
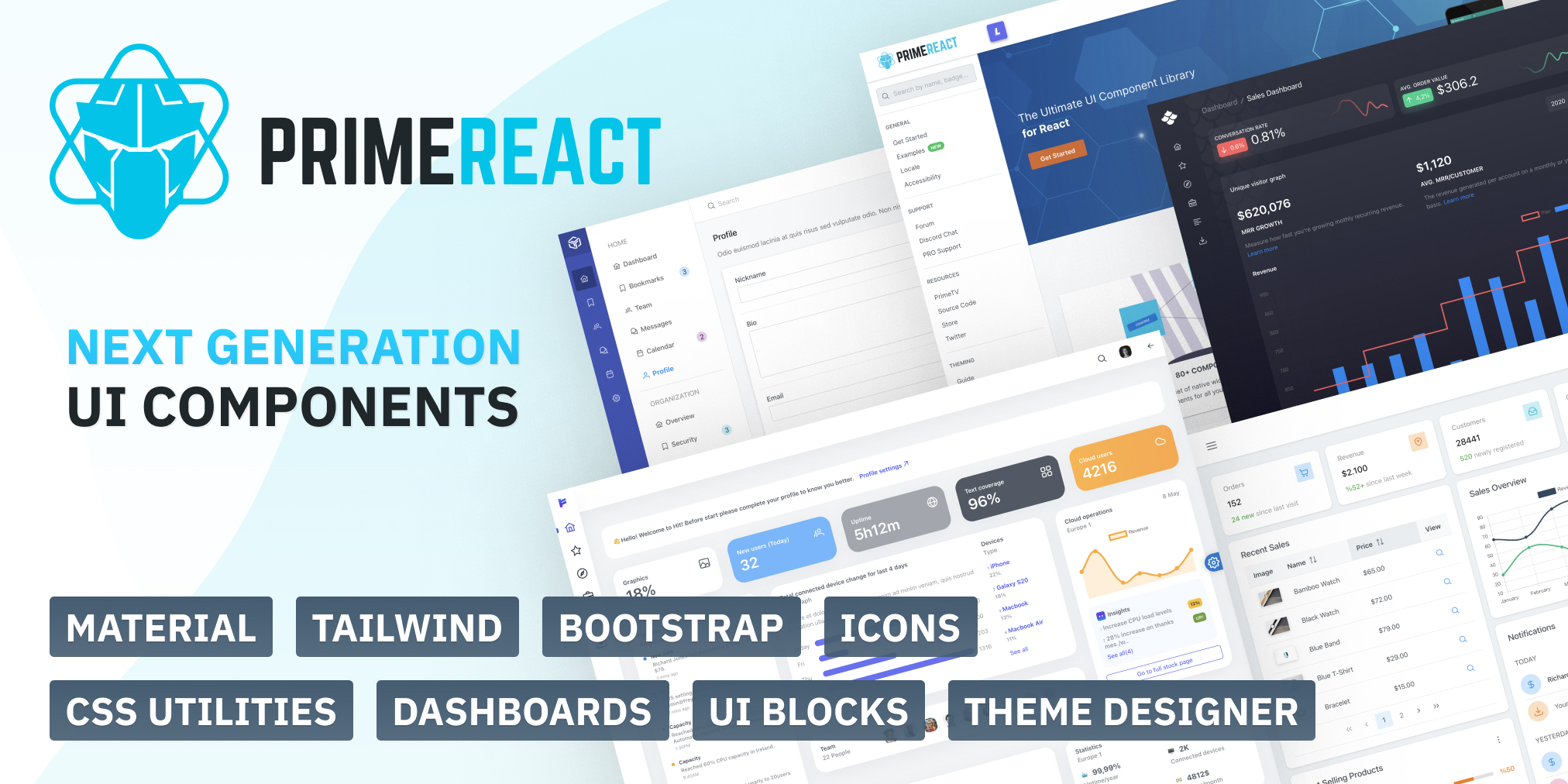
PrimeReact
PrimeReact is a rich set of open source UI Components for React. See PrimeReact homepage for live showcase and documentation.
Download
PrimeReact is available at npm, if you have an existing application run the following command to download it to your project.
// with npm
npm install primereact primeicons
// with yarn
yarn add primereact primeicons
Please note that react >= 17.0.0 and react-dom >= 17.0.0 are peer dependencies and some components have optional dependencies.
