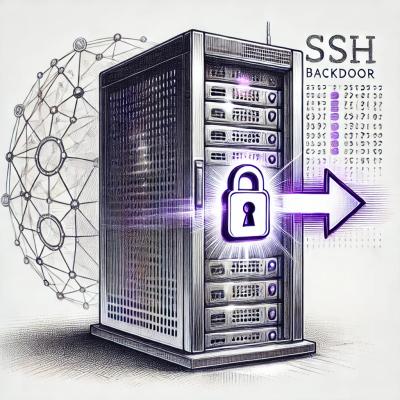
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@synqly/connect-react-sdk
Advanced tools
The Synqly Connect React SDK provides convenient hooks for managing integrations using Synqly Connect.
The Synqly Connect React SDK provides convenient hooks for managing integrations using Synqly Connect.
To use this SDK you must have access to a Synqly organization. If you aren't yet a Synqly customer and like to know more, please contact us to schedule a demo.
Use your favorite package manager to install @synqly/connect-react-sdk
:
npm install @synqly/connect-react-sdk
The Synqly Connect React SDK consists of a single hook:
useIntegrationPoint
: high-level hook used to interface with Synqly
Connect for managing integrations and providing utilities for embedding
Synqly Connect into your application[!TIP] For examples on how to use this library in an application, please see: https://github.com/Synqly/examples/tree/main/connect
In order to use this hook, you will need to provide an organization access token. More information about authentication is available in the Synqly API documentation.
useIntegrationPoint
This high-level hook provides you with all relevant data for a given integration, as well as functions to open a Connect session for your end-users to manage their integrations.
To use this hook you must provide the id
or name
of an integration
point, as well as a token
with relevant access.
[!CAUTION] The
token
should be limited in scope as much as possible, so that it's appropriate for use in an end-user browser environment. To help with this, please review the Token Exchange API. We recommend using theconnect-ui
permission set when working with Connect UI.
Example:
function NotificationsIntegrationCard({ accountId, token }) {
const integrationPoint = useIntegrationPoint('app-notifications', token, {
account: accountId,
})
const {
integrations: [integration],
connect,
disconnect,
isLoading,
} = integrationPoint
const handleClick = useCallback(() => {
return integration ? disconnect() : connect({ type: 'tab' })
}, [connect, disconnect])
return (
<div className="integration-card">
<h1>Application Notifications</h1>
<p>
Integrate with your favorite notifications provider to receive updates
from our app!
</p>
<button type="button" disabled={isLoading} onClick={handleClick}>
{integration ? 'Disconnect' : 'Connect'}
</button>
</div>
)
}
[!TIP] The hooks use smart data fetching and will by default revalidate the data as needed. The default settings can be overridden for greater control over the data fetching process.
FAQs
The Synqly Connect React SDK provides convenient hooks for managing integrations using Synqly Connect.
The npm package @synqly/connect-react-sdk receives a total of 139 weekly downloads. As such, @synqly/connect-react-sdk popularity was classified as not popular.
We found that @synqly/connect-react-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.