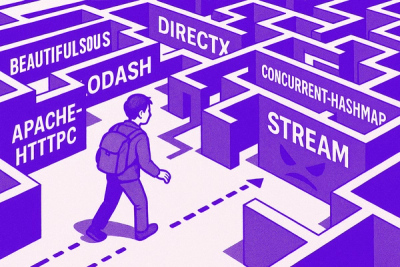
Research
NPM targeted by malware campaign mimicking familiar library names
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
@terencesun/githubdb
Advanced tools
A lightweight GitHub database operator, let your GitHub repo like mongodb
npm install @terencesun/githubdb
import { GithubDb } from "@terence/githubdb";
async function main() {
const githubdb = new GithubDb({
token: "<your GitHub token, generat from https://github.com/settings/tokens>",
owner: "<your username, like db server name>",
repo: "<your repository to store the db's data, like database name>", // Suggest that repo be private
path: "<your file path in the repository to store the db's data, like table name>",
branch: "<your repository's branch you can choose, optional, default is your default branch>"
});
// NOTE: the programe will create the branch you set if you branch is not created, and the base sha is the latest default branch commit
// if you don't want the behavior, you should create the branch by yourself
// you can use usageInfo() to get the current usage information of the API
// more detail infomation about rate limit, visit: https://docs.github.com/en/rest/using-the-rest-api/rate-limits-for-the-rest-api?apiVersion=2022-11-28
// we only focus on the primary rate limit, but not the secondary rate limit
// and you should control the rate when you use this package
const usage = await githubdb.usageInfo();
console.log(usage);
// connect to db, if the repo or path is not exist, here will create them
await githubdb.connect();
// insert operations, insertOne/insertMany
await githubdb.insertOne({ test: 1 });
await githubdb.insertMany([ { test: 1, test2: 2 }, { test3: 3 } ]);
// delete operations, deleteOne/deleteMany
await githubdb.deleteOne({ test: 1 });
await githubdb.deleteMany({ test: 1 });
// update operations, updateOne/updateMany, here is not the options like mongodb's update operation
await githubdb.updateOne({ test: 1 }, { $set: { test2: 123 } });
await githubdb.updateMany({ test: 1 }, { $set: { test2: 123 } });
// find operations, find, only one method here
await githubdb.find({ test: 1 });
/*
* Support Operators
* Query Operators: $eq/$gt/$gte/$lt/$lte/$ne/$in/$nin/$exists/$and/$or/$not/$geoWithin/$geoIntersects/$near
* Update Operators: $inc/$mul/$rename/$set/$unset/$min/$max/$currentDate/$pop/$pullAll/$pull/$push
* - Array Update Operator Modifiers: $each/$slice/$position
* - Array Update Comparison Operators: $eq/$gt/$gte/$lt/$lte/$ne/$in/$nin
* Powered by https://github.com/jclo/picodb
* thx so much
* */
// All the methods and operators is above
}
main();
We all use GitHub as a database, so, Low performance, but can be used to record some small things
MIT
FAQs
operate with github like mongodb for nodejs
The npm package @terencesun/githubdb receives a total of 0 weekly downloads. As such, @terencesun/githubdb popularity was classified as not popular.
We found that @terencesun/githubdb demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
Research
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.