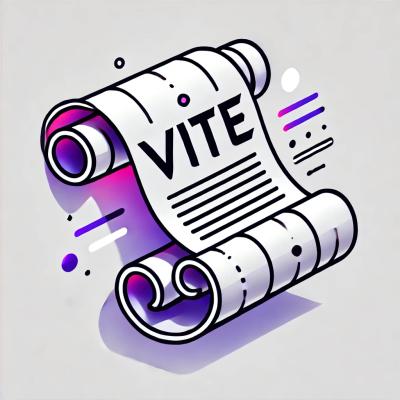
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
@tambo-ai/react
Advanced tools
Build AI assistants and agents in React with a few lines of code.
tambo ai is a React library that deals with the boring parts. Get started with an AI assistant in minutes.
We handle:
You get clean React hooks that integrate seamlessly with your codebase.
npm install @tambo-ai/react
Use our template:
git clone https://github.com/tambo-ai/tambo-template.git && cd tambo-template && npm install && npx tambo init
npm run dev
or try adding it to your existing project:
npx tambo full-send
import { TamboProvider } from "@tambo-ai/react";
function App() {
return (
<TamboProvider apiKey="your-api-key">
<YourApp />
</TamboProvider>
);
}
import { useTambo, useTamboThreadInput } from "@tambo-ai/react";
function ChatInterface() {
const { thread, sendThreadMessage } = useTambo();
const { value, setValue, submit } = useTamboThreadInput();
return (
<div>
{/* Display messages */}
<div>
{thread.messages.map((message, index) => (
<div key={index} className={`message ${message.role}`}>
<div>{message.content}</div>
{message.component && message.component.renderedComponent}
</div>
))}
</div>
{/* Input form */}
<form
onSubmit={(e) => {
e.preventDefault();
submit();
}}
className="input-form"
>
<input
type="text"
value={value}
onChange={(e) => setValue(e.target.value)}
placeholder="Type your message..."
/>
<button type="submit">Send</button>
</form>
</div>
);
}
Specialized hooks for specific needs:
useTambo
- Primary entrypoint for the Tambo React SDKuseTamboThreadInput
- Input state and submissionuseTamboSuggestions
- AI-powered message suggestionsuseTamboThreadList
- Conversation managementuseTamboComponentState
- AI-generated component stateuseTamboThread
- Access to current thread contextComponent registration for AI-generated UI
Tool integration for your data sources
Streaming responses for real-time interactions
Define which components your AI assistant can use to respond to users by passing them to the TamboProvider:
import { TamboProvider } from "@tambo-ai/react";
import { WeatherCard } from "./components/WeatherCard";
import { z } from "zod";
// Define your components
const components = [
{
name: "WeatherCard",
description: "A component that displays weather information",
component: WeatherCard,
propsSchema: z.object({
temperature: z.number(),
condition: z.string(),
location: z.string(),
}),
},
];
// Pass them to the provider
function App() {
return (
<TamboProvider apiKey="your-api-key" components={components}>
<YourApp />
</TamboProvider>
);
}
You can also use z.describe()
for extra prompting to the AI:
import { z } from "zod";
schema = z.object({
data: z.object({
labels: z.array(z.string()).describe("Use single words or short phrases."),
values: z.array(z.number()).describe("Use whole numbers."),
}),
type: z
.enum(["bar", "line", "pie"])
.describe(
"Use a chart type that is appropriate for the data. Only use pie charts when less than 5 values.",
),
});
Connect your data sources without writing complex integration code:
import { TamboProvider } from "@tambo-ai/react";
import { WeatherCard } from "./components/WeatherCard";
import { z } from "zod";
// Define a tool with Zod schema
const dataTool = {
name: "fetchData",
description: "Fetch data for visualization",
tool: async ({ dataType }) => {
/* fetch data */
},
toolSchema: z
.function()
.args(z.object({ dataType: z.string() }))
.returns(z.any()),
};
// Define your components with associated tools
const components = [
{
name: "WeatherCard",
description: "A component that displays weather information",
component: WeatherCard,
propsSchema: z.object({
temperature: z.number(),
condition: z.string(),
location: z.string(),
}),
associatedTools: [dataTool], // Associate the tool with the component
},
];
// Pass them to the provider
function App() {
return (
<TamboProvider apiKey="your-api-key" components={components}>
<YourApp />
</TamboProvider>
);
}
MIT License - see the LICENSE file for details.
We're building tools for the future of user interfaces. Your contributions matter.
Star this repo to support our work.
Join our Discord to connect with other developers.
FAQs
React client package for Tambo AI
The npm package @tambo-ai/react receives a total of 736 weekly downloads. As such, @tambo-ai/react popularity was classified as not popular.
We found that @tambo-ai/react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.