Import
Module
import { Dialog } from 'primereact/dialog';
import { Accordion, AccordionTab } from 'primereact/accordion';
CDN
<script src="https://unpkg.com/primereact/core/core.min.js"></script>
<script src="https://unpkg.com/primereact/accordion/accordion.min.js"></script>
const { Dialog } = primereact.dialog;
const { Accordion, AccordionTab } = primereact.accordion;
Import all components and structures
<script src="https://unpkg.com/primereact/primereact.all.min.js"></script>
Dependencies
Majority of PrimeReact components (95%) are native and there are some exceptions having 3rd party dependencies such as Google Maps for GMap.
In addition, components require PrimeIcons for icons and react-transition-group for animations. The react-transition-group is available as dependencies in the npm package of PrimeReact.
dependencies: {
"react": "^17.0.0 || ^18.0.0",
"react-dom": "^17.0.0 || ^18.0.0",
"primeicons": "^5.0.0 || ^6.0.0"
}
Optional
Here is the list of components with 3rd party dependencies.
Charts | Charts.js 3.x |
GMap | Google Maps |
Editor | Quill.js |
DataView | PrimeFlex |
Styles
The css dependencies are as follows, note that you may change the theme with another one of your choice.
primereact/resources/themes/lara-light-indigo/theme.css
primereact/resources/primereact.min.css
primeicons/primeicons.css
If you are using a bundler such as webpack with a css loader you may also import them to your main application component, an example from create-react-app would be.
import 'primereact/resources/themes/lara-light-indigo/theme.css';
import 'primereact/resources/primereact.min.css';
import 'primeicons/primeicons.css';
QuickStart
Example applications based on create-react-app and Next.js are available at github.
TypeScript
Typescript is fully supported as type definition files are provided in the npm package of PrimeReact. A sample typescript-primereact application is available as well at github.
Contributors
8.7.3 (2022-12-05)
Full Changelog
Implemented New Features and Enhancements:
- MultiSelect vs Dropdown has different focus behaviour #3721
- Datatable: Get the values from the table when the filter is done #3720
- Carousel: Marquee animation #3710
- Datatable: onRowMouseEnter callback #3703
- DataTable: createResponsiveStyle not called on scrollable property change #3694
- TreeSelect: allow focus in React Hook Form #3685
- BlockUI: Add
style
and className
to container #3683
- Image: imageStyle prop is of type string instead of React.CSSProperties #3668
- Datatable FilterDisplay as menu: Apply with 'Enter' #3655
- ColorPicker: Allow to set the panel's className #3654
- Listbox: Add emptyMessage like in Dropdown #3649
- Avatar: Fallback to label or icon variant when image loading fails #3647
- DataTable: RowGrouping Header Template allow control of colspan #3643
- TreeNode: Add
id
property #3616
- MultiSelect: not selecting correct value when "options" objects contain property "value" #3392
- MultiSelect: Add "overlayVisible" property #3302
- InputNumber just perform the onChange when blur #3003
- Tooltip: incorrectly positioned #2796
- MultiSelect: Enable more display options #2745
- Showcase: Save theme setting in localStorage #2671
- Tooltip: show at mouse position without following the mouse #2588
- Multiple groupField issue #2333
- ContextMenu: Submenu overflowing the page #2318
- DataTable onRowBlur and onRowMouseLeave events #1945
- DataTable grouping rows with similar names, more than one field at a time #1039
- Multi Row Grouping for DataTable #728
Fixed bugs:
- Slider with range and max=10 - initial right slider position is 1000 #3738
- Chart initialized in unmounted component #3725
- Messages/Toast: The messages.current.show([]) method causes the browser to crash #3716
- Core: PrimeReact.nullSortOrder not working correctly #3712
- Button: Tooltip remains displayed after button is disabled #3692
- TreeTable: cellClose throwing error #3689
- Tooltip: Vertical scrollbar appears when tooltip shows the first time #3687
- Dropdown: Expose focusInputRef for React Hook Form #3662
- AutoComplete: Reusing references for suggestions prop bug #3659
- Tristate and Multicheckbox: Unable to change disabled checkbox cursor #3641
- RadioButton: onChange event is called for each click #3636
- nmp run dev fails under Linux #3631
- DataTable: TypeError when adding and starting editing a new row #3476
- Dialog/Overlay: Flickering in Vite #3122
- InputNumber: Value typed by the user is not entered, when suffix used #3029
- TieredMenu: sub-items are not visible when there's not enough space at the bottom #2837
- InputNumber: input content can desync from value prop after blur #2527
- DataTable: ContextMenu showcase/theme doesn't highlight selection #2526
- OrderList: Drag & drop is not working #1883
- InputNumber: Arrow keys not working properly #1866
- DataTable: onValueChange not called when setting filter state programmatically #1396
- Cell editor with sort-filter fails #1257
- Cell Editor reapplies focus to invalid field #1247