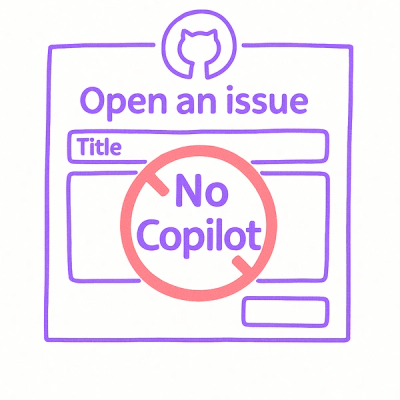
Security News
Open Source Maintainers Demand Ability to Block Copilot-Generated Issues and PRs
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
@stytch/vanilla-js
Advanced tools
npm install @stytch/vanilla-js
The vanilla Stytch Javascript SDK is built on open web standards and is compatible with all Javascript frameworks.
StytchUIClient
The Stytch UI Client provides methods for interacting with the Stytch API from a browser environment, along with prebuilt UI components such as our login form.
import { StytchUIClient } from '@stytch/vanilla-js';
const stytch = new StytchUIClient('public-token-test-xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx');
// Call Stytch APIs from the browser
stytch.magicLinks.email.loginOrCreate('charles.babbage@example.com');
// Render prebuilt UI
stytch.mountLogin({
elementId: '#magic-link',
config: {
products: ['emailMagicLinks', 'oauth'],
emailMagicLinksOptions: {
loginRedirectURL: 'https://example.com/authenticate',
loginExpirationMinutes: 30,
signupRedirectURL: 'https://example.com/authenticate',
signupExpirationMinutes: 30,
createUserAsPending: true,
},
oauthOptions: {
providers: [{ type: 'google' }, { type: 'microsoft' }, { type: 'apple' }],
loginRedirectURL: 'https://example.com/authenticate',
signupRedirectURL: 'https://example.com/authenticate',
},
},
});
StytchHeadlessClient
Developers that don't use Stytch UI elements can use the StytchHeadlessClient
instead, which is significantly smaller!
import { StytchHeadlessClient } from '@stytch/vanilla-js/headless';
const stytch = new StytchHeadlessClient('public-token-test-xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx');
// Call Stytch APIs from the browser
stytch.magicLinks.email.loginOrCreate('charles.babbage@example.com');
StytchB2BUIClient
The Stytch UI Client provides methods for interacting with the Stytch API from a browser environment, along with prebuilt UI components such as our login form.
import { StytchB2BUIClient } from '@stytch/vanilla-js/b2b';
const stytch = new StytchB2BUIClient('public-token-test-xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx');
// Call Stytch APIs from the browser
stytch.magicLinks.email.loginOrSignup({
email_address: 'charles.babbage@example.com',
organization_id: 'organization-test-07971b06-ac8b-4cdb-9c15-63b17e653931',
});
// Render prebuilt UI
stytch.mount({
elementId: '#magic-link',
config: {
authFlowType: 'Discovery',
products: ['emailMagicLinks', 'oauth'],
emailMagicLinksOptions: {
loginRedirectURL: 'https://example.com/authenticate',
signupRedirectURL: 'https://example.com/authenticate',
},
oauthOptions: {
providers: [{ type: 'google' }, { type: 'microsoft' }],
loginRedirectURL: 'https://example.com/authenticate',
signupRedirectURL: 'https://example.com/authenticate',
},
sessionOptions: {
sessionDurationMinutes: 60,
},
},
});
StytchB2BHeadlessClient
Developers that don't use Stytch UI elements can use the StytchB2BHeadlessClient
instead, which is significantly smaller!
import { StytchB2BHeadlessClient } from '@stytch/vanilla-js/b2b/headless';
const stytch = new StytchB2BHeadlessClient('public-token-test-xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx');
// Call Stytch APIs from the browser
stytch.magicLinks.email.loginOrSignup({
email_address: 'charles.babbage@example.com',
organization_id: 'organization-test-07971b06-ac8b-4cdb-9c15-63b17e653931',
});
For more information on how to use the Stytch SDK, please refer to the docs.
FAQs
Stytch's official Javascript Client Library
The npm package @stytch/vanilla-js receives a total of 24,902 weekly downloads. As such, @stytch/vanilla-js popularity was classified as popular.
We found that @stytch/vanilla-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 18 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
Research
Security News
Malicious Koishi plugin silently exfiltrates messages with hex strings to a hardcoded QQ account, exposing secrets in chatbots across platforms.
Research
Security News
Malicious PyPI checkers validate stolen emails against TikTok and Instagram APIs, enabling targeted account attacks and dark web credential sales.