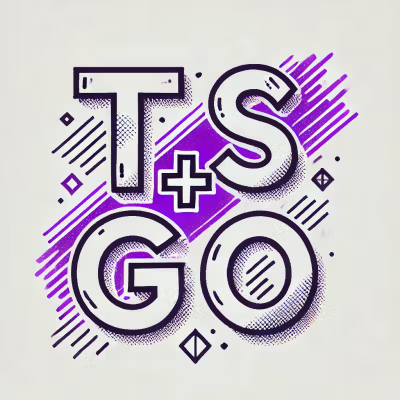
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
@tenoxui/static
Advanced tools
@tenoxui/static
This package contains CSS rule generator if you don't want to use inline-style
approach. Recommended for build.
npm i @tenoxui/static
import { TenoxUI } from '@tenoxui/static'
// usage
// new TenoxUI(config)
// example
const tenoxui = new TenoxUI({
property: {
bg: 'background'
}
})
// process the class names and include them to be processed later
tenoxui.processClassNames(['bg-red', 'bg-green'])
const stylesheet = tenoxui.generateStylesheet()
console.log(stylesheet)
/* Output :
.bg-red { background: red }
.bg-yellow { background: yellow }
*/
new TenoxUI({
property: {},
values: {},
classes: {},
aliases: {},
breakpoints: [],
reserveClass: [],
apply: {}
})
You can read documentation for property
, values
, classes
, aliases
, and breakpoints
on the main README.md
reserveClass
Reserve any class names to include on the output :
export default {
property: {
bg: 'background'
},
reserveClass: ['bg-red', 'bg-yellow']
}
Output :
.bg-red {
background: red;
}
.bg-yellow {
background: yellow;
}
apply
apply
is where you can add custom css selector, or even stacking them like regular CSS. Same as reserveClass
field, all of the rules inside apply
will included in the output. Example :
export default {
property: {
bg: 'background',
text: 'color'
},
apply: {
':root': '[--black]-#000 [--white]-#fff',
body: 'bg-$white text-$black',
'@media (prefers-color-scheme: dark)': {
':root': '[--white]-#000 [--black]-#fff'
}
}
}
Output :
:root {
--black: #000;
--white: #fff;
}
body {
background: var(--white);
color: var(--black);
}
@media (prefers-color-scheme: dark) {
:root {
--white: #000;
--black: #fff;
}
}
class TenoxUI {
private property: Property
private values: Values
private classes: Classes
private aliases: Aliases
private breakpoints: Breakpoint[]
private reserveClass: string[]
private styleMap: Map<string, Set<string>>
private apply: Record<string, StyleValue>
constructor({
property = {},
values = {},
classes = {},
aliases = {},
breakpoints = [],
reserveClass = [],
apply = {}
}: TenoxUIParams = {}) {
this.property = property
this.values = values
this.classes = classes
this.aliases = aliases
this.breakpoints = breakpoints
this.reserveClass = reserveClass
this.styleMap = new Map()
this.apply = apply
// ...
}
}
First, let's initialize tenoxui
first :
import { TenoxUI } from '@tenoxui/static'
const tenoxui = new TenoxUI({
property: {
bg: 'background'
}
/* other config here */
})
parseClassName
Main TenoxUI class name parser.
function parseClassName(
className: string
):
| [
prefix: string | undefined,
type: string,
value: string | undefined,
unit: string | undefined,
secValue: string | undefined,
secUnit: string | undefined
]
| null {}
tenoxui.parseClassName('bg-red') // [ undefined, 'bg', 'red', '', undefined, undefined ]
tenoxui.parseClassName('bg-[rgb(255_0_0)]') // [ undefined, 'bg', '[rgb(255_0_0)]', '', undefined, undefined ]
tenoxui.parseClassName('[background]-[rgb(255_0_0)]') // [ undefined, '[background]', '[rgb(255_0_0)]', '', undefined, undefined ]
tenoxui.parseClassName('p-10px') // [ undefined, 'p', '10', 'px', undefined, undefined ]
tenoxui.parseClassName('hover:m-10px/1rem') // [ 'hover', 'm', '10', 'px', '1', 'rem' ]
processShorthand
Process regular shorthands and direct CSS properties or variables.
type ProcessedStyle = {
className: string
cssRules: string | string[]
value: string | null
prefix?: string
}
processShorthand(
type: string,
value: string,
unit: string = '',
prefix?: string,
secondValue?: string,
secondUnit?: string
): ProcessedStyle | null;
Regular shorthand
tenoxui.processShorthand('bg', 'red')
Output :
{
className: 'bg-red',
cssRules: 'background',
value: 'red',
prefix: undefined
}
Direct properties
tenoxui.processShorthand('[--red,background,border-color]', 'red', '', 'hover')
Output :
{
className: '[--red,background,border-color]-red',
cssRules: '--red: red; background: red; border-color: red',
value: null,
prefix: 'hover'
}
processCustomClass
Process class names defined under config.classes
.
