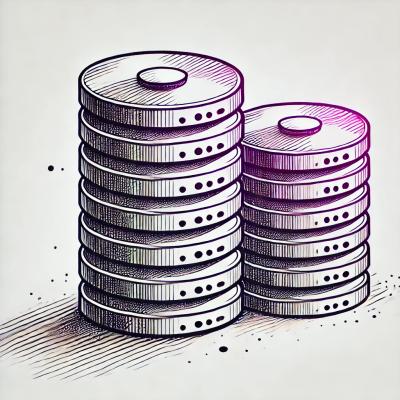
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
@starryinternet/map-memo
Advanced tools
Generic memoization using Map
and WeakMap
.
npm install --save @starryinternet/map-memo
Memoization in JavaScript has typically been limited to arguments with primitive values, or by utilizing hacks such as stringifying objects.
By storing arguments using a series of nested cache objects backed by Map
and WeakMap
, map-memo
is able to memoize functions with any
argument types.
'use strict';
const memoize = require('@starryinternet/map-memo');
function loop( fn, n ) {
let v;
for ( let i = 0; i < n; ++i ) {
v = fn( i );
}
return v;
}
let mem = memoize( loop );
console.log( mem( Math.sqrt, 1e9 ) ); // slow
console.log( mem( Math.sqrt, 1e9 ) ); // fast!
'use strict';
const memoize = require('@starryinternet/map-memo');
function getRandom() {
return Math.random();
}
let mem = memoize( getRandom, { ttl: 1000 } );
console.log( mem() );
console.log( mem() ); // Same value as above
setTimeout( function() {
console.log( mem() ); // Different value
}, 1001 );
'use strict';
const memoize = require('@starryinternet/map-memo');
function loopAsync( fn, n ) {
return new Promise( ( resolve, reject ) => {
let v;
for ( let i = 0; i < n; ++i ) {
v = fn( i );
}
resolve( v );
});
}
let mem = memoize( loopAsync );
mem( Math.sqrt, 1e9 ).then( result => {
console.log( result ); // slow
mem( Math.sqrt, 1e9 ).then( console.log ); // fast!
});
FAQs
Generic memoization with Map and WeakMap
We found that @starryinternet/map-memo demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.