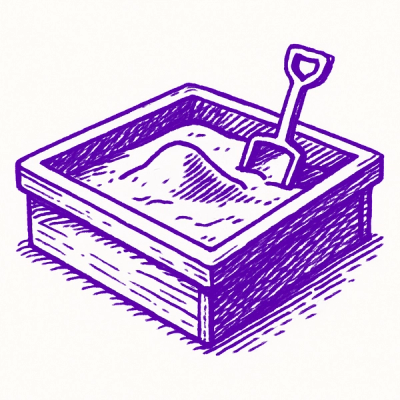
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
@stdlib/string-format
Advanced tools
We believe in a future in which the web is a preferred environment for numerical computation. To help realize this future, we've built stdlib. stdlib is a standard library, with an emphasis on numerical and scientific computation, written in JavaScript (and C) for execution in browsers and in Node.js.
The library is fully decomposable, being architected in such a way that you can swap out and mix and match APIs and functionality to cater to your exact preferences and use cases.
When you use stdlib, you can be absolutely certain that you are using the most thorough, rigorous, well-written, studied, documented, tested, measured, and high-quality code out there.
To join us in bringing numerical computing to the web, get started by checking us out on GitHub, and please consider financially supporting stdlib. We greatly appreciate your continued support!
Insert supplied variable values into a format string.
npm install @stdlib/string-format
var format = require( '@stdlib/string-format' );
Inserts supplied variable values into a format string.
var str = 'Hello, %s! My name is %s.';
var out = format( str, 'world', 'Bob' );
// returns 'Hello, world! My name is Bob.'
The format string is a string literal containing zero or more conversion specifications, each of which results in a string value being inserted to the output string. A conversion specification consists of a percent sign (%
) followed by one or more of the following flags, width, precision, and conversion type characters. It thus takes the following form:
%[flags][width][.precision]specifier
Arguments following the format string are used to replace the placeholders in the format string. The number of arguments following the format string should be equal to the number of placeholders in the format string.
var str = '%s %s';
var out = format( str, 'Hello', 'World' );
// returns 'Hello World'
To supply arguments in a different order than they appear in the format string, positional placeholders as indicated by a $
character in the format string are used. In this case, the conversion specification takes the form:
%[pos$][flags][width][.precision]specifier
var str = '%3$s %2$s %1$s';
var out = format( str, 'foo', 'bar', 'baz' );
// returns 'baz bar foo'
The following table summarizes the supported specifiers:
type | description | example |
---|---|---|
s | string | beep boop |
c | character | a |
d, i | signed decimal integer | -12 |
u | unsigned decimal integer | 390 |
b | unsigned binary integer | 11011011 |
o | unsigned octal integer | 510 |
x | unsigned hexadecimal (lowercase) | 7b |
X | unsigned hexadecimal (uppercase) | 7B |
f, F | decimal floating point | 390.24 |
e | scientific notation (lowercase) | 3.9e+1 |
E | scientific notation (uppercase) | 3.9E+1 |
g | shortest representation (e /f ) | 3.9 |
G | shortest representation (E /F ) | 3.9 |
var str = '%i written as a binary number is %b.';
var out = format( str, 9, 9 );
// returns '9 written as a binary number is 1001.'
str = '%i written as an octal number is %o.';
out = format( str, 17, 17 );
// returns '17 written as an octal number is 21.'
str = '%i written as a hexadecimal number is %x.';
out = format( str, 255, 255 );
// returns '255 written as a hexadecimal number is ff.'
str = '%i written as a hexadecimal number is %X (uppercase letters).';
out = format( str, 255, 255 );
// returns '255 written as a hexadecimal number is FF (uppercase letters).'
str = '%i written as a floating point number with default precision is %f!';
out = format( str, 8, 8 );
// returns '8 written as a floating point number with default precision is 8.000000!'
str = 'Scientific notation: %e';
out = format( str, 3.14159 );
// returns 'Scientific notation: 3.141590e+00'
str = 'Scientific notation: %E (uppercase).';
out = format( str, 3.14159 );
// returns 'Scientific notation: 3.141590E+00 (uppercase).'
