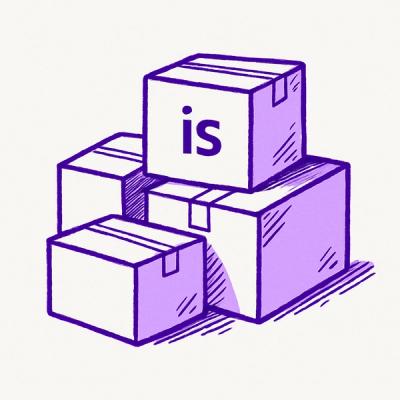
Security News
npm ‘is’ Package Hijacked in Expanding Supply Chain Attack
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
@storybook/react-simple-di
Advanced tools
Simple dependancy injection solution for React.
npm i react-simple-di
In react-simple-di
, we've two types of dependencies, they are:
Every action will receive the
context
as it's first argument.
First, we need to inject dependencies to a root level React component. Mostly, this will be the main layout component of our app.
Here are our dependencies:
const context = {
DB,
Router,
appName: 'My Blog'
};
const actions = {
posts: {
create({DB, Router}, title, content) {
const id = String(Math.random());
DB.createPost(id, title, content);
Router.go(`/post/${id}`);
}
}
};
First we've defined our context. Then, we have our actions. Here actions must follow a structure like mentioned above.
Let's inject our dependencies:
import {injectDeps} from 'react-simple-di';
import Layout from './layout.jsx';
// Above mentioned actions and context are defined here.
const LayoutWithDeps = injectDeps(context, actions)(Layout);
Now you can use LayoutWithDeps
anywhere in your app.
Any component rendered inside LayoutWithDeps
can access both context and actions.
When using dependecies it will compose a new React component and pass dependencies via props to the original component.
First let's create our UI component. Here it will expect dependecies to come via props appName
and createPost
.
class CreatePost extends React.Component {
render() {
const {appName} = this.props;
return (
<div>
Create a blog post on app: ${appName}. <br/>
<button onClick={this.create.bind(this)}>Create Now</button>
</div>
);
}
create() {
const {createPost} = this.props;
createPost('My Blog Title', 'Some Content');
}
}
So, let's use dependencies:
import {useDeps} from 'react-simple-di';
// Assume above mentioned CreatePost react component is
// defined here.
const depsToPropsMapper = (context, actions) => ({
appName: context.appName,
createPost: actions.posts.create
});
const CreatePostWithDeps = useDeps(depsToPropsMapper)(CreatePost);
That's it.
Note: Here when calling the
actions.posts.create
action, you don't need to provide the context as the first argument. It'll handle byreact-simple-di
.
Default Mapper
If you didn't provide a mapper function, useDeps will use a default mapper function will allows you to get context and props directy. Here's that default mapper:
const mapper = (context, actions) => ({
context: () => context,
actions: () => actions
});
FAQs
Simple Dependancy Injection Solution for React
The npm package @storybook/react-simple-di receives a total of 29,506 weekly downloads. As such, @storybook/react-simple-di popularity was classified as popular.
We found that @storybook/react-simple-di demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 11 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
Security News
A critical flaw in the popular npm form-data package could allow HTTP parameter pollution, affecting millions of projects until patched versions are adopted.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.