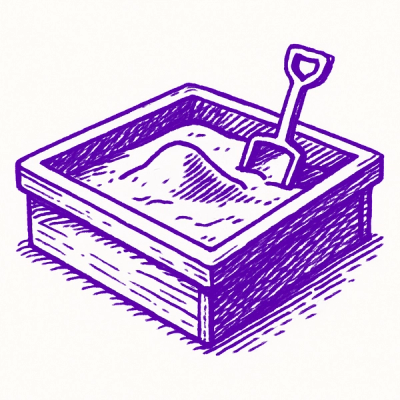
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
@supabase/auth-js
Advanced tools
@supabase/auth-js is a JavaScript library that provides authentication functionalities for Supabase, an open-source Firebase alternative. It allows developers to manage user authentication, including sign-up, sign-in, password recovery, and social logins, among other features.
Sign Up
This feature allows users to sign up for an account using their email and password. The code sample demonstrates how to use the `signUp` method to create a new user.
const { createClient } = require('@supabase/supabase-js');
const supabase = createClient('https://your-project.supabase.co', 'public-anon-key');
async function signUp(email, password) {
const { user, error } = await supabase.auth.signUp({
email: email,
password: password
});
if (error) console.error('Error signing up:', error);
else console.log('User signed up:', user);
}
signUp('user@example.com', 'password123');
Sign In
This feature allows users to sign in to their account using their email and password. The code sample demonstrates how to use the `signIn` method to authenticate a user.
const { createClient } = require('@supabase/supabase-js');
const supabase = createClient('https://your-project.supabase.co', 'public-anon-key');
async function signIn(email, password) {
const { user, error } = await supabase.auth.signIn({
email: email,
password: password
});
if (error) console.error('Error signing in:', error);
else console.log('User signed in:', user);
}
signIn('user@example.com', 'password123');
Password Recovery
This feature allows users to recover their password by sending a password recovery email. The code sample demonstrates how to use the `resetPasswordForEmail` method to initiate the password recovery process.
const { createClient } = require('@supabase/supabase-js');
const supabase = createClient('https://your-project.supabase.co', 'public-anon-key');
async function resetPassword(email) {
const { data, error } = await supabase.auth.api.resetPasswordForEmail(email);
if (error) console.error('Error sending password recovery email:', error);
else console.log('Password recovery email sent:', data);
}
resetPassword('user@example.com');
Social Logins
This feature allows users to sign in using social login providers like GitHub, Google, etc. The code sample demonstrates how to use the `signIn` method with a provider to authenticate a user via social login.
const { createClient } = require('@supabase/supabase-js');
const supabase = createClient('https://your-project.supabase.co', 'public-anon-key');
async function signInWithProvider(provider) {
const { user, session, error } = await supabase.auth.signIn({
provider: provider
});
if (error) console.error('Error signing in with provider:', error);
else console.log('User signed in with provider:', user);
}
signInWithProvider('github');
Firebase is a comprehensive app development platform that includes authentication services. It supports email/password authentication, social logins, and more. Compared to @supabase/auth-js, Firebase offers a broader range of services beyond authentication, such as real-time databases, cloud functions, and analytics.
Auth0 is a flexible, drop-in solution to add authentication and authorization services to your applications. It supports various authentication methods, including social logins, enterprise logins, and multi-factor authentication. Compared to @supabase/auth-js, Auth0 provides more advanced features and integrations but can be more complex to set up and manage.
NextAuth.js is a complete open-source authentication solution for Next.js applications. It supports various authentication methods, including email/password, OAuth providers, and more. Compared to @supabase/auth-js, NextAuth.js is specifically designed for Next.js and offers seamless integration with it, while @supabase/auth-js can be used with any JavaScript framework.
auth-js
An isomorphic JavaScript client library for the Supabase Auth API.
auth-js
: https://supabase.com/docs/reference/javascript/auth-signupInstall
npm install --save @supabase/auth-js
Usage
import { AuthClient } from '@supabase/auth-js'
const GOTRUE_URL = 'http://localhost:9999'
const auth = new AuthClient({ url: GOTRUE_URL })
signUp()
: https://supabase.io/docs/reference/javascript/auth-signupsignIn()
: https://supabase.io/docs/reference/javascript/auth-signinsignOut()
: https://supabase.io/docs/reference/javascript/auth-signoutfetch
implementationauth-js
uses the cross-fetch
library to make HTTP requests, but an alternative fetch
implementation can be provided as an option. This is most useful in environments where cross-fetch
is not compatible, for instance Cloudflare Workers:
import { AuthClient } from '@supabase/auth-js'
const AUTH_URL = 'http://localhost:9999'
const auth = new AuthClient({ url: AUTH_URL, fetch: fetch })
We are building the features of Firebase using enterprise-grade, open source products. We support existing communities wherever possible, and if the products don’t exist we build them and open source them ourselves.
FAQs
Official client library for Supabase Auth
The npm package @supabase/auth-js receives a total of 2,765,957 weekly downloads. As such, @supabase/auth-js popularity was classified as popular.
We found that @supabase/auth-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 14 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.