str = '%g (shortest representation)';
out = format( str, 3.14159 );
// returns '3.14159'
A conversion specification may contain zero or more flags, which modify the behavior of the conversion. The following flags are supported:
flag | description |
---|---|
- | left-justify the output within the given field width by padding with spaces on the right |
0 | left-pad the output with zeros instead of spaces when padding is required |
# | use an alternative format for o and x conversions |
+ | prefix the output with a plus (+) or minus (-) sign even if the value is a positive number |
space | prefix the value with a space character if no sign is written |
var str = 'Always prefix with a sign: %+i';
var out = format( str, 9 );
// returns 'Always prefix with a sign: +9'
out = format( str, -9 );
// returns 'Always prefix with a sign: -9'
str = 'Only prefix with a sign if negative: %i';
out = format( str, 6 );
// returns 'Only prefix with a sign if negative: 6'
out = format( str, -6 );
// returns 'Only prefix with a sign if negative: -6'
str = 'Prefix with a sign if negative and a space if positive: % i';
out = format( str, 3 );
// returns 'Prefix with a sign if negative and a space if positive: 3'
out = format( str, -3 );
// returns 'Prefix with a sign if negative and a space if positive: -3'
The width
may be specified as a decimal integer representing the minimum number of characters to be written to the output. If the value to be written is shorter than this number, the result is padded with spaces on the left. The value is not truncated even if the result is larger. Alternatively, the width
may be specified as an asterisk character (*
), in which case the argument preceding the conversion specification is used as the minimum field width.
var str = '%5s';
var out = format( str, 'baz' );
// returns ' baz'
str = '%-5s';
out = format( str, 'baz' );
// returns 'baz '
str = '%05i';
out = format( str, 2 );
// returns '00002'
str = '%*i';
out = format( str, 5, 2 );
// returns ' 2'
The precision
may be specified as a decimal integer or as an asterisk character (*
), in which case the argument preceding the conversion specification is used as the precision value. The behavior of the precision
differs depending on the conversion type:
s
specifiers, the precision
specifies the maximum number of characters to be written to the output.f
, F
, e
, E
), the precision
specifies the number of digits after the decimal point to be written to the output (by default, this is 6
).g
and G
specifiers, the precision
specifies the maximum number of significant digits to be written to the output.d
, i
, u
, b
, o
, x
, X
), the precision
specifies the minimum number of digits to be written to the output. If the value to be written is shorter than this number, the result is padded with zeros on the left. The value is not truncated even if the result is longer. ForAlternatively, the precision
may be specified as an asterisk character (*
), in which case the argument preceding the conversion specification is used as the minimum number of digits.
var str = '%5.2s';
var out = format( str, 'baz' );
// returns ' ba'
str = 'PI: ~%.2f';
out = format( str, 3.14159 );
// returns 'PI: ~3.14'
str = 'Agent %.3i';
out = format( str, 7 );
// returns 'Agent 007'
var format = require( '@stdlib/string-format' );
var out = format( '%s %s!', 'Hello', 'World' );
// returns 'Hello World!'
out = format( 'Pi: ~%.2f', 3.141592653589793 );
// returns 'Pi: ~3.14'
out = format( '%-10s %-10s', 'a', 'b' );
// returns 'a b '
out = format( '%10s %10s', 'a', 'b' );
// returns ' a b'
out = format( '%2$s %1$s %3$s', 'b', 'a', 'c' );
// returns 'a b c'
This package is part of stdlib, a standard library for JavaScript and Node.js, with an emphasis on numerical and scientific computing. The library provides a collection of robust, high performance libraries for mathematics, statistics, streams, utilities, and more.
For more information on the project, filing bug reports and feature requests, and guidance on how to develop stdlib, see the main project repository.
See LICENSE.
Copyright © 2016-2024. The Stdlib Authors.
0.2.2 (2024-07-26)
No changes reported for this release.
</section> <!-- /.release --> <section class="release" id="v0.2.1">FAQs
Insert supplied variable values into a format string.
We found that @stdlib/string-format demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.