type ProcessedStyle = {
className: string
cssRules: string | string[]
value: string | null
prefix?: string
}
processCustomClass(className: string, prefix?: string): ProcessedStyle | null;
Let's initialize some classes :
const tenoxui = new TenoxUI({
classes: {
display: {
flex: 'flex',
'flex-center': 'flex'
},
justifyContent: {
'flex-center': 'center'
}
}
})
Regular shorthand
tenoxui.processCustomClass('flex')
Output :
{
className: 'flex-center',
cssRules: 'display: flex; justify-content: center',
value: null,
prefix: undefined
}
Direct properties
tenoxui.processCustomClass('flex-center', 'hover')
Output :
{
className: 'flex-center',
cssRules: 'display: flex; justify-content: center',
value: null,
prefix: 'hover'
}
processAlias
Process class names defined under config.aliases
.
type ProcessedStyle = {
className: string
cssRules: string | string[]
value: string | null
prefix?: string
}
processAlias(className: string, prefix?: string): ProcessedStyle | null;
Let's initialize some classes :
const tenoxui = new TenoxUI({
property: {
bg: 'background',
text: 'color',
p: 'padding'
},
classes: {
borderRadius: {
'rounded-md': '6px'
}
},
aliases: {
btn: 'bg-red text-blue p-10px rounded-md'
}
})
Example :
tenoxui.processCustomClass('btn')
Output :
{
className: 'btn',
cssRules: 'background: red; color: blue; padding: 10px; border-radius: 6px',
value: null,
prefix: ''
}
processClassNames
Include the class names into the global styleMap
and ensure them to be processed later.
processClassNames(classNames: string[]): void;
tenoxui.processClassNames(['bg-red', 'bg-yellow'])
getStyle
Return current styleMap
getStyle(): Map<string, Set<string>>;
tenoxui.processClassNames(['bg-red'])
console.log(tenoxui.getStyle()) // Map(1) { 'bg-red' => Set(1) { 'background: red' } }
addStyle
Add custom CSS rule to the styleMap
.
addStyle(className: string, cssRules: string | string[], value?: string | null, prefix?: string | null, isCustomSelector?: boolean | null): void;
Breakdown :
className
By default, this is what class name for the rule. However, you can include other selector as you want, but you have to set isCustomSelector
(last parameter) to true
. Usage example :
tenoxui.addStyle('bg-red') // => .bg-red
tenoxui.addStyle('.bg-red') // => ..bg-red (double '.')
tenoxui.addStyle('.bg-red', '', '', '', true) // .bg-red ()
cssRules
& value
These parameters is depends on each other, to ensure the functionality. Inside cssRules
, you can define direct CSS rule such as :
background: red; color: blue; padding: 10px;
But, you have to set the value
parameter to null
. However, you can set the cssRules
into array of CSS properties or variables, it allows you to set same value for multiple properties defined inside cssRules
parameter. For example :
tenoxui.addStyle('...', 'background: red; color: blue', null) // => background: red; color: blue
tenoxui.addStyle('...', ['background', 'color', '--my-color'], 'blue') // => background: blue; color: blue; --my-color: blue
prefix
(default: null
)
This is what prefix you will add to the className
, but you have to make sure the isCustomSelector
is false
.
isCustomSelector
(default: false
)
By default, the className
is treated as class selector, but you can set this to true
to prevent it treated as class selector.
tenoxui.addStyle('my-selector', 'background: red; color: blue', null, null, false)
tenoxui.addStyle('.my-selector:hover', ['--color', 'background'], 'red', null, true)
console.log(ui.getStyle())
// Output
Map(2) {
'.my-selector' => Set(1) { 'background: red; color: blue' },
'.my-selector:hover' => Set(1) { '--color: red; background: red' }
}
generateStylesheet
Generate CSS style rules that stored inside styleMap
tenoxui.processClassNames(['bg-red', 'bg-yellow'])
console.log(tenoxui.generateStylesheet())
/* Output :
.bg-red { background: red }
.bg-yellow { background: yellow }
*/
FAQs
TenoxUI static-css engine for generating stylesheet
The npm package @tenoxui/static receives a total of 391 weekly downloads. As such, @tenoxui/static popularity was classified as not popular.
We found that @tenoxui/static demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.